目录
STM32 F103图解
01.GPIO输出—使用固件库点亮LED
GPIO_LED_B
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LED_GPIO_Config();
while(1)
{
//GPIO_SetBits(LED_B_GPIO_PORT, LED_B_GPIO_PIN);
LED_B(OFF);
Delay(0xFFFFF);
//GPIO_ResetBits(LED_B_GPIO_PORT, LED_B_GPIO_PIN);
LED_B(ON);
Delay(0xFFFFF);
}
}
bsp_led.c
#include "bsp_led.h"
void LED_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(LED_B_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_B_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_B_GPIO_PORT, &GPIO_InitStruct);
}
bsp _led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_B_GPIO_PIN GPIO_Pin_1
#define LED_B_GPIO_PORT GPIOB
#define LED_B_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_B(a) if(a) \
GPIO_ResetBits(LED_B_GPIO_PORT, LED_B_GPIO_PIN); \
else GPIO_SetBits(LED_B_GPIO_PORT, LED_B_GPIO_PIN);
void LED_GPIO_Config(void);
#endif /* __BSP_LED_H */
GPIO_LED_G
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LED_GPIO_Config();
while(1)
{
//GPIO_SetBits(LED_G_GPIO_PORT, LED_G_GPIO_PIN);
LED_G(OFF);
Delay(0xFFFFF);
//GPIO_ResetBits(LED_G_GPIO_PORT, LED_G_GPIO_PIN);
LED_G(ON);
Delay(0xFFFFF);
}
}
bsp_led.c
#include "bsp_led.h"
void LED_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(LED_G_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_G_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_G_GPIO_PORT, &GPIO_InitStruct);
}
bsp_led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_G_GPIO_PORT GPIOB
#define LED_G_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_G(a) if(a) \
GPIO_ResetBits(LED_G_GPIO_PORT, LED_G_GPIO_PIN); \
else GPIO_SetBits(LED_G_GPIO_PORT, LED_G_GPIO_PIN);
void LED_GPIO_Config(void);
#endif /* __BSP_LED_H */
GPIO_LED_R
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LED_GPIO_Config();
while(1)
{
//GPIO_SetBits(LED_R_GPIO_PORT, LED_R_GPIO_PIN);
LED_R(OFF);
Delay(0xFFFFF);
//GPIO_ResetBits(LED_R_GPIO_PORT, LED_R_GPIO_PIN);
LED_R(ON);
Delay(0xFFFFF);
}
}
bsp_led.c
#include "bsp_led.h"
void LED_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(LED_R_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_R_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_R_GPIO_PORT, &GPIO_InitStruct);
}
bsp_led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_R_GPIO_PIN GPIO_Pin_5
#define LED_R_GPIO_PORT GPIOB
#define LED_R_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_R(a) if(a) \
GPIO_ResetBits(LED_R_GPIO_PORT, LED_R_GPIO_PIN); \
else GPIO_SetBits(LED_R_GPIO_PORT, LED_R_GPIO_PIN);
void LED_GPIO_Config(void);
#endif /* __BSP_LED_H */
GPIO_LED_RGB
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LEDR_GPIO_Config();
LEDG_GPIO_Config();
LEDB_GPIO_Config();
while(1)
{
//GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
LED_R(ON);
Delay(0xFFFFF);
//GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
LED_R(OFF);
Delay(0xFFFFF);
//GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
LED_G(ON);
Delay(0xFFFFF);
//GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
LED_G(OFF);
Delay(0xFFFFF);
//GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
LED_B(ON);
Delay(0xFFFFF);
//GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
LED_B(OFF);
Delay(0xFFFFF);
}
}
bsp_led.c
#include "bsp_led.h"
#define LED_R_GPIO_PIN GPIO_Pin_5
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_B_GPIO_PIN GPIO_Pin_1
#define LED_RGB_GPIO_PORT GPIOB
void LEDR_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_R_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDB_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_B_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDG_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_G_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
bsp_led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_R_GPIO_PIN GPIO_Pin_5
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_B_GPIO_PIN GPIO_Pin_1
#define LED_RGB_GPIO_PORT GPIOB
#define LED_RGB_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_R(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN); \
else GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
#define LED_G(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN); \
else GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
#define LED_B(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN); \
else GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
void LED_GPIO_Config(void);
#endif /* __BSP_LED_H */
02.GPIO输入-按键检测
按一次亮,再按一次灭
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
#include "bsp_key.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LED_GPIO_Config();
KEY_GPIO_Config();
while(1)
{
if( Key_Scan(KEY1_GPIO_PORT,KEY1_GPIO_PIN) ==KEY_ON )
LED_G_TOGGLE;
}
}
bsp_led.c
#include "bsp_led.h"
void LED_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(LED_G_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_G_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_G_GPIO_PORT, &GPIO_InitStruct);
}
bsp_led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_G_GPIO_PORT GPIOB
#define LED_G_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_G(a) if(a) \
GPIO_ResetBits(LED_G_GPIO_PORT, LED_G_GPIO_PIN); \
else GPIO_SetBits(LED_G_GPIO_PORT, LED_G_GPIO_PIN);
#define LED_G_TOGGLE {LED_G_GPIO_PORT->ODR ^= LED_G_GPIO_PIN;}
void LED_GPIO_Config(void);
#endif /* __BSP_LED_H */
bsp_key.c
#include "bsp_key.h"
void KEY_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(KEY1_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = KEY1_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(KEY1_GPIO_PORT, &GPIO_InitStruct);
}
uint8_t Key_Scan(GPIO_TypeDef *GPIOx,uint16_t GPIO_Pin)
{
if( GPIO_ReadInputDataBit(GPIOx, GPIO_Pin) == KEY_ON )
{
while( GPIO_ReadInputDataBit(GPIOx, GPIO_Pin) == KEY_ON );
return KEY_ON;
}
else return KEY_OFF;
}
bsp_key.h
#ifndef __BSP_KEY_H
#define __BSP_KEY_H
#include "stm32f10x.h"
#define KEY_ON 1
#define KEY_OFF 0
#define KEY1_GPIO_PIN GPIO_Pin_0
#define KEY1_GPIO_PORT GPIOA
#define KEY1_GPIO_CLK RCC_APB2Periph_GPIOA
void KEY_GPIO_Config(void);
uint8_t Key_Scan(GPIO_TypeDef *GPIOx,uint16_t GPIO_Pin);
#endif /* __BSP_KEY_H */
按下常亮,放开时灭
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
#include "bsp_key.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LEDG_GPIO_Config();
LEDB_GPIO_Config();
LEDR_GPIO_Config();
KEY_GPIO_Config();
while(1)
{
if(Key_Scan(KEY1_GPIO_PORT,KEY1_GPIO_PIN) ==KEY_ON )
{
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
}
else
{
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
}
}
}
bsp_led.c
#include "bsp_led.h"
#define LED_R_GPIO_PIN GPIO_Pin_5
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_B_GPIO_PIN GPIO_Pin_1
#define LED_RGB_GPIO_PORT GPIOB
void LEDR_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_R_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDB_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_B_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDG_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_G_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
bsp_led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_R_GPIO_PIN GPIO_Pin_5
#define LED_B_GPIO_PIN GPIO_Pin_1
#define LED_RGB_GPIO_PORT GPIOB
#define LED_RGB_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_R(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN); \
else GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
#define LED_G(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN); \
else GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
#define LED_B(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN); \
else GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
void LEDG_GPIO_Config(void);
void LEDR_GPIO_Config(void);
void LEDB_GPIO_Config(void);
#endif /* __BSP_LED_H */
bsp_key.c
#include "bsp_key.h"
void KEY_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(KEY1_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = KEY1_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(KEY1_GPIO_PORT, &GPIO_InitStruct);
}
uint8_t Key_Scan(GPIO_TypeDef *GPIOx,uint16_t GPIO_Pin)
{
if( GPIO_ReadInputDataBit(GPIOx, GPIO_Pin) == KEY_ON )
{
return KEY_ON;
}
else return KEY_OFF;
}
bsp_key.h
#ifndef __BSP_KEY_H
#define __BSP_KEY_H
#include "stm32f10x.h"
#define KEY_ON 1
#define KEY_OFF 0
#define KEY1_GPIO_PIN GPIO_Pin_0
#define KEY1_GPIO_PORT GPIOA
#define KEY1_GPIO_CLK RCC_APB2Periph_GPIOA
void KEY_GPIO_Config(void);
uint8_t Key_Scan(GPIO_TypeDef *GPIOx,uint16_t GPIO_Pin);
#endif /* __BSP_KEY_H */
03EXTI—外部中断事件控制器
按下RGB全亮,再按下RGB轮流闪烁(有瑕)
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
#include "bsp_exti.h"
void Delay( uint32_t count )
{
for(; count!=0; count--);
}
int main(void)
{
LEDR_GPIO_Config();
LEDG_GPIO_Config();
LEDB_GPIO_Config();
EXIT_Key_Config();
LEDRGB_GPIO_Config();
while(1)
{
}
}
bsp_led.c
#include "bsp_led.h"
void LEDR_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_R_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDB_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_B_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDG_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStruct.GPIO_Pin = LED_G_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_RGB_GPIO_PORT, &GPIO_InitStruct);
}
void LEDRGB_GPIO_Config(void)
{
while(1)
{
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
Delay(0xFFFFF);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
Delay(0xFFFFF);
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
Delay(0xFFFFF);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
Delay(0xFFFFF);
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
Delay(0xFFFFF);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
Delay(0xFFFFF);
}
}
bsp_led.h
#ifndef __BSP_LED_H
#define __BSP_LED_H
#include "stm32f10x.h"
#define LED_G_GPIO_PIN GPIO_Pin_0
#define LED_R_GPIO_PIN GPIO_Pin_5
#define LED_B_GPIO_PIN GPIO_Pin_1
#define LED_RGB_GPIO_PORT GPIOB
#define LED_RGB_GPIO_CLK RCC_APB2Periph_GPIOB
#define ON 1
#define OFF 0
#define LED_R(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN); Delay(0xFFFFF);\
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);Delay(0xFFFFF);\
else GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);
#define LED_G(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN); Delay(0xFFFFF);\
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);Delay(0xFFFFF);\
else GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);
#define LED_B(a) if(a) \
GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);Delay(0xFFFFF); \
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);Delay(0xFFFFF);\
else GPIO_ResetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);
#define LED_R_TOGGLE {LED_RGB_GPIO_PORT->ODR ^= GPIO_Pin_5;}
#define LED_G_TOGGLE {LED_RGB_GPIO_PORT->ODR ^= GPIO_Pin_0;}
#define LED_B_TOGGLE {LED_RGB_GPIO_PORT->ODR ^= GPIO_Pin_1;}
void LEDR_GPIO_Config(void);
void LEDG_GPIO_Config(void);
void LEDB_GPIO_Config(void);
void LEDRGB_GPIO_Config(void);
#endif /* __BSP_LED_H */
bsp_exit.c
#include "bsp_exti.h"
#include "bsp_led.h"
static void EXTI_NVIC_Config(void)
{
NVIC_InitTypeDef NVIC_InitStruct;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1);
NVIC_InitStruct.NVIC_IRQChannel = EXTI0_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
}
void EXIT_Key_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
EXTI_InitTypeDef EXTI_InitStruct;
// ÅäÖÃÖжÏÓÅÏȼ¶
EXTI_NVIC_Config();
// ³õʼ»¯GPIO
RCC_APB2PeriphClockCmd(KEY1_INT_GPIO_CLK, ENABLE);
GPIO_InitStruct.GPIO_Pin = KEY1_INT_GPIO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(KEY1_INT_GPIO_PORT, &GPIO_InitStruct);
// ³õʼ»¯EXTI
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE);
GPIO_EXTILineConfig(GPIO_PortSourceGPIOA, GPIO_PinSource0);
EXTI_InitStruct.EXTI_Line = EXTI_Line0;
EXTI_InitStruct.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStruct.EXTI_Trigger = EXTI_Trigger_Rising;
EXTI_InitStruct.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStruct);
}
bsp_exit.h
#ifndef __BSP_EXTI_H
#define __BSP_EXTI_H
#include "stm32f10x.h"
#define KEY1_INT_GPIO_PIN GPIO_Pin_0
#define KEY1_INT_GPIO_PORT GPIOA
#define KEY1_INT_GPIO_CLK RCC_APB2Periph_GPIOA
void EXIT_Key_Config(void);
#endif /* __BSP_EXTI_H */
stm32f10x_it.c
/**
******************************************************************************
* @file Project/STM32F10x_StdPeriph_Template/stm32f10x_it.c
* @author MCD Application Team
* @version V3.5.0
* @date 08-April-2011
* @brief Main Interrupt Service Routines.
* This file provides template for all exceptions handler and
* peripherals interrupt service routine.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x_it.h"
#include "bsp_led.h"
#include "bsp_exti.h"
/** @addtogroup STM32F10x_StdPeriph_Template
* @{
*/
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
/* Private functions ---------------------------------------------------------*/
/******************************************************************************/
/* Cortex-M3 Processor Exceptions Handlers */
/******************************************************************************/
/**
* @brief This function handles NMI exception.
* @param None
* @retval None
*/
void NMI_Handler(void)
{
}
/**
* @brief This function handles Hard Fault exception.
* @param None
* @retval None
*/
void HardFault_Handler(void)
{
/* Go to infinite loop when Hard Fault exception occurs */
while (1)
{
}
}
/**
* @brief This function handles Memory Manage exception.
* @param None
* @retval None
*/
void MemManage_Handler(void)
{
/* Go to infinite loop when Memory Manage exception occurs */
while (1)
{
}
}
/**
* @brief This function handles Bus Fault exception.
* @param None
* @retval None
*/
void BusFault_Handler(void)
{
/* Go to infinite loop when Bus Fault exception occurs */
while (1)
{
}
}
/**
* @brief This function handles Usage Fault exception.
