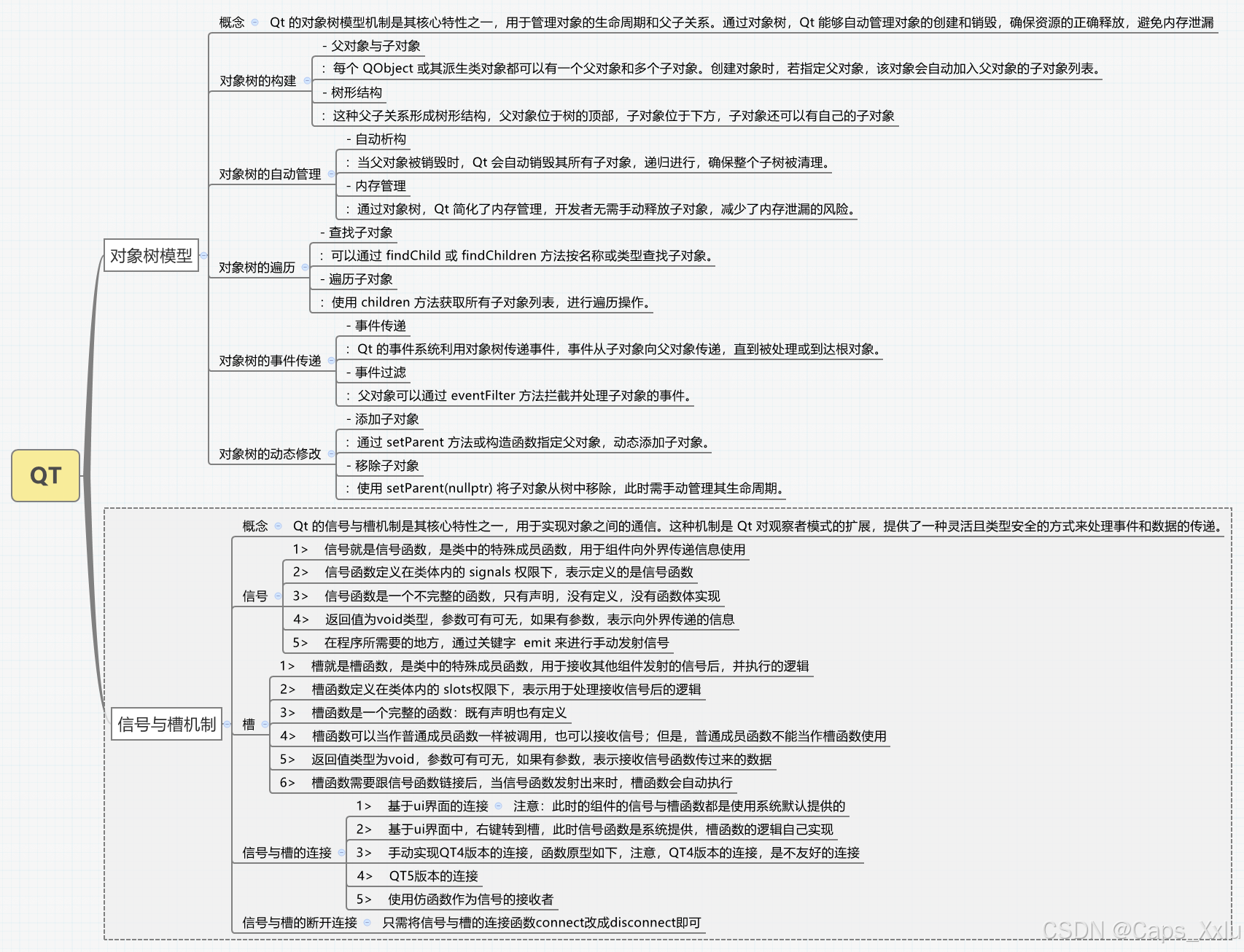
#include "housework.h"
#include "ui_housework.h"
#include<QPushButton>
#include <QPixmap>
#include<QBitmap>
#include<QPainter>
void HouseWork::handleLogin()
{
QString acc = account->text();
QString pass = password->text();
//非空判断
if(acc.isEmpty() || pass.isEmpty()){
QMessageBox::warning(this, "警告", "账号或密码不能为空!");
return;
}
// 逻辑判断、账号密码相同即为有效
if(acc == pass){
QMessageBox::information(this, "成功", "登录成功!");
emit jumpToMainWindow();
this->close();
}else{
QMessageBox::critical(this, "错误", "账号或密码错误!");
password->clear();
password->setFocus();
}
}
void HouseWork::handleCancel()
{
this->close();
}
HouseWork::HouseWork(QWidget *parent)
: QWidget(parent)
, ui(new Ui::HouseWork)
{
ui->setupUi(this);
//setupUI();
setWindowTitle("登录界面");
setFixedSize(600, 800);
this->setStyleSheet("background-color:skyblue;");
QLabel *lab1 = new QLabel(this);
lab1->resize(200,200);
lab1->move(200,150);
lab1->setPixmap(QPixmap("C:\\Users\\23866\\OneDrive\\Desktop\\头像.jpg").scaled(200,200,Qt::KeepAspectRatio,Qt::SmoothTransformation));
QBitmap mask(200, 200);