* @param None
* @retval None
*/
void UsageFault_Handler(void)
{
/* Go to infinite loop when Usage Fault exception occurs */
while (1)
{
}
}
/**
* @brief This function handles SVCall exception.
* @param None
* @retval None
*/
void SVC_Handler(void)
{
}
/**
* @brief This function handles Debug Monitor exception.
* @param None
* @retval None
*/
void DebugMon_Handler(void)
{
}
/**
* @brief This function handles PendSVC exception.
* @param None
* @retval None
*/
void PendSV_Handler(void)
{
}
/**
* @brief This function handles SysTick Handler.
* @param None
* @retval None
*/
void SysTick_Handler(void)
{
}
void EXTI0_IRQHandler (void)
{
if(EXTI_GetITStatus(EXTI_Line0) != RESET)
{
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_R_GPIO_PIN);//Delay(0xFFFFF);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_G_GPIO_PIN);//Delay(0xFFFFF);
GPIO_SetBits(LED_RGB_GPIO_PORT, LED_B_GPIO_PIN);//Delay(0xFFFFF);
LED_R_TOGGLE;//Delay(0xFFFFFF);
LED_G_TOGGLE;//Delay(0xFFFFFF);
LED_B_TOGGLE;Delay(0xFFFFFF);
}
EXTI_ClearITPendingBit(EXTI_Line0);
}
/******************************************************************************/
/* STM32F10x Peripherals Interrupt Handlers */
/* Add here the Interrupt Handler for the used peripheral(s) (PPP), for the */
/* available peripheral interrupt handler's name please refer to the startup */
/* file (startup_stm32f10x_xx.s). */
/******************************************************************************/
/**
* @brief This function handles PPP interrupt request.
* @param None
* @retval None
*/
/*void PPP_IRQHandler(void)
{
}*/
/**
* @}
*/
/******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
04 ST-LINK V2仿真器
接线
ST-LINK/V2 stm32单片机
3.3V ---------------- 3.3v
Gnd ---------------- Gnd
SWCLK ---------------PA14/TCK/SWCLK
SWDIO ---------------PA13/TMS/SWDIO
RST ----------------15引脚/RST
记得拔盖插入电脑
05 编译读取中文常见问题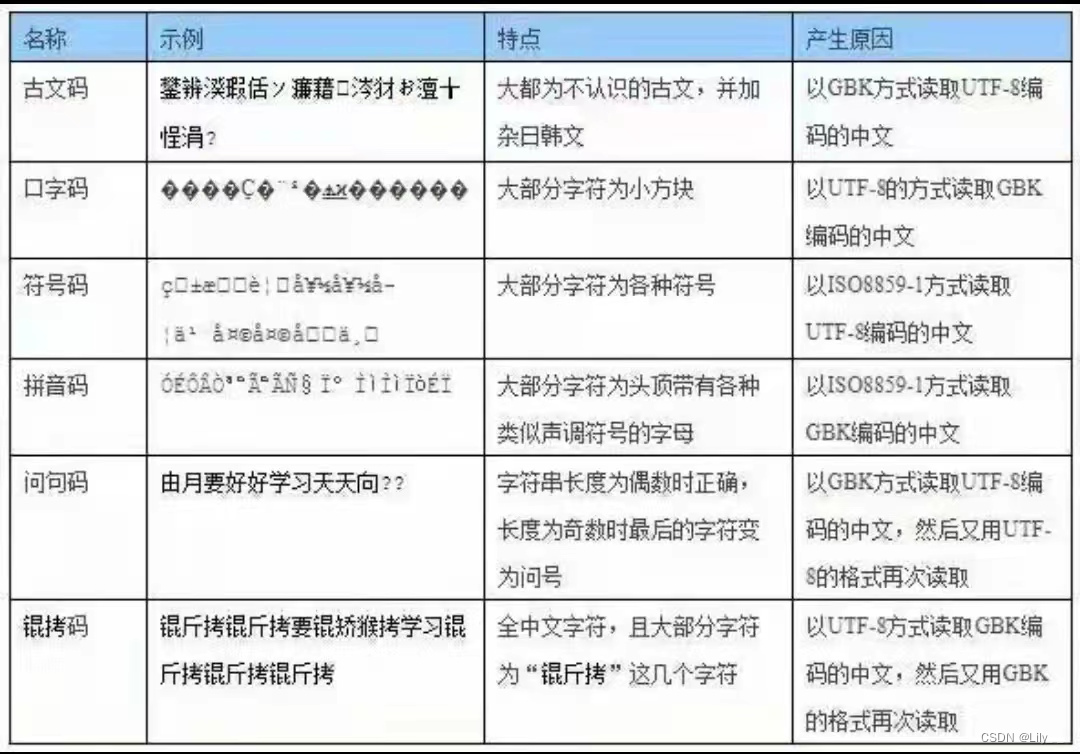
06 编译后找不到h.文件
然后选择工程文件(后缀.uvprojx)所在的目录即可。
07 JDY-31蓝牙模块手册
https://pan.baidu.com/s/1V3ZK5eqk0trze7PLqD3wgA
提取码:7185
08 蓝牙与STM32通信点亮LED
使用串口与蓝牙模块通讯点亮LED灯,发送端发送数字1点亮R灯,2点亮B灯,3点亮G灯。
注:【手机为发送端,STM32开发板为接受端】
蓝牙模块与USB转TTL的接线
VCC-----5V
GND-----GND
TXD-----RXD
RXD-----TXD
具体看其他博主有具体讲解
电脑端需下载秉火串口调试助手V1.0(其他博主有给出下载网盘)
波特率为9600
蓝牙模块与stm32接线
RXD---------PA9
TXD---------PA10
GND---------GND
VCC---------5V
!!记得把跳帽拔了
main.c
#include "stm32f10x.h"
#include "bsp_led.h"
#include "bsp_usart.h"
/**
* @brief Ö÷º¯Êý
* @param ÎÞ
* @retval ÎÞ
*/
int main(void)
{
uint8_t ch;
USART_Config();
LED_GPIO_Config();
printf( "ÕâÊÇÒ»¸ö´®¿Ú¿ØÖÆRGBµÆµÄ³ÌÐò\n" );
while (1)
{
ch = getchar();
printf( "ch=%c\n",ch );
switch(ch)
{
case '1': LED_RED;
break;
case '2': LED_BLUE;
break;
case '3': LED_GREEN;
break;
default: LED_RGBOFF;
break;
}
}
}
/*********************************************END OF FILE**********************/
bsp_usart.c
#include "bsp_usart.h"
//static void NVIC_Configuration(void)
//{
// NVIC_InitTypeDef NVIC_InitStructure;
//
// /* ǶÌ×ÏòÁ¿ÖжϿØÖÆÆ÷×éÑ¡Ôñ */
// NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
//
// /* ÅäÖÃUSARTΪÖжÏÔ´ */
// NVIC_InitStructure.NVIC_IRQChannel = DEBUG_USART_IRQ;
// /* ÇÀ¶ÏÓÅÏȼ¶*/
// NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
// /* ×ÓÓÅÏȼ¶ */
// NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
// /* ʹÄÜÖÐ¶Ï */
// NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
// /* ³õʼ»¯ÅäÖÃNVIC */
// NVIC_Init(&NVIC_InitStructure);
//}
void USART_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// ´ò¿ª´®¿ÚGPIOµÄʱÖÓ
DEBUG_USART_GPIO_APBxClkCmd(DEBUG_USART_GPIO_CLK, ENABLE);
// ´ò¿ª´®¿ÚÍâÉèµÄʱÖÓ
DEBUG_USART_APBxClkCmd(DEBUG_USART_CLK, ENABLE);
// ½«USART TxµÄGPIOÅäÖÃÎªÍÆÍ츴ÓÃģʽ
GPIO_InitStructure.GPIO_Pin = DEBUG_USART_TX_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DEBUG_USART_TX_GPIO_PORT, &GPIO_InitStructure);
// ½«USART RxµÄGPIOÅäÖÃΪ¸¡¿ÕÊäÈëģʽ
GPIO_InitStructure.GPIO_Pin = DEBUG_USART_RX_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(DEBUG_USART_RX_GPIO_PORT, &GPIO_InitStructure);
// ÅäÖô®¿ÚµÄ¹¤×÷²ÎÊý
// ÅäÖò¨ÌØÂÊ
USART_InitStructure.USART_BaudRate = DEBUG_USART_BAUDRATE;
// ÅäÖà ÕëÊý¾Ý×Ö³¤
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
// ÅäÖÃֹͣλ
USART_InitStructure.USART_StopBits = USART_StopBits_1;
// ÅäÖÃУÑéλ
USART_InitStructure.USART_Parity = USART_Parity_No ;
// ÅäÖÃÓ²¼þÁ÷¿ØÖÆ
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
// ÅäÖù¤×÷ģʽ£¬ÊÕ·¢Ò»Æð
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
// Íê³É´®¿ÚµÄ³õʼ»¯ÅäÖÃ
USART_Init(DEBUG_USARTx, &USART_InitStructure);
// ´®¿ÚÖжÏÓÅÏȼ¶ÅäÖÃ
// // NVIC_Configuration();
//
// // ʹÄÜ´®¿Ú½ÓÊÕÖжÏ
// USART_ITConfig(DEBUG_USARTx, USART_IT_RXNE, ENABLE);
// ʹÄÜ´®¿Ú
USART_Cmd(DEBUG_USARTx, ENABLE);
}
/* ·¢ËÍÒ»¸ö×Ö½Ú */
void Usart_SendByte(USART_TypeDef* pUSARTx, uint8_t data)
{
USART_SendData(pUSARTx, data);
while( USART_GetFlagStatus(pUSARTx, USART_FLAG_TXE) == RESET );
}
/* ·¢ËÍÁ½¸ö×Ö½ÚµÄÊý¾Ý */
void Usart_SendHalfWord(USART_TypeDef* pUSARTx, uint16_t data)
{
uint8_t temp_h,temp_l;
temp_h = (data&0xff00) >> 8 ;
temp_l = data&0xff;
USART_SendData(pUSARTx, temp_h);
while( USART_GetFlagStatus(pUSARTx, USART_FLAG_TXE) == RESET );
USART_SendData(pUSARTx, temp_l);
while( USART_GetFlagStatus(pUSARTx, USART_FLAG_TXE) == RESET );
}
/* ·¢ËÍ8λÊý¾ÝµÄÊý×é */
void Usart_SendArray(USART_TypeDef* pUSARTx, uint8_t *array,uint8_t num)
{
uint8_t i;
for( i=0; i<num; i++ )
{
Usart_SendByte(pUSARTx, array[i]);
}
while( USART_GetFlagStatus(pUSARTx, USART_FLAG_TC) == RESET );
}
/* ·¢ËÍ×Ö·û´® */
void Usart_SendStr(USART_TypeDef* pUSARTx, uint8_t *str)
{
uint8_t i=0;
do
{
Usart_SendByte(pUSARTx, *(str+i));
i++;
}while(*(str+i) != '\0');
while( USART_GetFlagStatus(pUSARTx, USART_FLAG_TC) == RESET );
}
///ÖØ¶¨Ïòc¿âº¯Êýprintfµ½´®¿Ú£¬Öض¨Ïòºó¿ÉʹÓÃprintfº¯Êý
int fputc(int ch, FILE *f)
{
/* ·¢ËÍÒ»¸ö×Ö½ÚÊý¾Ýµ½´®¿Ú */
USART_SendData(DEBUG_USARTx, (uint8_t) ch);
/* µÈ´ý·¢ËÍÍê±Ï */
while (USART_GetFlagStatus(DEBUG_USARTx, USART_FLAG_TXE) == RESET);
return (ch);
}
///ÖØ¶¨Ïòc¿âº¯Êýscanfµ½´®¿Ú£¬ÖØÐ´Ïòºó¿ÉʹÓÃscanf¡¢getcharµÈº¯Êý
int fgetc(FILE *f)
{
/* µÈ´ý´®¿ÚÊäÈëÊý¾Ý */
while (USART_GetFlagStatus(DEBUG_USARTx, USART_FLAG_RXNE) == RESET);
return (int)USART_ReceiveData(DEBUG_USARTx);
}
bsp_usart.h
#ifndef __BSP_USART_H
#define __BSP_USART_H
#include "stm32f10x.h"
#include <stdio.h>
#define DEBUG_USART1 1
#define DEBUG_USART2 0
#define DEBUG_USART3 0
#define DEBUG_USART4 0
#define DEBUG_USART5 0
#if DEBUG_USART1
// ´®¿Ú1-USART1
#define DEBUG_USARTx USART1
#define DEBUG_USART_CLK RCC_APB2Periph_USART1
#define DEBUG_USART_APBxClkCmd RCC_APB2PeriphClockCmd
#define DEBUG_USART_BAUDRATE 9600
// USART GPIO Òý½Åºê¶¨Òå
#define DEBUG_USART_GPIO_CLK (RCC_APB2Periph_GPIOA)
#define DEBUG_USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
#define DEBUG_USART_TX_GPIO_PORT GPIOA
#define DEBUG_USART_TX_GPIO_PIN GPIO_Pin_9
#define DEBUG_USART_RX_GPIO_PORT GPIOA
#define DEBUG_USART_RX_GPIO_PIN GPIO_Pin_10
#define DEBUG_USART_IRQ USART1_IRQn
#define DEBUG_USART_IRQHandler USART1_IRQHandler
#elif DEBUG_USART2
//´®¿Ú2-USART2
#define DEBUG_USARTx USART2
#define DEBUG_USART_CLK RCC_APB1Periph_USART2
#define DEBUG_USART_APBxClkCmd RCC_APB1PeriphClockCmd
#define DEBUG_USART_BAUDRATE 115200
// USART GPIO Òý½Åºê¶¨Òå
#define DEBUG_USART_GPIO_CLK (RCC_APB2Periph_GPIOA)
#define DEBUG_USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
#define DEBUG_USART_TX_GPIO_PORT GPIOA
#define DEBUG_USART_TX_GPIO_PIN GPIO_Pin_2
#define DEBUG_USART_RX_GPIO_PORT GPIOA
#define DEBUG_USART_RX_GPIO_PIN GPIO_Pin_3
#define DEBUG_USART_IRQ USART2_IRQn
#define DEBUG_USART_IRQHandler USART2_IRQHandler
#elif DEBUG_USART3
//´®¿Ú3-USART3
#define DEBUG_USARTx USART3
#define DEBUG_USART_CLK RCC_APB1Periph_USART3
#define DEBUG_USART_APBxClkCmd RCC_APB1PeriphClockCmd
#define DEBUG_USART_BAUDRATE 115200
// USART GPIO Òý½Åºê¶¨Òå
#define DEBUG_USART_GPIO_CLK (RCC_APB2Periph_GPIOB)
#define DEBUG_USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