mask.fill(Qt::color0); // 初始化为透明
QPainter painter(&mask);
painter.setBrush(Qt::color1);
painter.drawEllipse(0, 0, 200, 200); // 绘制圆形
lab1->setMask(mask); // 应用遮罩
//账号
this->accountLabel = new QLabel("账号:",this);
//设置字体样式
accountLabel->setFont(QFont("楷体", 13, 10));
accountLabel->move(140,430);
//密码
this->passwordLabel = new QLabel("密码:",this);
//设置字体样式
passwordLabel->setFont(QFont("楷体", 13, 10));
passwordLabel->move(140,510);
//账号输入框
account = new QLineEdit(this);
account->resize(220,40);
account->move(200,420);
account->setStyleSheet("background-color:white;");
//密码输入框
password = new QLineEdit(this);
password->resize(220,40);
password->move(200,500);
password->setEchoMode(QLineEdit::Password);
password->setStyleSheet("background-color:white;");
//定义登录按钮
this->loginBtn = new QPushButton("登录",this);
loginBtn->resize(120,60);
loginBtn->move(150,600);
//设置按钮样式
loginBtn->setStyleSheet("background-color:white;border-radius:10px");
//定义取消按钮
this->cancelBtn = new QPushButton("取消",this);
cancelBtn->resize(this->loginBtn->size());
cancelBtn->move(this->loginBtn->x()+200,this->loginBtn->y());