#define DEBUG_USART_TX_GPIO_PORT GPIOB
#define DEBUG_USART_TX_GPIO_PIN GPIO_Pin_10
#define DEBUG_USART_RX_GPIO_PORT GPIOB
#define DEBUG_USART_RX_GPIO_PIN GPIO_Pin_11
#define DEBUG_USART_IRQ USART3_IRQn
#define DEBUG_USART_IRQHandler USART3_IRQHandler
#elif DEBUG_USART4
//´®¿Ú4-UART4
#define DEBUG_USARTx UART4
#define DEBUG_USART_CLK RCC_APB1Periph_UART4
#define DEBUG_USART_APBxClkCmd RCC_APB1PeriphClockCmd
#define DEBUG_USART_BAUDRATE 115200
// USART GPIO Òý½Åºê¶¨Òå
#define DEBUG_USART_GPIO_CLK (RCC_APB2Periph_GPIOC)
#define DEBUG_USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
#define DEBUG_USART_TX_GPIO_PORT GPIOC
#define DEBUG_USART_TX_GPIO_PIN GPIO_Pin_10
#define DEBUG_USART_RX_GPIO_PORT GPIOC
#define DEBUG_USART_RX_GPIO_PIN GPIO_Pin_11
#define DEBUG_USART_IRQ UART4_IRQn
#define DEBUG_USART_IRQHandler UART4_IRQHandler
#elif DEBUG_USART5
//´®¿Ú5-UART5
#define DEBUG_USARTx UART5
#define DEBUG_USART_CLK RCC_APB1Periph_UART5
#define DEBUG_USART_APBxClkCmd RCC_APB1PeriphClockCmd
#define DEBUG_USART_BAUDRATE 115200
// USART GPIO Òý½Åºê¶¨Òå
#define DEBUG_USART_GPIO_CLK (RCC_APB2Periph_GPIOC|RCC_APB2Periph_GPIOD)
#define DEBUG_USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
#define DEBUG_USART_TX_GPIO_PORT GPIOC
#define DEBUG_USART_TX_GPIO_PIN GPIO_Pin_12
#define DEBUG_USART_RX_GPIO_PORT GPIOD
#define DEBUG_USART_RX_GPIO_PIN GPIO_Pin_2
#define DEBUG_USART_IRQ UART5_IRQn
#define DEBUG_USART_IRQHandler UART5_IRQHandler
#endif
void USART_Config(void);
void Usart_SendByte(USART_TypeDef* pUSARTx, uint8_t data);
void Usart_SendHalfWord(USART_TypeDef* pUSARTx, uint16_t data);
void Usart_SendArray(USART_TypeDef* pUSARTx, uint8_t *array,uint8_t num);
void Usart_SendStr(USART_TypeDef* pUSARTx, uint8_t *str);
#endif /* __BSP_USART_H */
led.c
/**
******************************************************************************
* @file bsp_led.c
* @author fire
* @version V1.0
* @date 2013-xx-xx
* @brief ledÓ¦Óú¯Êý½Ó¿Ú
******************************************************************************
* @attention
*
* ʵÑéÆ½Ì¨:±ü»ð F103-°ÔµÀ STM32 ¿ª·¢°å
* ÂÛ̳ :http://www.firebbs.cn
* ÌÔ±¦ :http://firestm32.taobao.com
*
******************************************************************************
*/
#include "bsp_led.h"
/**
* @brief ³õʼ»¯¿ØÖÆLEDµÄIO
* @param ÎÞ
* @retval ÎÞ
*/
void LED_GPIO_Config(void)
{
/*¶¨ÒåÒ»¸öGPIO_InitTypeDefÀàÐ͵ĽṹÌå*/
GPIO_InitTypeDef GPIO_InitStructure;
/*¿ªÆôLEDÏà¹ØµÄGPIOÍâÉèʱÖÓ*/
RCC_APB2PeriphClockCmd( LED1_GPIO_CLK | LED2_GPIO_CLK | LED3_GPIO_CLK, ENABLE);
/*Ñ¡ÔñÒª¿ØÖƵÄGPIOÒý½Å*/
GPIO_InitStructure.GPIO_Pin = LED1_GPIO_PIN;
/*ÉèÖÃÒý½ÅģʽΪͨÓÃÍÆÍìÊä³ö*/
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
/*ÉèÖÃÒý½ÅËÙÂÊΪ50MHz */
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
/*µ÷Óÿ⺯Êý£¬³õʼ»¯GPIO*/
GPIO_Init(LED1_GPIO_PORT, &GPIO_InitStructure);
/*Ñ¡ÔñÒª¿ØÖƵÄGPIOÒý½Å*/
GPIO_InitStructure.GPIO_Pin = LED2_GPIO_PIN;
/*µ÷Óÿ⺯Êý£¬³õʼ»¯GPIO*/
GPIO_Init(LED2_GPIO_PORT, &GPIO_InitStructure);
/*Ñ¡ÔñÒª¿ØÖƵÄGPIOÒý½Å*/
GPIO_InitStructure.GPIO_Pin = LED3_GPIO_PIN;
/*µ÷Óÿ⺯Êý£¬³õʼ»¯GPIOF*/
GPIO_Init(LED3_GPIO_PORT, &GPIO_InitStructure);
/* ¹Ø±ÕËùÓÐledµÆ */
GPIO_SetBits(LED1_GPIO_PORT, LED1_GPIO_PIN);
/* ¹Ø±ÕËùÓÐledµÆ */
GPIO_SetBits(LED2_GPIO_PORT, LED2_GPIO_PIN);
/* ¹Ø±ÕËùÓÐledµÆ */
GPIO_SetBits(LED3_GPIO_PORT, LED3_GPIO_PIN);
}
/*********************************************END OF FILE**********************/
led.h
#ifndef __LED_H
#define __LED_H
#include "stm32f10x.h"
/* ¶¨ÒåLEDÁ¬½ÓµÄGPIO¶Ë¿Ú, Óû§Ö»ÐèÒªÐÞ¸ÄÏÂÃæµÄ´úÂë¼´¿É¸Ä±ä¿ØÖƵÄLEDÒý½Å */
// R-ºìÉ«
#define LED1_GPIO_PORT GPIOB /* GPIO¶Ë¿Ú */
#define LED1_GPIO_CLK RCC_APB2Periph_GPIOB /* GPIO¶Ë¿ÚʱÖÓ */
#define LED1_GPIO_PIN GPIO_Pin_5 /* Á¬½Óµ½SCLʱÖÓÏßµÄGPIO */
// G-ÂÌÉ«
#define LED2_GPIO_PORT GPIOB /* GPIO¶Ë¿Ú */
#define LED2_GPIO_CLK RCC_APB2Periph_GPIOB /* GPIO¶Ë¿ÚʱÖÓ */
#define LED2_GPIO_PIN GPIO_Pin_0 /* Á¬½Óµ½SCLʱÖÓÏßµÄGPIO */
// B-À¶É«
#define LED3_GPIO_PORT GPIOB /* GPIO¶Ë¿Ú */
#define LED3_GPIO_CLK RCC_APB2Periph_GPIOB /* GPIO¶Ë¿ÚʱÖÓ */
#define LED3_GPIO_PIN GPIO_Pin_1 /* Á¬½Óµ½SCLʱÖÓÏßµÄGPIO */
/** the macro definition to trigger the led on or off
* 1 - off
*0 - on
*/
#define ON 0
#define OFF 1
/* ʹÓñê×¼µÄ¹Ì¼þ¿â¿ØÖÆIO*/
#define LED1(a) if (a) \
GPIO_SetBits(LED1_GPIO_PORT,LED1_GPIO_PIN);\
else \
GPIO_ResetBits(LED1_GPIO_PORT,LED1_GPIO_PIN)
#define LED2(a) if (a) \
GPIO_SetBits(LED2_GPIO_PORT,LED2_GPIO_PIN);\
else \
GPIO_ResetBits(LED2_GPIO_PORT,LED2_GPIO_PIN)
#define LED3(a) if (a) \
GPIO_SetBits(LED3_GPIO_PORT,LED3_GPIO_PIN);\
else \
GPIO_ResetBits(LED3_GPIO_PORT,LED3_GPIO_PIN)
/* Ö±½Ó²Ù×÷¼Ä´æÆ÷µÄ·½·¨¿ØÖÆIO */
#define digitalHi(p,i) {p->BSRR=i;} //Êä³öΪ¸ßµçƽ
#define digitalLo(p,i) {p->BRR=i;} //Êä³öµÍµçƽ
#define digitalToggle(p,i) {p->ODR ^=i;} //Êä³ö·´×ª×´Ì¬
/* ¶¨Òå¿ØÖÆIOµÄºê */
#define LED1_TOGGLE digitalToggle(LED1_GPIO_PORT,LED1_GPIO_PIN)
#define LED1_OFF digitalHi(LED1_GPIO_PORT,LED1_GPIO_PIN)
#define LED1_ON digitalLo(LED1_GPIO_PORT,LED1_GPIO_PIN)
#define LED2_TOGGLE digitalToggle(LED2_GPIO_PORT,LED2_GPIO_PIN)
#define LED2_OFF digitalHi(LED2_GPIO_PORT,LED2_GPIO_PIN)
#define LED2_ON digitalLo(LED2_GPIO_PORT,LED2_GPIO_PIN)
#define LED3_TOGGLE digitalToggle(LED3_GPIO_PORT,LED3_GPIO_PIN)
#define LED3_OFF digitalHi(LED3_GPIO_PORT,LED3_GPIO_PIN)
#define LED3_ON digitalLo(LED3_GPIO_PORT,LED3_GPIO_PIN)
/* »ù±¾»ìÉ«£¬ºóÃæ¸ß¼¶Ó÷¨Ê¹ÓÃPWM¿É»ì³öÈ«²ÊÑÕÉ«,ÇÒЧ¹û¸üºÃ */
//ºì
#define LED_RED \
LED1_ON;\
LED2_OFF\
LED3_OFF
//ÂÌ
#define LED_GREEN \
LED1_OFF;\
LED2_ON\
LED3_OFF
//˦
#define LED_BLUE \
LED1_OFF;\
LED2_OFF\
LED3_ON
//»Æ(ºì+ÂÌ)
#define LED_YELLOW \
LED1_ON;\
LED2_ON\
LED3_OFF
//×Ï(ºì+À¶)
#define LED_PURPLE \
LED1_ON;\
LED2_OFF\
LED3_ON
//Çà(ÂÌ+À¶)
#define LED_CYAN \
LED1_OFF;\
LED2_ON\
LED3_ON
//°×(ºì+ÂÌ+À¶)
#define LED_WHITE \
LED1_ON;\
LED2_ON\
LED3_ON
//ºÚ(È«²¿¹Ø±Õ)
#define LED_RGBOFF \
LED1_OFF;\
LED2_OFF\
LED3_OFF
void LED_GPIO_Config(void);
#endif /* __LED_H */
stm32f10x_conf.h
/**
******************************************************************************
* @file Project/STM32F10x_StdPeriph_Template/stm32f10x_conf.h
* @author MCD Application Team
* @version V3.5.0
* @date 08-April-2011
* @brief Library configuration file.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Define to prevent recursive inclusion -------------------------------------*/
#ifndef __STM32F10x_CONF_H
#define __STM32F10x_CONF_H
/* Includes ------------------------------------------------------------------*/
/* Uncomment/Comment the line below to enable/disable peripheral header file inclusion */
#include "stm32f10x_adc.h"
#include "stm32f10x_bkp.h"
#include "stm32f10x_can.h"
#include "stm32f10x_cec.h"
#include "stm32f10x_crc.h"
#include "stm32f10x_dac.h"
#include "stm32f10x_dbgmcu.h"
#include "stm32f10x_dma.h"
#include "stm32f10x_exti.h"
#include "stm32f10x_flash.h"
#include "stm32f10x_fsmc.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_i2c.h"
#include "stm32f10x_iwdg.h"
#include "stm32f10x_pwr.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_rtc.h"
#include "stm32f10x_sdio.h"
#include "stm32f10x_spi.h"
#include "stm32f10x_tim.h"
#include "stm32f10x_usart.h"
#include "stm32f10x_wwdg.h"
#include "misc.h" /* High level functions for NVIC and SysTick (add-on to CMSIS functions) */
/* Exported types ------------------------------------------------------------*/
/* Exported constants --------------------------------------------------------*/
/* Uncomment the line below to expanse the "assert_param" macro in the
Standard Peripheral Library drivers code */
/* #define USE_FULL_ASSERT 1 */
/* Exported macro ------------------------------------------------------------*/
//#define USE_FULL_ASSERT
#ifdef USE_FULL_ASSERT
/**
* @brief The assert_param macro is used for function's parameters check.
* @param expr: If expr is false, it calls assert_failed function which reports
* the name of the source file and the source line number of the call
* that failed. If expr is true, it returns no value.
* @retval None
*/
#define assert_param(expr) ((expr) ? (void)0 : assert_failed((uint8_t *)__FILE__, __LINE__))
/* Exported functions ------------------------------------------------------- */
void assert_failed(uint8_t* file, uint32_t line);
#else
#define assert_param(expr) ((void)0)
#endif /* USE_FULL_ASSERT */
#endif /* __STM32F10x_CONF_H */
/******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
stm32f10x_usart.c
/**
******************************************************************************
* @file stm32f10x_usart.c
* @author MCD Application Team
* @version V3.5.0
* @date 11-March-2011
* @brief This file provides all the USART firmware functions.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x_usart.h"
#include "stm32f10x_rcc.h"
/** @addtogroup STM32F10x_StdPeriph_Driver
* @{
*/
/** @defgroup USART
* @brief USART driver modules
* @{
*/
/** @defgroup USART_Private_TypesDefinitions
* @{
*/
/**
* @}
*/
/** @defgroup USART_Private_Defines
* @{
*/
#define CR1_UE_Set ((uint16_t)0x2000) /*!< USART Enable Mask */
#define CR1_UE_Reset ((uint16_t)0xDFFF) /*!< USART Disable Mask */
#define CR1_WAKE_Mask ((uint16_t)0xF7FF) /*!< USART WakeUp Method Mask */
#define CR1_RWU_Set ((uint16_t)0x0002) /*!< USART mute mode Enable Mask */
#define CR1_RWU_Reset ((uint16_t)0xFFFD) /*!< USART mute mode Enable Mask */
#define CR1_SBK_Set ((uint16_t)0x0001) /*!< USART Break Character send Mask */
#define CR1_CLEAR_Mask ((uint16_t)0xE9F3) /*!< USART CR1 Mask */
#define CR2_Address_Mask ((uint16_t)0xFFF0) /*!< USART address Mask */
#define CR2_LINEN_Set ((uint16_t)0x4000) /*!< USART LIN Enable Mask */
#define CR2_LINEN_Reset ((uint16_t)0xBFFF) /*!< USART LIN Disable Mask */
#define CR2_LBDL_Mask ((uint16_t)0xFFDF) /*!< USART LIN Break detection Mask */
#define CR2_STOP_CLEAR_Mask ((uint16_t)0xCFFF) /*!< USART CR2 STOP Bits Mask */
#define CR2_CLOCK_CLEAR_Mask ((uint16_t)0xF0FF) /*!< USART CR2 Clock Mask */
#define CR3_SCEN_Set ((uint16_t)0x0020) /*!< USART SC Enable Mask */
#define CR3_SCEN_Reset ((uint16_t)0xFFDF) /*!< USART SC Disable Mask */
#define CR3_NACK_Set ((uint16_t)0x0010) /*!< USART SC NACK Enable Mask */
#define CR3_NACK_Reset ((uint16_t)0xFFEF) /*!< USART SC NACK Disable Mask */
#define CR3_HDSEL_Set ((uint16_t)0x0008) /*!< USART Half-Duplex Enable Mask */
#define CR3_HDSEL_Reset ((uint16_t)0xFFF7) /*!< USART Half-Duplex Disable Mask */
#define CR3_IRLP_Mask ((uint16_t)0xFFFB) /*!< USART IrDA LowPower mode Mask */
#define CR3_CLEAR_Mask ((uint16_t)0xFCFF) /*!< USART CR3 Mask */
#define CR3_IREN_Set ((uint16_t)0x0002) /*!< USART IrDA Enable Mask */
#define CR3_IREN_Reset ((uint16_t)0xFFFD) /*!< USART IrDA Disable Mask */
#define GTPR_LSB_Mask ((uint16_t)0x00FF) /*!< Guard Time Register LSB Mask */
#define GTPR_MSB_Mask ((uint16_t)0xFF00) /*!< Guard Time Register MSB Mask */
#define IT_Mask ((uint16_t)0x001F) /*!< USART Interrupt Mask */
/* USART OverSampling-8 Mask */
#define CR1_OVER8_Set ((u16)0x8000) /* USART OVER8 mode Enable Mask */
#define CR1_OVER8_Reset ((u16)0x7FFF) /* USART OVER8 mode Disable Mask */
/* USART One Bit Sampling Mask */
#define CR3_ONEBITE_Set ((u16)0x0800) /* USART ONEBITE mode Enable Mask */
#define CR3_ONEBITE_Reset ((u16)0xF7FF) /* USART ONEBITE mode Disable Mask */
/**
* @}
*/
/** @defgroup USART_Private_Macros
* @{
*/
/**
* @}
*/
/** @defgroup USART_Private_Variables
* @{
*/
/**
* @}
*/
/** @defgroup USART_Private_FunctionPrototypes
* @{
*/
/**
* @}
*/
/** @defgroup USART_Private_Functions
* @{
*/
/**
* @brief Deinitializes the USARTx peripheral registers to their default reset values.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @retval None
*/
void USART_DeInit(USART_TypeDef* USARTx)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
if (USARTx == USART1)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_USART1, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_USART1, DISABLE);
}
else if (USARTx == USART2)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART2, DISABLE);
}
else if (USARTx == USART3)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART3, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_USART3, DISABLE);
}
else if (USARTx == UART4)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART4, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART4, DISABLE);
}
else
{
if (USARTx == UART5)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART5, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_UART5, DISABLE);
}
}
}
/**
* @brief Initializes the USARTx peripheral according to the specified
* parameters in the USART_InitStruct .
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_InitStruct: pointer to a USART_InitTypeDef structure
* that contains the configuration information for the specified USART
* peripheral.
* @retval None
*/
void USART_Init(USART_TypeDef* USARTx, USART_InitTypeDef* USART_InitStruct)
{
uint32_t tmpreg = 0x00, apbclock = 0x00;
uint32_t integerdivider = 0x00;
uint32_t fractionaldivider = 0x00;
uint32_t usartxbase = 0;
RCC_ClocksTypeDef RCC_ClocksStatus;
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_BAUDRATE(USART_InitStruct->USART_BaudRate));
assert_param(IS_USART_WORD_LENGTH(USART_InitStruct->USART_WordLength));
assert_param(IS_USART_STOPBITS(USART_InitStruct->USART_StopBits));
assert_param(IS_USART_PARITY(USART_InitStruct->USART_Parity));
assert_param(IS_USART_MODE(USART_InitStruct->USART_Mode));
assert_param(IS_USART_HARDWARE_FLOW_CONTROL(USART_InitStruct->USART_HardwareFlowControl));
/* The hardware flow control is available only for USART1, USART2 and USART3 */
if (USART_InitStruct->USART_HardwareFlowControl != USART_HardwareFlowControl_None)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
usartxbase = (uint32_t)USARTx;
/*---------------------------- USART CR2 Configuration -----------------------*/
tmpreg = USARTx->CR2;
/* Clear STOP[13:12] bits */
tmpreg &= CR2_STOP_CLEAR_Mask;
/* Configure the USART Stop Bits, Clock, CPOL, CPHA and LastBit ------------*/
/* Set STOP[13:12] bits according to USART_StopBits value */
tmpreg |= (uint32_t)USART_InitStruct->USART_StopBits;
/* Write to USART CR2 */
USARTx->CR2 = (uint16_t)tmpreg;
/*---------------------------- USART CR1 Configuration -----------------------*/
tmpreg = USARTx->CR1;
/* Clear M, PCE, PS, TE and RE bits */
tmpreg &= CR1_CLEAR_Mask;
/* Configure the USART Word Length, Parity and mode ----------------------- */
/* Set the M bits according to USART_WordLength value */
/* Set PCE and PS bits according to USART_Parity value */
/* Set TE and RE bits according to USART_Mode value */
tmpreg |= (uint32_t)USART_InitStruct->USART_WordLength | USART_InitStruct->USART_Parity |
USART_InitStruct->USART_Mode;
/* Write to USART CR1 */
USARTx->CR1 = (uint16_t)tmpreg;
/*---------------------------- USART CR3 Configuration -----------------------*/
tmpreg = USARTx->CR3;
/* Clear CTSE and RTSE bits */
tmpreg &= CR3_CLEAR_Mask;
/* Configure the USART HFC -------------------------------------------------*/
/* Set CTSE and RTSE bits according to USART_HardwareFlowControl value */
tmpreg |= USART_InitStruct->USART_HardwareFlowControl;
/* Write to USART CR3 */
USARTx->CR3 = (uint16_t)tmpreg;
/*---------------------------- USART BRR Configuration -----------------------*/
/* Configure the USART Baud Rate -------------------------------------------*/
RCC_GetClocksFreq(&RCC_ClocksStatus);
if (usartxbase == USART1_BASE)
{
apbclock = RCC_ClocksStatus.PCLK2_Frequency;
}
else
{
apbclock = RCC_ClocksStatus.PCLK1_Frequency;
}
/* Determine the integer part */
if ((USARTx->CR1 & CR1_OVER8_Set) != 0)
{
/* Integer part computing in case Oversampling mode is 8 Samples */
integerdivider = ((25 * apbclock) / (2 * (USART_InitStruct->USART_BaudRate)));
}
else /* if ((USARTx->CR1 & CR1_OVER8_Set) == 0) */
{
/* Integer part computing in case Oversampling mode is 16 Samples */
integerdivider = ((25 * apbclock) / (4 * (USART_InitStruct->USART_BaudRate)));
}
tmpreg = (integerdivider / 100) << 4;
/* Determine the fractional part */
fractionaldivider = integerdivider - (100 * (tmpreg >> 4));
/* Implement the fractional part in the register */
if ((USARTx->CR1 & CR1_OVER8_Set) != 0)
{
tmpreg |= ((((fractionaldivider * 8) + 50) / 100)) & ((uint8_t)0x07);
}
else /* if ((USARTx->CR1 & CR1_OVER8_Set) == 0) */
{
tmpreg |= ((((fractionaldivider * 16) + 50) / 100)) & ((uint8_t)0x0F);
}
/* Write to USART BRR */
USARTx->BRR = (uint16_t)tmpreg;
}
/**
* @brief Fills each USART_InitStruct member with its default value.
* @param USART_InitStruct: pointer to a USART_InitTypeDef structure
* which will be initialized.
* @retval None
*/
void USART_StructInit(USART_InitTypeDef* USART_InitStruct)
{
/* USART_InitStruct members default value */
USART_InitStruct->USART_BaudRate = 9600;
USART_InitStruct->USART_WordLength = USART_WordLength_8b;
USART_InitStruct->USART_StopBits = USART_StopBits_1;
USART_InitStruct->USART_Parity = USART_Parity_No ;
USART_InitStruct->USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_InitStruct->USART_HardwareFlowControl = USART_HardwareFlowControl_None;
}
/**
* @brief Initializes the USARTx peripheral Clock according to the
* specified parameters in the USART_ClockInitStruct .
* @param USARTx: where x can be 1, 2, 3 to select the USART peripheral.
* @param USART_ClockInitStruct: pointer to a USART_ClockInitTypeDef
* structure that contains the configuration information for the specified
* USART peripheral.
* @note The Smart Card and Synchronous modes are not available for UART4 and UART5.
* @retval None
*/
void USART_ClockInit(USART_TypeDef* USARTx, USART_ClockInitTypeDef* USART_ClockInitStruct)
{
uint32_t tmpreg = 0x00;
/* Check the parameters */
assert_param(IS_USART_123_PERIPH(USARTx));
assert_param(IS_USART_CLOCK(USART_ClockInitStruct->USART_Clock));
assert_param(IS_USART_CPOL(USART_ClockInitStruct->USART_CPOL));
assert_param(IS_USART_CPHA(USART_ClockInitStruct->USART_CPHA));
assert_param(IS_USART_LASTBIT(USART_ClockInitStruct->USART_LastBit));
/*---------------------------- USART CR2 Configuration -----------------------*/
tmpreg = USARTx->CR2;
/* Clear CLKEN, CPOL, CPHA and LBCL bits */
tmpreg &= CR2_CLOCK_CLEAR_Mask;
/* Configure the USART Clock, CPOL, CPHA and LastBit ------------*/
/* Set CLKEN bit according to USART_Clock value */
/* Set CPOL bit according to USART_CPOL value */
/* Set CPHA bit according to USART_CPHA value */
/* Set LBCL bit according to USART_LastBit value */
tmpreg |= (uint32_t)USART_ClockInitStruct->USART_Clock | USART_ClockInitStruct->USART_CPOL |
USART_ClockInitStruct->USART_CPHA | USART_ClockInitStruct->USART_LastBit;
/* Write to USART CR2 */
USARTx->CR2 = (uint16_t)tmpreg;
}
/**
* @brief Fills each USART_ClockInitStruct member with its default value.
* @param USART_ClockInitStruct: pointer to a USART_ClockInitTypeDef
* structure which will be initialized.
* @retval None
*/
void USART_ClockStructInit(USART_ClockInitTypeDef* USART_ClockInitStruct)
{
/* USART_ClockInitStruct members default value */
USART_ClockInitStruct->USART_Clock = USART_Clock_Disable;
USART_ClockInitStruct->USART_CPOL = USART_CPOL_Low;
USART_ClockInitStruct->USART_CPHA = USART_CPHA_1Edge;
USART_ClockInitStruct->USART_LastBit = USART_LastBit_Disable;
}
/**
* @brief Enables or disables the specified USART peripheral.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the USARTx peripheral.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_Cmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the selected USART by setting the UE bit in the CR1 register */
USARTx->CR1 |= CR1_UE_Set;
}
else
{
/* Disable the selected USART by clearing the UE bit in the CR1 register */
USARTx->CR1 &= CR1_UE_Reset;
}
}
/**
* @brief Enables or disables the specified USART interrupts.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_IT: specifies the USART interrupt sources to be enabled or disabled.