//设置按钮样式
cancelBtn->setStyleSheet("background-color:white;border-radius:10px");
// 连接信号槽
connect(loginBtn, &QPushButton::clicked, this, &HouseWork::handleLogin);
connect(cancelBtn, &QPushButton::clicked, this, &HouseWork::handleCancel);
}
HouseWork::~HouseWork()
{
delete ui;
}
#ifndef HOUSEWORK_H
#define HOUSEWORK_H
#include <QWidget>
#include <QLineEdit>
#include <QPushButton>
#include <QLabel>
#include <QMessageBox>
#include <QVBoxLayout>
#include <QHBoxLayout>
QT_BEGIN_NAMESPACE
namespace Ui { class HouseWork; }
QT_END_NAMESPACE
class HouseWork : public QWidget
{
Q_OBJECT
public:
signals:
void jumpToMainWindow(); // 跳转主界面信号
private slots:
void handleLogin(); // 处理登录逻辑
void handleCancel(); // 处理取消操作
public:
HouseWork(QWidget *parent = nullptr);
~HouseWork();
private:
Ui::HouseWork *ui;
QLineEdit *account;
QLineEdit *password;
QLabel *accountLabel;
QLabel *passwordLabel;
QPushButton *cancelBtn;
QPushButton *loginBtn;
//void setupUI(); // 初始化界面
};
#endif // HOUSEWORK_H
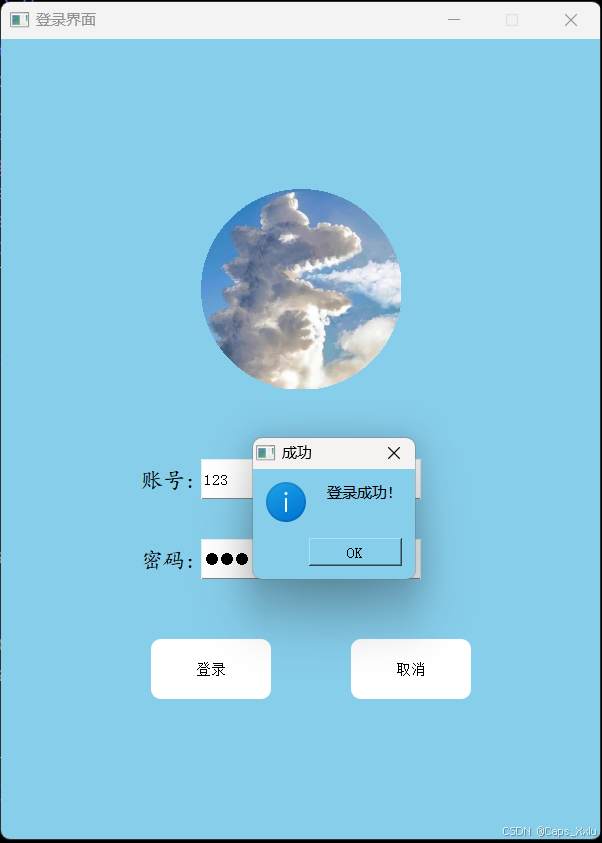
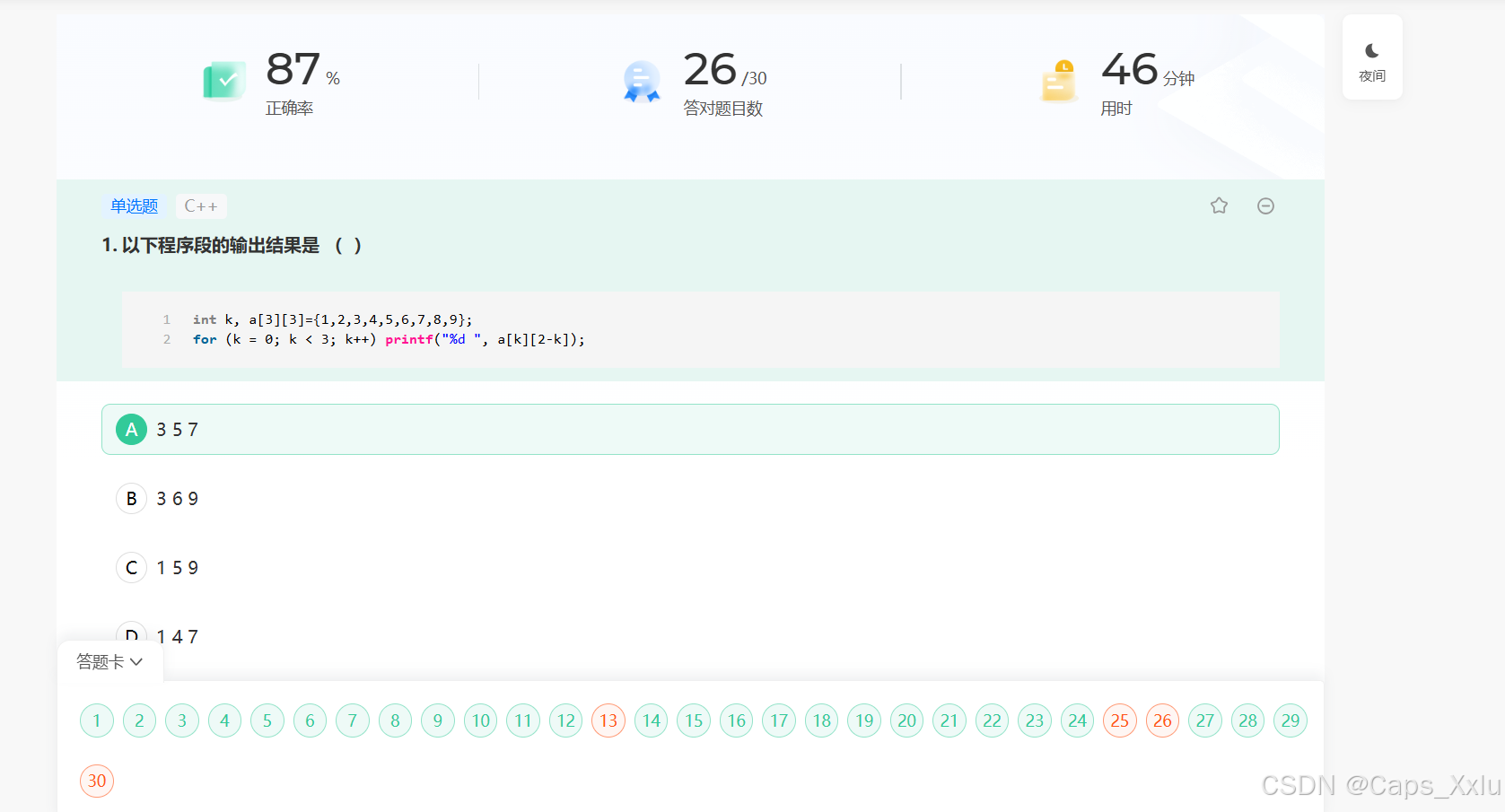