* This parameter can be one of the following values:
* @arg USART_IT_CTS: CTS change interrupt (not available for UART4 and UART5)
* @arg USART_IT_LBD: LIN Break detection interrupt
* @arg USART_IT_TXE: Transmit Data Register empty interrupt
* @arg USART_IT_TC: Transmission complete interrupt
* @arg USART_IT_RXNE: Receive Data register not empty interrupt
* @arg USART_IT_IDLE: Idle line detection interrupt
* @arg USART_IT_PE: Parity Error interrupt
* @arg USART_IT_ERR: Error interrupt(Frame error, noise error, overrun error)
* @param NewState: new state of the specified USARTx interrupts.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState)
{
uint32_t usartreg = 0x00, itpos = 0x00, itmask = 0x00;
uint32_t usartxbase = 0x00;
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_CONFIG_IT(USART_IT));
assert_param(IS_FUNCTIONAL_STATE(NewState));
/* The CTS interrupt is not available for UART4 and UART5 */
if (USART_IT == USART_IT_CTS)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
usartxbase = (uint32_t)USARTx;
/* Get the USART register index */
usartreg = (((uint8_t)USART_IT) >> 0x05);
/* Get the interrupt position */
itpos = USART_IT & IT_Mask;
itmask = (((uint32_t)0x01) << itpos);
if (usartreg == 0x01) /* The IT is in CR1 register */
{
usartxbase += 0x0C;
}
else if (usartreg == 0x02) /* The IT is in CR2 register */
{
usartxbase += 0x10;
}
else /* The IT is in CR3 register */
{
usartxbase += 0x14;
}
if (NewState != DISABLE)
{
*(__IO uint32_t*)usartxbase |= itmask;
}
else
{
*(__IO uint32_t*)usartxbase &= ~itmask;
}
}
/**
* @brief Enables or disables the USART’s DMA interface.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_DMAReq: specifies the DMA request.
* This parameter can be any combination of the following values:
* @arg USART_DMAReq_Tx: USART DMA transmit request
* @arg USART_DMAReq_Rx: USART DMA receive request
* @param NewState: new state of the DMA Request sources.
* This parameter can be: ENABLE or DISABLE.
* @note The DMA mode is not available for UART5 except in the STM32
* High density value line devices(STM32F10X_HD_VL).
* @retval None
*/
void USART_DMACmd(USART_TypeDef* USARTx, uint16_t USART_DMAReq, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_DMAREQ(USART_DMAReq));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the DMA transfer for selected requests by setting the DMAT and/or
DMAR bits in the USART CR3 register */
USARTx->CR3 |= USART_DMAReq;
}
else
{
/* Disable the DMA transfer for selected requests by clearing the DMAT and/or
DMAR bits in the USART CR3 register */
USARTx->CR3 &= (uint16_t)~USART_DMAReq;
}
}
/**
* @brief Sets the address of the USART node.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_Address: Indicates the address of the USART node.
* @retval None
*/
void USART_SetAddress(USART_TypeDef* USARTx, uint8_t USART_Address)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_ADDRESS(USART_Address));
/* Clear the USART address */
USARTx->CR2 &= CR2_Address_Mask;
/* Set the USART address node */
USARTx->CR2 |= USART_Address;
}
/**
* @brief Selects the USART WakeUp method.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_WakeUp: specifies the USART wakeup method.
* This parameter can be one of the following values:
* @arg USART_WakeUp_IdleLine: WakeUp by an idle line detection
* @arg USART_WakeUp_AddressMark: WakeUp by an address mark
* @retval None
*/
void USART_WakeUpConfig(USART_TypeDef* USARTx, uint16_t USART_WakeUp)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_WAKEUP(USART_WakeUp));
USARTx->CR1 &= CR1_WAKE_Mask;
USARTx->CR1 |= USART_WakeUp;
}
/**
* @brief Determines if the USART is in mute mode or not.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the USART mute mode.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_ReceiverWakeUpCmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the USART mute mode by setting the RWU bit in the CR1 register */
USARTx->CR1 |= CR1_RWU_Set;
}
else
{
/* Disable the USART mute mode by clearing the RWU bit in the CR1 register */
USARTx->CR1 &= CR1_RWU_Reset;
}
}
/**
* @brief Sets the USART LIN Break detection length.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_LINBreakDetectLength: specifies the LIN break detection length.
* This parameter can be one of the following values:
* @arg USART_LINBreakDetectLength_10b: 10-bit break detection
* @arg USART_LINBreakDetectLength_11b: 11-bit break detection
* @retval None
*/
void USART_LINBreakDetectLengthConfig(USART_TypeDef* USARTx, uint16_t USART_LINBreakDetectLength)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_LIN_BREAK_DETECT_LENGTH(USART_LINBreakDetectLength));
USARTx->CR2 &= CR2_LBDL_Mask;
USARTx->CR2 |= USART_LINBreakDetectLength;
}
/**
* @brief Enables or disables the USART’s LIN mode.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the USART LIN mode.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_LINCmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the LIN mode by setting the LINEN bit in the CR2 register */
USARTx->CR2 |= CR2_LINEN_Set;
}
else
{
/* Disable the LIN mode by clearing the LINEN bit in the CR2 register */
USARTx->CR2 &= CR2_LINEN_Reset;
}
}
/**
* @brief Transmits single data through the USARTx peripheral.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param Data: the data to transmit.
* @retval None
*/
void USART_SendData(USART_TypeDef* USARTx, uint16_t Data)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_DATA(Data));
/* Transmit Data */
USARTx->DR = (Data & (uint16_t)0x01FF);
}
/**
* @brief Returns the most recent received data by the USARTx peripheral.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @retval The received data.
*/
uint16_t USART_ReceiveData(USART_TypeDef* USARTx)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
/* Receive Data */
return (uint16_t)(USARTx->DR & (uint16_t)0x01FF);
}
/**
* @brief Transmits break characters.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @retval None
*/
void USART_SendBreak(USART_TypeDef* USARTx)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
/* Send break characters */
USARTx->CR1 |= CR1_SBK_Set;
}
/**
* @brief Sets the specified USART guard time.
* @param USARTx: where x can be 1, 2 or 3 to select the USART peripheral.
* @param USART_GuardTime: specifies the guard time.
* @note The guard time bits are not available for UART4 and UART5.
* @retval None
*/
void USART_SetGuardTime(USART_TypeDef* USARTx, uint8_t USART_GuardTime)
{
/* Check the parameters */
assert_param(IS_USART_123_PERIPH(USARTx));
/* Clear the USART Guard time */
USARTx->GTPR &= GTPR_LSB_Mask;
/* Set the USART guard time */
USARTx->GTPR |= (uint16_t)((uint16_t)USART_GuardTime << 0x08);
}
/**
* @brief Sets the system clock prescaler.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_Prescaler: specifies the prescaler clock.
* @note The function is used for IrDA mode with UART4 and UART5.
* @retval None
*/
void USART_SetPrescaler(USART_TypeDef* USARTx, uint8_t USART_Prescaler)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
/* Clear the USART prescaler */
USARTx->GTPR &= GTPR_MSB_Mask;
/* Set the USART prescaler */
USARTx->GTPR |= USART_Prescaler;
}
/**
* @brief Enables or disables the USART’s Smart Card mode.
* @param USARTx: where x can be 1, 2 or 3 to select the USART peripheral.
* @param NewState: new state of the Smart Card mode.
* This parameter can be: ENABLE or DISABLE.
* @note The Smart Card mode is not available for UART4 and UART5.
* @retval None
*/
void USART_SmartCardCmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_123_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the SC mode by setting the SCEN bit in the CR3 register */
USARTx->CR3 |= CR3_SCEN_Set;
}
else
{
/* Disable the SC mode by clearing the SCEN bit in the CR3 register */
USARTx->CR3 &= CR3_SCEN_Reset;
}
}
/**
* @brief Enables or disables NACK transmission.
* @param USARTx: where x can be 1, 2 or 3 to select the USART peripheral.
* @param NewState: new state of the NACK transmission.
* This parameter can be: ENABLE or DISABLE.
* @note The Smart Card mode is not available for UART4 and UART5.
* @retval None
*/
void USART_SmartCardNACKCmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_123_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the NACK transmission by setting the NACK bit in the CR3 register */
USARTx->CR3 |= CR3_NACK_Set;
}
else
{
/* Disable the NACK transmission by clearing the NACK bit in the CR3 register */
USARTx->CR3 &= CR3_NACK_Reset;
}
}
/**
* @brief Enables or disables the USART’s Half Duplex communication.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the USART Communication.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_HalfDuplexCmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the Half-Duplex mode by setting the HDSEL bit in the CR3 register */
USARTx->CR3 |= CR3_HDSEL_Set;
}
else
{
/* Disable the Half-Duplex mode by clearing the HDSEL bit in the CR3 register */
USARTx->CR3 &= CR3_HDSEL_Reset;
}
}
/**
* @brief Enables or disables the USART's 8x oversampling mode.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the USART one bit sampling method.
* This parameter can be: ENABLE or DISABLE.
* @note
* This function has to be called before calling USART_Init()
* function in order to have correct baudrate Divider value.
* @retval None
*/
void USART_OverSampling8Cmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the 8x Oversampling mode by setting the OVER8 bit in the CR1 register */
USARTx->CR1 |= CR1_OVER8_Set;
}
else
{
/* Disable the 8x Oversampling mode by clearing the OVER8 bit in the CR1 register */
USARTx->CR1 &= CR1_OVER8_Reset;
}
}
/**
* @brief Enables or disables the USART's one bit sampling method.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the USART one bit sampling method.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_OneBitMethodCmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the one bit method by setting the ONEBITE bit in the CR3 register */
USARTx->CR3 |= CR3_ONEBITE_Set;
}
else
{
/* Disable tthe one bit method by clearing the ONEBITE bit in the CR3 register */
USARTx->CR3 &= CR3_ONEBITE_Reset;
}
}
/**
* @brief Configures the USART's IrDA interface.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_IrDAMode: specifies the IrDA mode.
* This parameter can be one of the following values:
* @arg USART_IrDAMode_LowPower
* @arg USART_IrDAMode_Normal
* @retval None
*/
void USART_IrDAConfig(USART_TypeDef* USARTx, uint16_t USART_IrDAMode)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_IRDA_MODE(USART_IrDAMode));
USARTx->CR3 &= CR3_IRLP_Mask;
USARTx->CR3 |= USART_IrDAMode;
}
/**
* @brief Enables or disables the USART's IrDA interface.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param NewState: new state of the IrDA mode.
* This parameter can be: ENABLE or DISABLE.
* @retval None
*/
void USART_IrDACmd(USART_TypeDef* USARTx, FunctionalState NewState)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the IrDA mode by setting the IREN bit in the CR3 register */
USARTx->CR3 |= CR3_IREN_Set;
}
else
{
/* Disable the IrDA mode by clearing the IREN bit in the CR3 register */
USARTx->CR3 &= CR3_IREN_Reset;
}
}
/**
* @brief Checks whether the specified USART flag is set or not.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_FLAG: specifies the flag to check.
* This parameter can be one of the following values:
* @arg USART_FLAG_CTS: CTS Change flag (not available for UART4 and UART5)
* @arg USART_FLAG_LBD: LIN Break detection flag
* @arg USART_FLAG_TXE: Transmit data register empty flag
* @arg USART_FLAG_TC: Transmission Complete flag
* @arg USART_FLAG_RXNE: Receive data register not empty flag
* @arg USART_FLAG_IDLE: Idle Line detection flag
* @arg USART_FLAG_ORE: OverRun Error flag
* @arg USART_FLAG_NE: Noise Error flag
* @arg USART_FLAG_FE: Framing Error flag
* @arg USART_FLAG_PE: Parity Error flag
* @retval The new state of USART_FLAG (SET or RESET).
*/
FlagStatus USART_GetFlagStatus(USART_TypeDef* USARTx, uint16_t USART_FLAG)
{
FlagStatus bitstatus = RESET;
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_FLAG(USART_FLAG));
/* The CTS flag is not available for UART4 and UART5 */
if (USART_FLAG == USART_FLAG_CTS)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
if ((USARTx->SR & USART_FLAG) != (uint16_t)RESET)
{
bitstatus = SET;
}
else
{
bitstatus = RESET;
}
return bitstatus;
}
/**
* @brief Clears the USARTx's pending flags.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_FLAG: specifies the flag to clear.
* This parameter can be any combination of the following values:
* @arg USART_FLAG_CTS: CTS Change flag (not available for UART4 and UART5).
* @arg USART_FLAG_LBD: LIN Break detection flag.
* @arg USART_FLAG_TC: Transmission Complete flag.
* @arg USART_FLAG_RXNE: Receive data register not empty flag.
*
* @note
* - PE (Parity error), FE (Framing error), NE (Noise error), ORE (OverRun
* error) and IDLE (Idle line detected) flags are cleared by software
* sequence: a read operation to USART_SR register (USART_GetFlagStatus())
* followed by a read operation to USART_DR register (USART_ReceiveData()).
* - RXNE flag can be also cleared by a read to the USART_DR register
* (USART_ReceiveData()).
* - TC flag can be also cleared by software sequence: a read operation to
* USART_SR register (USART_GetFlagStatus()) followed by a write operation
* to USART_DR register (USART_SendData()).
* - TXE flag is cleared only by a write to the USART_DR register
* (USART_SendData()).
* @retval None
*/
void USART_ClearFlag(USART_TypeDef* USARTx, uint16_t USART_FLAG)
{
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_CLEAR_FLAG(USART_FLAG));
/* The CTS flag is not available for UART4 and UART5 */
if ((USART_FLAG & USART_FLAG_CTS) == USART_FLAG_CTS)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
USARTx->SR = (uint16_t)~USART_FLAG;
}
/**
* @brief Checks whether the specified USART interrupt has occurred or not.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_IT: specifies the USART interrupt source to check.
* This parameter can be one of the following values:
* @arg USART_IT_CTS: CTS change interrupt (not available for UART4 and UART5)
* @arg USART_IT_LBD: LIN Break detection interrupt
* @arg USART_IT_TXE: Tansmit Data Register empty interrupt
* @arg USART_IT_TC: Transmission complete interrupt
* @arg USART_IT_RXNE: Receive Data register not empty interrupt
* @arg USART_IT_IDLE: Idle line detection interrupt
* @arg USART_IT_ORE: OverRun Error interrupt
* @arg USART_IT_NE: Noise Error interrupt
* @arg USART_IT_FE: Framing Error interrupt
* @arg USART_IT_PE: Parity Error interrupt
* @retval The new state of USART_IT (SET or RESET).
*/
ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT)
{
uint32_t bitpos = 0x00, itmask = 0x00, usartreg = 0x00;
ITStatus bitstatus = RESET;
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_GET_IT(USART_IT));
/* The CTS interrupt is not available for UART4 and UART5 */
if (USART_IT == USART_IT_CTS)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
/* Get the USART register index */
usartreg = (((uint8_t)USART_IT) >> 0x05);
/* Get the interrupt position */
itmask = USART_IT & IT_Mask;
itmask = (uint32_t)0x01 << itmask;
if (usartreg == 0x01) /* The IT is in CR1 register */
{
itmask &= USARTx->CR1;
}
else if (usartreg == 0x02) /* The IT is in CR2 register */
{
itmask &= USARTx->CR2;
}
else /* The IT is in CR3 register */
{
itmask &= USARTx->CR3;
}
bitpos = USART_IT >> 0x08;
bitpos = (uint32_t)0x01 << bitpos;
bitpos &= USARTx->SR;
if ((itmask != (uint16_t)RESET)&&(bitpos != (uint16_t)RESET))
{
bitstatus = SET;
}
else
{
bitstatus = RESET;
}
return bitstatus;
}
/**
* @brief Clears the USARTx's interrupt pending bits.
* @param USARTx: Select the USART or the UART peripheral.
* This parameter can be one of the following values:
* USART1, USART2, USART3, UART4 or UART5.
* @param USART_IT: specifies the interrupt pending bit to clear.
* This parameter can be one of the following values:
* @arg USART_IT_CTS: CTS change interrupt (not available for UART4 and UART5)
* @arg USART_IT_LBD: LIN Break detection interrupt
* @arg USART_IT_TC: Transmission complete interrupt.
* @arg USART_IT_RXNE: Receive Data register not empty interrupt.
*
* @note
* - PE (Parity error), FE (Framing error), NE (Noise error), ORE (OverRun
* error) and IDLE (Idle line detected) pending bits are cleared by
* software sequence: a read operation to USART_SR register
* (USART_GetITStatus()) followed by a read operation to USART_DR register
* (USART_ReceiveData()).
* - RXNE pending bit can be also cleared by a read to the USART_DR register
* (USART_ReceiveData()).
* - TC pending bit can be also cleared by software sequence: a read
* operation to USART_SR register (USART_GetITStatus()) followed by a write
* operation to USART_DR register (USART_SendData()).
* - TXE pending bit is cleared only by a write to the USART_DR register
* (USART_SendData()).
* @retval None
*/
void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT)
{
uint16_t bitpos = 0x00, itmask = 0x00;
/* Check the parameters */
assert_param(IS_USART_ALL_PERIPH(USARTx));
assert_param(IS_USART_CLEAR_IT(USART_IT));
/* The CTS interrupt is not available for UART4 and UART5 */
if (USART_IT == USART_IT_CTS)
{
assert_param(IS_USART_123_PERIPH(USARTx));
}
bitpos = USART_IT >> 0x08;
itmask = ((uint16_t)0x01 << (uint16_t)bitpos);
USARTx->SR = (uint16_t)~itmask;
}
/**
* @}
*/
/**
* @}
*/
/**
* @}
*/
/******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
手机端需下载蓝牙调试器APP
连接密码一般为1234(具体看其他博主)
09 OLED显示屏
接线
GND-------GND
VCC-------3.3V
D0--------PB6
D1--------PB7
RES-------VCC
DC--------GND
CS--------GND
一定要接3.3V,不然会烧坏OLED
原理
文字取模工具
阿里云盘链接:阿里云盘分享
1.PCtoLCD界面
2.字模提取v2.1界面
字模提取的使用方法+字模提取V2.1 - 豌豆茶 - 博客园
main
#include "stm32f10x.h"
#include "bsp_usart.h"
#include "./i2c/bsp_i2c.h"
#include "delay.h"
#include "sys.h"
#include "oled.h"
#include "bmp.h"
#include "bsp_led.h"
uint8_t readData[10]={0};
uint8_t writeData[8]={4,5,6,7,8,9,10,11};
/**
* @brief Ö÷º¯Êý
* @param ÎÞ
* @retval ÎÞ
*/
int main(void)
{
u8 t=' ';
delay_init();
OLED_Init();
OLED_DisPlay_On();
OLED_ColorTurn(0);//0Õý³£ÏÔʾ£¬1 ·´É«ÏÔʾ
OLED_DisplayTurn(0);//0Õý³£ÏÔʾ 1 ÆÁÄ»·×ªÏÔʾ
LED0=0;
OLED_Refresh();
delay_ms(500);
OLED_Clear();
OLED_ShowChinese(0,0,0,16,1);//zhao
OLED_ShowChinese(16,0,1,16,1);//qing
OLED_ShowChinese(32,0,2,16,1);//xue
OLED_ShowChinese(48,0,3,16,1);//yuan
OLED_ShowNum(0,18,2,8,1,1);//2
OLED_ShowNum(8,18,0,8,1,1);//0
OLED_ShowNum(16,18,2,8,1,1);//2
OLED_ShowNum(24,18,2,8,1,1);//2
OLED_ShowNum(32,18,2,8,1,1);//2
OLED_ShowNum(40,18,4,8,1,1);//4
OLED_ShowNum(48,18,1,8,1,1);//1
OLED_ShowNum(56,18,0,8,1,1);//0
OLED_ShowNum(64,18,2,8,1,1);//2
OLED_ShowNum(72,18,1,8,1,1);//1
OLED_ShowNum(80,18,0,8,1,1);//0
OLED_ShowNum(88,18,1,8,1,1);//1
OLED_ShowChinese(0,28,4,16,1);//wang
OLED_ShowChinese(16,28,5,16,1);//bai
OLED_ShowChinese(32,28,6,16,1);//he
while(1)
{
}
}
/*********************************************END OF FILE**********************/
bsp_usart.c
#include "bsp_usart.h"
/**
* @brief ÅäÖÃǶÌ×ÏòÁ¿ÖжϿØÖÆÆ÷NVIC
* @param ÎÞ
* @retval ÎÞ
*/
//static void NVIC_Configuration(void)
//{
// NVIC_InitTypeDef NVIC_InitStructure;
//
// /* ǶÌ×ÏòÁ¿ÖжϿØÖÆÆ÷×éÑ¡Ôñ */
// NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
//
// /* ÅäÖÃUSARTΪÖжÏÔ´ */
// NVIC_InitStructure.NVIC_IRQChannel = USART_IRQ;
// /* ÇÀ¶ÏÓÅÏȼ¶*/
// NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
// /* ×ÓÓÅÏȼ¶ */
// NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
// /* ʹÄÜÖÐ¶Ï */
// NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
// /* ³õʼ»¯ÅäÖÃNVIC */
// NVIC_Init(&NVIC_InitStructure);
//}
/**
* @brief USART GPIO ÅäÖÃ,¹¤×÷²ÎÊýÅäÖÃ
* @param ÎÞ
* @retval ÎÞ
*/
void USART_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// ´ò¿ª´®¿ÚGPIOµÄʱÖÓ
USART_GPIO_APBxClkCmd(USART_GPIO_CLK, ENABLE);
// ´ò¿ª´®¿ÚÍâÉèµÄʱÖÓ
USART_APBxClkCmd(USART_CLK, ENABLE);
// ½«USART TxµÄGPIOÅäÖÃÎªÍÆÍ츴ÓÃģʽ
GPIO_InitStructure.GPIO_Pin = USART_TX_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(USART_TX_GPIO_PORT, &GPIO_InitStructure);
// ½«USART RxµÄGPIOÅäÖÃΪ¸¡¿ÕÊäÈëģʽ
GPIO_InitStructure.GPIO_Pin = USART_RX_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(USART_RX_GPIO_PORT, &GPIO_InitStructure);
// ÅäÖô®¿ÚµÄ¹¤×÷²ÎÊý
// ÅäÖò¨ÌØÂÊ
USART_InitStructure.USART_BaudRate = USART_BAUDRATE;
// ÅäÖà ÕëÊý¾Ý×Ö³¤
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
// ÅäÖÃֹͣλ
USART_InitStructure.USART_StopBits = USART_StopBits_1;
// ÅäÖÃУÑéλ
USART_InitStructure.USART_Parity = USART_Parity_No ;
// ÅäÖÃÓ²¼þÁ÷¿ØÖÆ
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
// ÅäÖù¤×÷ģʽ£¬ÊÕ·¢Ò»Æð
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
// Íê³É´®¿ÚµÄ³õʼ»¯ÅäÖÃ
USART_Init(USARTx, &USART_InitStructure);
// ´®¿ÚÖжÏÓÅÏȼ¶ÅäÖÃ
// NVIC_Configuration();
//
// // ʹÄÜ´®¿Ú½ÓÊÕÖжÏ
// USART_ITConfig(USARTx, USART_IT_RXNE, ENABLE);
// ʹÄÜ´®¿Ú
USART_Cmd(USARTx, ENABLE);
}
/***************** ·¢ËÍÒ»¸ö×Ö·û **********************/
void Usart_SendByte( USART_TypeDef * pUSARTx, uint8_t ch)
{
/* ·¢ËÍÒ»¸ö×Ö½ÚÊý¾Ýµ½USART */
USART_SendData(pUSARTx,ch);
/* µÈ´ý·¢ËÍÊý¾Ý¼Ä´æÆ÷Ϊ¿Õ */
while (USART_GetFlagStatus(pUSARTx, USART_FLAG_TXE) == RESET);
}
/***************** ·¢ËÍ×Ö·û´® **********************/
void Usart_SendString( USART_TypeDef * pUSARTx, char *str)
{
unsigned int k=0;
do
{
Usart_SendByte( pUSARTx, *(str + k) );
k++;
} while(*(str + k)!='\0');
/* µÈ´ý·¢ËÍÍê³É */
while(USART_GetFlagStatus(pUSARTx,USART_FLAG_TC)==RESET)
{}
}
/***************** ·¢ËÍÒ»¸ö16λÊý **********************/
void Usart_SendHalfWord( USART_TypeDef * pUSARTx, uint16_t ch)
{
uint8_t temp_h, temp_l;
/* È¡³ö¸ß°Ëλ */
temp_h = (ch&0XFF00)>>8;
/* È¡³öµÍ°Ëλ */
temp_l = ch&0XFF;
/* ·¢Ë͸߰Ëλ */
USART_SendData(pUSARTx,temp_h);
while (USART_GetFlagStatus(pUSARTx, USART_FLAG_TXE) == RESET);
/* ·¢Ë͵ͰËλ */
USART_SendData(pUSARTx,temp_l);
while (USART_GetFlagStatus(pUSARTx, USART_FLAG_TXE) == RESET);
}
///ÖØ¶¨Ïòc¿âº¯Êýprintfµ½´®¿Ú£¬Öض¨Ïòºó¿ÉʹÓÃprintfº¯Êý
int fputc(int ch, FILE *f)
{
/* ·¢ËÍÒ»¸ö×Ö½ÚÊý¾Ýµ½´®¿Ú */
USART_SendData(USARTx, (uint8_t) ch);
/* µÈ´ý·¢ËÍÍê±Ï */
while (USART_GetFlagStatus(USARTx, USART_FLAG_TXE) == RESET);
return (ch);
}
///ÖØ¶¨Ïòc¿âº¯Êýscanfµ½´®¿Ú£¬ÖØÐ´Ïòºó¿ÉʹÓÃscanf¡¢getcharµÈº¯Êý
int fgetc(FILE *f)
{
/* µÈ´ý´®¿ÚÊäÈëÊý¾Ý */
while (USART_GetFlagStatus(USARTx, USART_FLAG_RXNE) == RESET);
return (int)USART_ReceiveData(USARTx);
}
bsp_usart.h
#ifndef __USART_H
#define __USART_H
#include "stm32f10x.h"
#include <stdio.h>
/**
* ´®¿Úºê¶¨Ò壬²»Í¬µÄ´®¿Ú¹ÒÔØµÄ×ÜÏߺÍIO²»Ò»Ñù£¬ÒÆÖ²Ê±ÐèÒªÐÞ¸ÄÕ⼸¸öºê
* 1-ÐÞ¸Ä×ÜÏßʱÖӵĺ꣬uart1¹ÒÔØµ½apb2×ÜÏߣ¬ÆäËûuart¹ÒÔØµ½apb1×ÜÏß
* 2-ÐÞ¸ÄGPIOµÄºê
*/
// ´®¿Ú1-USART1
#define USARTx USART1
#define USART_CLK RCC_APB2Periph_USART1
#define USART_APBxClkCmd RCC_APB2PeriphClockCmd
#define USART_BAUDRATE 115200
// USART GPIO Òý½Åºê¶¨Òå
#define USART_GPIO_CLK (RCC_APB2Periph_GPIOA)
#define USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
#define USART_TX_GPIO_PORT GPIOA
#define USART_TX_GPIO_PIN GPIO_Pin_9
#define USART_RX_GPIO_PORT GPIOA
#define USART_RX_GPIO_PIN GPIO_Pin_10
#define USART_IRQ USART1_IRQn
#define USART_IRQHandler USART1_IRQHandler
// ´®¿Ú2-USART2
//#define USARTx USART2
//#define USART_CLK RCC_APB1Periph_USART2
//#define USART_APBxClkCmd RCC_APB1PeriphClockCmd
//#define USART_BAUDRATE 115200
USART GPIO Òý½Åºê¶¨Òå
//#define USART_GPIO_CLK (RCC_APB2Periph_GPIOA)
//#define USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
//
//#define USART_TX_GPIO_PORT GPIOA
//#define USART_TX_GPIO_PIN GPIO_Pin_2
//#define USART_RX_GPIO_PORT GPIOA
//#define USART_RX_GPIO_PIN GPIO_Pin_3
//#define USART_IRQ USART2_IRQn
//#define USART_IRQHandler USART2_IRQHandler
// ´®¿Ú3-USART3
//#define USARTx USART3
//#define USART_CLK RCC_APB1Periph_USART3
//#define USART_APBxClkCmd RCC_APB1PeriphClockCmd
//#define USART_BAUDRATE 115200
USART GPIO Òý½Åºê¶¨Òå
//#define USART_GPIO_CLK (RCC_APB2Periph_GPIOB)
//#define USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
//
//#define USART_TX_GPIO_PORT GPIOB
//#define USART_TX_GPIO_PIN GPIO_Pin_10
//#define USART_RX_GPIO_PORT GPIOB
//#define USART_RX_GPIO_PIN GPIO_Pin_11
//#define USART_IRQ USART3_IRQn
//#define USART_IRQHandler USART3_IRQHandler
// ´®¿Ú4-UART4
//#define USARTx UART4
//#define USART_CLK RCC_APB1Periph_UART4
//#define USART_APBxClkCmd RCC_APB1PeriphClockCmd
//#define USART_BAUDRATE 115200
USART GPIO Òý½Åºê¶¨Òå
//#define USART_GPIO_CLK (RCC_APB2Periph_GPIOC)
//#define USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
//
//#define USART_TX_GPIO_PORT GPIOC
//#define USART_TX_GPIO_PIN GPIO_Pin_10
//#define USART_RX_GPIO_PORT GPIOC
//#define USART_RX_GPIO_PIN GPIO_Pin_11
//#define USART_IRQ UART4_IRQn
//#define USART_IRQHandler UART4_IRQHandler
// ´®¿Ú5-UART5
//#define USARTx UART5
//#define USART_CLK RCC_APB1Periph_UART5
//#define USART_APBxClkCmd RCC_APB1PeriphClockCmd
//#define USART_BAUDRATE 115200
USART GPIO Òý½Åºê¶¨Òå
//#define USART_GPIO_CLK (RCC_APB2Periph_GPIOC|RCC_APB2Periph_GPIOD)
//#define USART_GPIO_APBxClkCmd RCC_APB2PeriphClockCmd
//
//#define USART_TX_GPIO_PORT GPIOC
//#define USART_TX_GPIO_PIN GPIO_Pin_12
//#define USART_RX_GPIO_PORT GPIOD
//#define USART_RX_GPIO_PIN GPIO_Pin_2
//#define USART_IRQ UART5_IRQn
//#define USART_IRQHandler UART5_IRQHandler
void USART_Config(void);
void Usart_SendByte( USART_TypeDef * pUSARTx, uint8_t ch);
void Usart_SendString( USART_TypeDef * pUSARTx, char *str);
void Usart_SendHalfWord( USART_TypeDef * pUSARTx, uint16_t ch);
#endif /* __USART_H */
stm32f10x_it.c
/**
******************************************************************************
* @file Project/STM32F10x_StdPeriph_Template/stm32f10x_it.c
* @author MCD Application Team
* @version V3.5.0
* @date 08-April-2011
* @brief Main Interrupt Service Routines.
* This file provides template for all exceptions handler and
* peripherals interrupt service routine.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTI
AL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x_it.h"
#include "bsp_usart.h"
/** @addtogroup STM32F10x_StdPeriph_Template
* @{
*/
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
/* Private functions ---------------------------------------------------------*/
/******************************************************************************/
/* Cortex-M3 Processor Exceptions Handlers */
/******************************************************************************/
/**
* @brief This function handles NMI exception.
* @param None
* @retval None
*/
void NMI_Handler(void)
{
}
/**
* @brief This function handles Hard Fault exception.
* @param None
* @retval None
*/
void HardFault_Handler(void)
{
/* Go to infinite loop when Hard Fault exception occurs */
while (1)
{
}
}
/**
* @brief This function handles Memory Manage exception.
* @param None
* @retval None
*/
void MemManage_Handler(void)
{
/* Go to infinite loop when Memory Manage exception occurs */
while (1)
{
}
}
/**
* @brief This function handles Bus Fault exception.
* @param None
* @retval None
*/
void BusFault_Handler(void)
{
/* Go to infinite loop when Bus Fault exception occurs */
while (1)
{
}
}
/**
* @brief This function handles Usage Fault exception.
* @param None
* @retval None
*/
void UsageFault_Handler(void)
{
/* Go to infinite loop when Usage Fault exception occurs */
while (1)
{
}
}
/**
* @brief This function handles SVCall exception.
* @param None
* @retval None
*/
void SVC_Handler(void)
{
}
/**
* @brief This function handles Debug Monitor exception.
* @param None
* @retval None
*/
void DebugMon_Handler(void)
{
}
/**
* @brief This function handles PendSVC exception.
* @param None
* @retval None
*/
void PendSV_Handler(void)
{
}
/**
* @brief This function handles SysTick Handler.
* @param None
* @retval None
*/
void SysTick_Handler(void)
{
}
// ´®¿ÚÖжϷþÎñº¯Êý
//void USART_IRQHandler(void)
//{
// uint8_t ucTemp;
// if(USART_GetITStatus(USARTx,USART_IT_RXNE)!=RESET)
// {
// ucTemp = USART_ReceiveData(USARTx);
// USART_SendData(USARTx,ucTemp);
// }
//}
/**
* @brief This function handles PPP interrupt request.
* @param None
* @retval None
*/
/*void PPP_IRQHandler(void)
{
}*/
/**
* @}
*/
/******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
stm32f10x_it.h
/**
******************************************************************************
* @file Project/STM32F10x_StdPeriph_Template/stm32f10x_it.h
* @author MCD Application Team
* @version V3.5.0
* @date 08-April-2011
* @brief This file contains the headers of the interrupt handlers.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Define to prevent recursive inclusion -------------------------------------*/
#ifndef __STM32F10x_IT_H
#define __STM32F10x_IT_H
#ifdef __cplusplus
extern "C" {
#endif
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x.h"
/* Exported types ------------------------------------------------------------*/
/* Exported constants --------------------------------------------------------*/
/* Exported macro ------------------------------------------------------------*/
/* Exported functions ------------------------------------------------------- */
void NMI_Handler(void);
void HardFault_Handler(void);
void MemManage_Handler(void);
void BusFault_Handler(void);
void UsageFault_Handler(void);
void SVC_Handler(void);
void DebugMon_Handler(void);
void PendSV_Handler(void);
void SysTick_Handler(void);
#ifdef __cplusplus
}
#endif
#endif /* __STM32F10x_IT_H */
/******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
bsp_i2c.c
/**
******************************************************************************
* @file bsp_i2c_ee.c
* @version V1.0
* @date 2013-xx-xx
* @brief ÓÃgpioÄ£Äâi2c×ÜÏß, ÊÊÓÃÓÚSTM32ϵÁÐCPU¡£¸ÃÄ£¿é²»°üÀ¨Ó¦ÓòãÃüÁîÖ¡£¬½ö°üÀ¨I2C×ÜÏß»ù±¾²Ù×÷º¯Êý¡£
******************************************************************************
* @attention
*
* ʵÑéÆ½Ì¨:Ò°»ð F103-Ö¸ÄÏÕß STM32 ¿ª·¢°å
* ÂÛ̳ :http://www.firebbs.cn
* ÌÔ±¦ :https://fire-stm32.taobao.com
*
******************************************************************************
*/
/*
Ó¦ÓÃ˵Ã÷£º
ÔÚ·ÃÎÊI2CÉ豸ǰ£¬ÇëÏȵ÷Óà i2c_CheckDevice() ¼ì²âI2CÉ豸ÊÇ·ñÕý³££¬¸Ãº¯Êý»áÅäÖÃGPIO
*/
#include "bsp_i2c.h"
#include "stm32f10x.h"
#include "oled.h"
static void i2c_CfgGpio(void);
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_Delay
* ¹¦ÄÜ˵Ã÷: I2C×ÜÏßλÑÓ³Ù£¬×î¿ì400KHz
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
static void i2c_Delay(void)
{
uint8_t i;
/*¡¡
ÏÂÃæµÄʱ¼äÊÇͨ¹ýÂß¼·ÖÎöÒDzâÊԵõ½µÄ¡£
¹¤×÷Ìõ¼þ£ºCPUÖ÷Ƶ72MHz £¬MDK±àÒë»·¾³£¬1¼¶ÓÅ»¯
Ñ»·´ÎÊýΪ10ʱ£¬SCLƵÂÊ = 205KHz
Ñ»·´ÎÊýΪ7ʱ£¬SCLƵÂÊ = 347KHz£¬ SCL¸ßµçƽʱ¼ä1.5us£¬SCLµÍµçƽʱ¼ä2.87us
Ñ»·´ÎÊýΪ5ʱ£¬SCLƵÂÊ = 421KHz£¬ SCL¸ßµçƽʱ¼ä1.25us£¬SCLµÍµçƽʱ¼ä2.375us
*/
for (i = 0; i < 10; i++);
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_Start
* ¹¦ÄÜ˵Ã÷: CPU·¢ÆðI2C×ÜÏ߯ô¶¯ÐźÅ
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
void i2c_Start(void)
{
/* µ±SCL¸ßµçƽʱ£¬SDA³öÏÖÒ»¸öÏÂÌøÑØ±íʾI2C×ÜÏ߯ô¶¯ÐźŠ*/
I2C_SDA_1();
I2C_SCL_1();
i2c_Delay();
I2C_SDA_0();
i2c_Delay();
I2C_SCL_0();
i2c_Delay();
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_Stop
* ¹¦ÄÜ˵Ã÷: CPU·¢ÆðI2C×ÜÏßÍ£Ö¹ÐźÅ
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
void i2c_Stop(void)
{
/* µ±SCL¸ßµçƽʱ£¬SDA³öÏÖÒ»¸öÉÏÌøÑØ±íʾI2C×ÜÏßÍ£Ö¹ÐźŠ*/
I2C_SDA_0();
I2C_SCL_1();
i2c_Delay();
I2C_SDA_1();
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_SendByte
* ¹¦ÄÜ˵Ã÷: CPUÏòI2C×ÜÏßÉ豸·¢ËÍ8bitÊý¾Ý
* ÐÎ ²Î£º_ucByte £º µÈ´ý·¢Ë͵Ä×Ö½Ú
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
void i2c_SendByte(uint8_t _ucByte)
{
uint8_t i;
/* ÏÈ·¢ËÍ×ֽڵĸßλbit7 */
for (i = 0; i < 8; i++)
{
if (_ucByte & 0x80)
{
I2C_SDA_1();
}
else
{
I2C_SDA_0();
}
i2c_Delay();
I2C_SCL_1();
i2c_Delay();
I2C_SCL_0();
if (i == 7)
{
I2C_SDA_1(); // ÊÍ·Å×ÜÏß
}
_ucByte <<= 1; /* ×óÒÆÒ»¸öbit */
i2c_Delay();
}
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_ReadByte
* ¹¦ÄÜ˵Ã÷: CPU´ÓI2C×ÜÏßÉ豸¶ÁÈ¡8bitÊý¾Ý
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ¶Áµ½µÄÊý¾Ý
*********************************************************************************************************
*/
uint8_t i2c_ReadByte(void)
{
uint8_t i;
uint8_t value;
/* ¶Áµ½µÚ1¸öbitΪÊý¾ÝµÄbit7 */
value = 0;
for (i = 0; i < 8; i++)
{
value <<= 1;
I2C_SCL_1();
i2c_Delay();
if ( I2C_SDA_READ())
{
value++;
}
I2C_SCL_0();
i2c_Delay();
}
return value;
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_WaitAck
* ¹¦ÄÜ˵Ã÷: CPU²úÉúÒ»¸öʱÖÓ£¬²¢¶ÁÈ¡Æ÷¼þµÄACKÓ¦´ðÐźÅ
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ·µ»Ø0±íʾÕýÈ·Ó¦´ð£¬1±íʾÎÞÆ÷¼þÏìÓ¦
*********************************************************************************************************
*/
uint8_t i2c_WaitAck(void)
{
uint8_t re;
I2C_SDA_1(); /* CPUÊÍ·ÅSDA×ÜÏß */
i2c_Delay();
I2C_SCL_1(); /* CPUÇý¶¯SCL = 1, ´ËʱÆ÷¼þ»á·µ»ØACKÓ¦´ð */
i2c_Delay();
if ( I2C_SDA_READ()) /* CPU¶ÁÈ¡SDA¿ÚÏß״̬ */
{
re = 1;
}
else
{
re = 0;
}
I2C_SCL_0();
i2c_Delay();
return re;
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_Ack
* ¹¦ÄÜ˵Ã÷: CPU²úÉúÒ»¸öACKÐźÅ
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
void i2c_Ack(void)
{
I2C_SDA_0(); /* CPUÇý¶¯SDA = 0 */
i2c_Delay();
I2C_SCL_1(); /* CPU²úÉú1¸öʱÖÓ */
i2c_Delay();
I2C_SCL_0();
i2c_Delay();
I2C_SDA_1(); /* CPUÊÍ·ÅSDA×ÜÏß */
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_NAck
* ¹¦ÄÜ˵Ã÷: CPU²úÉú1¸öNACKÐźÅ
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
void i2c_NAck(void)
{
I2C_SDA_1(); /* CPUÇý¶¯SDA = 1 */
i2c_Delay();
I2C_SCL_1(); /* CPU²úÉú1¸öʱÖÓ */
i2c_Delay();
I2C_SCL_0();
i2c_Delay();
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_CfgGpio
* ¹¦ÄÜ˵Ã÷: ÅäÖÃI2C×ÜÏßµÄGPIO£¬²ÉÓÃÄ£ÄâIOµÄ·½Ê½ÊµÏÖ
* ÐÎ ²Î£ºÎÞ
* ·µ »Ø Öµ: ÎÞ
*********************************************************************************************************
*/
void i2c_CfgGpio(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd( RCC_I2C_PORT, ENABLE); /* ´ò¿ªGPIOʱÖÓ */
GPIO_InitStructure.GPIO_Pin = I2C_SCL_PIN | I2C_SDA_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD; /* ¿ªÂ©Êä³ö */
GPIO_Init( GPIO_PORT_I2C, &GPIO_InitStructure);
/* ¸øÒ»¸öÍ£Ö¹ÐźÅ, ¸´Î»I2C×ÜÏßÉϵÄËùÓÐÉ豸µ½´ý»úģʽ */
i2c_Stop();
}
/*
*********************************************************************************************************
* º¯ Êý Ãû: i2c_CheckDevice
* ¹¦ÄÜ˵Ã÷: ¼ì²âI2C×ÜÏßÉ豸£¬CPUÏò·¢ËÍÉ豸µØÖ·£¬È»ºó¶ÁÈ¡É豸Ӧ´ðÀ´ÅжϸÃÉ豸ÊÇ·ñ´æÔÚ
* ÐÎ ²Î£º_Address£ºÉ豸µÄI2C×ÜÏßµØÖ·
* ·µ »Ø Öµ: ·µ»ØÖµ 0 ±íʾÕýÈ·£¬ ·µ»Ø1±íʾδ̽²âµ½
*********************************************************************************************************
*/
uint8_t i2c_CheckDevice(uint8_t _Address)
{
uint8_t ucAck;
i2c_CfgGpio(); /* ÅäÖÃGPIO */
i2c_Start(); /* ·¢ËÍÆô¶¯ÐźŠ*/
/* ·¢ËÍÉ豸µØÖ·+¶Áд¿ØÖÆbit£¨0 = w£¬ 1 = r) bit7 ÏÈ´« */
i2c_SendByte(_Address | I2C_WR);
ucAck = i2c_WaitAck(); /* ¼ì²âÉ豸µÄACKÓ¦´ð */
i2c_Stop(); /* ·¢ËÍÍ£Ö¹ÐźŠ*/
return ucAck;
}
/*
***********************************************************************************************************
* º¯ Êý Ãû:I2C Write byte
***********************************************************************************************************
*/
void Write_I2C_Byte(uint8_t IIC_Byte)
{
uint8_t i;
uint8_t m,da;
da=IIC_Byte;
I2C_SCL_0();
for(i=0;i<8;i++)
{
m=da;
// OLED_SCLK_Clr();
m=m&0x80;
if(m==0x80)
{
I2C_SDA_1();
}
else
I2C_SDA_0();
da=da<<1;
I2C_SCL_1();
I2C_SCL_0();
}
}
/*
***********************************************************************************************************
* º¯ Êý Ãû:I2C Write command
***********************************************************************************************************
*/
void Write_I2C_Command(uint8_t I2C_Command) //???
{
i2c_Start();
Write_I2C_Byte(0x78); // OLED?I2C??(????)
i2c_WaitAck();
Write_I2C_Byte(0x00); // OLED ??(????)
i2c_WaitAck();
Write_I2C_Byte(I2C_Command);
i2c_WaitAck();
i2c_Stop();
}
/*
***********************************************************************************************************
* º¯ Êý Ãû:Write_I2C_Data
***********************************************************************************************************
*/
void Write_I2C_Data(uint8_t IIC_Data) //???
{
i2c_Start();
Write_I2C_Byte(0x78); // OLED?I2C??(????)
i2c_WaitAck();
Write_I2C_Byte(0x40); // OLED ??(????)
i2c_WaitAck();
Write_I2C_Byte(IIC_Data);
i2c_WaitAck();
i2c_Stop();
}
/*
***********************************************************************************************************
* º¯ Êý Ãû:zuobiaoshezhi
***********************************************************************************************************
*/
void OLED_Set_Pos(uint8_t x, uint8_t y)
{
OLED_WR_Byte(0xb0+y,OLED_CMD);
OLED_WR_Byte(((x&0xf0)>>4)|0x10,OLED_CMD);
OLED_WR_Byte((x&0x0f),OLED_CMD);
}
/*
***********************************************************************************************************
* º¯ Êý Ãû:OLED_Display_On
***********************************************************************************************************
*/
void OLED_Display_On(void)
{
OLED_WR_Byte(0X8D,OLED_CMD); //SET DCDC??
OLED_WR_Byte(0X14,OLED_CMD); //DCDC ON
OLED_WR_Byte(0XAF,OLED_CMD); //DISPLAY ON
}
/*
***********************************************************************************************************
* º¯ Êý Ãû:OLED_Display_Off
***********************************************************************************************************
*/
//void OLED_Display_Off(void)
//{
// OLED_WR_Byte(0X8D,OLED_CMD); //SET DCDC??
// OLED_WR_Byte(0X10,OLED_CMD); //DCDC OFF
// OLED_WR_Byte(0XAE,OLED_CMD); //DISPLAY OFF
//}
bsp_i2c.h
#ifndef _BSP_I2C_GPIO_H
#define _BSP_I2C_GPIO_H
#include <inttypes.h>
#define I2C_WR 0 /* д¿ØÖÆbit */
#define I2C_RD 1 /* ¶Á¿ØÖÆbit */
/* ¶¨ÒåI2C×ÜÏßÁ¬½ÓµÄGPIO¶Ë¿Ú, Óû§Ö»ÐèÒªÐÞ¸ÄÏÂÃæ4ÐдúÂë¼´¿ÉÈÎÒâ¸Ä±äSCLºÍSDAµÄÒý½Å */
#define GPIO_PORT_I2C GPIOB /* GPIO¶Ë¿Ú */
#define RCC_I2C_PORT RCC_APB2Periph_GPIOB /* GPIO¶Ë¿ÚʱÖÓ */
#define I2C_SCL_PIN GPIO_Pin_6 /* Á¬½Óµ½SCLʱÖÓÏßµÄGPIO */
#define I2C_SDA_PIN GPIO_Pin_7 /* Á¬½Óµ½SDAÊý¾ÝÏßµÄGPIO */
/* ¶¨Òå¶ÁдSCLºÍSDAµÄºê£¬ÒÑÔö¼Ó´úÂëµÄ¿ÉÒÆÖ²ÐԺͿÉÔĶÁÐÔ */
#if 0 /* Ìõ¼þ±àÒ룺 1 Ñ¡ÔñGPIOµÄ¿âº¯ÊýʵÏÖIO¶Áд */
#define I2C_SCL_1() GPIO_SetBits( GPIO_PORT_I2C, I2C_SCL_PIN) /* SCL = 1 */
#define I2C_SCL_0() GPIO_ResetBits( GPIO_PORT_I2C, I2C_SCL_PIN) /* SCL = 0 */
#define I2C_SDA_1() GPIO_SetBits( GPIO_PORT_I2C, I2C_SDA_PIN) /* SDA = 1 */
#define I2C_SDA_0() GPIO_ResetBits( GPIO_PORT_I2C, I2C_SDA_PIN) /* SDA = 0 */
#define I2C_SDA_READ() GPIO_ReadInputDataBit( GPIO_PORT_I2C, I2C_SDA_PIN) /* ¶ÁSDA¿ÚÏß״̬ */
#else /* Õâ¸ö·ÖÖ§Ñ¡ÔñÖ±½Ó¼Ä´æÆ÷²Ù×÷ʵÏÖIO¶Áд */
/*¡¡×¢Ò⣺ÈçÏÂд·¨£¬ÔÚIAR×î¸ß¼¶±ðÓÅ»¯Ê±£¬»á±»±àÒëÆ÷´íÎóÓÅ»¯ */
#define I2C_SCL_1() GPIO_PORT_I2C->BSRR = I2C_SCL_PIN /* SCL = 1 */
#define I2C_SCL_0() GPIO_PORT_I2C->BRR = I2C_SCL_PIN /* SCL = 0 */
#define I2C_SDA_1() GPIO_PORT_I2C->BSRR = I2C_SDA_PIN /* SDA = 1 */
#define I2C_SDA_0() GPIO_PORT_I2C->BRR = I2C_SDA_PIN /* SDA = 0 */
#define I2C_SDA_READ() (( GPIO_PORT_I2C->IDR & I2C_SDA_PIN) != 0) /* ¶ÁSDA¿ÚÏß״̬ */
#endif
void i2c_Start(void);
void i2c_Stop(void);
void i2c_SendByte(uint8_t _ucByte);
uint8_t i2c_ReadByte(void);
uint8_t i2c_WaitAck(void);
void i2c_Ack(void);
void i2c_NAck(void);
uint8_t i2c_CheckDevice(uint8_t _Address);
#endif
oled.c
#include "oled.h"
#include "stdlib.h"
#include "oledfont.h"
#include "delay.h"
u8 OLED_GRAM[144][8];
//·´ÏÔº¯Êý
void OLED_ColorTurn(u8 i)
{
if(i==0)
{
OLED_WR_Byte(0xA6,OLED_CMD);//Õý³£ÏÔʾ
}
if(i==1)
{
OLED_WR_Byte(0xA7,OLED_CMD);//·´É«ÏÔʾ
}
}
//ÆÁÄ»Ðýת180¶È
void OLED_DisplayTurn(u8 i)
{
if(i==0)
{
OLED_WR_Byte(0xC8,OLED_CMD);//Õý³£ÏÔʾ
OLED_WR_Byte(0xA1,OLED_CMD);
}
if(i==1)
{
OLED_WR_Byte(0xC0,OLED_CMD);//·´×ªÏÔʾ
OLED_WR_Byte(0xA0,OLED_CMD);
}
}
//ÑÓʱ
void IIC_delay(void)
{
u8 t=3;
while(t--);
}
//ÆðʼÐźÅ
void I2C_Start(void)
{
OLED_SDA_Set();
OLED_SCL_Set();
IIC_delay();
OLED_SDA_Clr();
IIC_delay();
OLED_SCL_Clr();
IIC_delay();
}
//½áÊøÐźÅ
void I2C_Stop(void)
{
OLED_SDA_Clr();
OLED_SCL_Set();
IIC_delay();
OLED_SDA_Set();
}
//µÈ´ýÐźÅÏìÓ¦
void I2C_WaitAck(void) //²âÊý¾ÝÐÅºÅµÄµçÆ½
{
OLED_SDA_Set();
IIC_delay();
OLED_SCL_Set();
IIC_delay();
OLED_SCL_Clr();
IIC_delay();
}
//дÈëÒ»¸ö×Ö½Ú
void Send_Byte(u8 dat)
{
u8 i;
for(i=0;i<8;i++)
{
if(dat&0x80)//½«datµÄ8λ´Ó×î¸ßλÒÀ´ÎдÈë
{
OLED_SDA_Set();
}
else
{
OLED_SDA_Clr();
}
IIC_delay();
OLED_SCL_Set();
IIC_delay();
OLED_SCL_Clr();//½«Ê±ÖÓÐźÅÉèÖÃΪµÍµçƽ
dat<<=1;
}
}
//·¢ËÍÒ»¸ö×Ö½Ú
//mode:Êý¾Ý/ÃüÁî±êÖ¾ 0,±íʾÃüÁî;1,±íʾÊý¾Ý;
void OLED_WR_Byte(u8 dat,u8 mode)
{
I2C_Start();
Send_Byte(0x78);
I2C_WaitAck();
if(mode){Send_Byte(0x40);}
else{Send_Byte(0x00);}
I2C_WaitAck();
Send_Byte(dat);
I2C_WaitAck();
I2C_Stop();
}
//¿ªÆôOLEDÏÔʾ
void OLED_DisPlay_On(void)
{
OLED_WR_Byte(0x8D,OLED_CMD);//µçºÉ±ÃʹÄÜ
OLED_WR_Byte(0x14,OLED_CMD);//¿ªÆôµçºÉ±Ã
OLED_WR_Byte(0xAF,OLED_CMD);//µãÁÁÆÁÄ»
}
//¹Ø±ÕOLEDÏÔʾ
void OLED_DisPlay_Off(void)
{
OLED_WR_Byte(0x8D,OLED_CMD);//µçºÉ±ÃʹÄÜ
OLED_WR_Byte(0x10,OLED_CMD);//¹Ø±ÕµçºÉ±Ã
OLED_WR_Byte(0xAE,OLED_CMD);//¹Ø±ÕÆÁÄ»
}
//¸üÐÂÏÔ´æµ½OLED
void OLED_Refresh(void)
{
u8 i,n;
for(i=0;i<8;i++)
{
OLED_WR_Byte(0xb0+i,OLED_CMD); //ÉèÖÃÐÐÆðʼµØÖ·
OLED_WR_Byte(0x00,OLED_CMD); //ÉèÖõÍÁÐÆðʼµØÖ·
OLED_WR_Byte(0x10,OLED_CMD); //ÉèÖøßÁÐÆðʼµØÖ·
I2C_Start();
Send_Byte(0x78);
I2C_WaitAck();
Send_Byte(0x40);
I2C_WaitAck();
for(n=0;n<128;n++)
{
Send_Byte(OLED_GRAM[n][i]);
I2C_WaitAck();
}
I2C_Stop();
}
}
//ÇåÆÁº¯Êý
void OLED_Clear(void)
{
u8 i,n;
for(i=0;i<8;i++)
{
for(n=0;n<128;n++)
{
OLED_GRAM[n][i]=0;//Çå³ýËùÓÐÊý¾Ý
}
}
OLED_Refresh();//¸üÐÂÏÔʾ
}
//»µã
//x:0~127
//y:0~63
//t:1 Ìî³ä 0,Çå¿Õ
void OLED_DrawPoint(u8 x,u8 y,u8 t)
{
u8 i,m,n;
i=y/8;
m=y%8;
n=1<<m;
if(t){OLED_GRAM[x][i]|=n;}
else
{
OLED_GRAM[x][i]=~OLED_GRAM[x][i];
OLED_GRAM[x][i]|=n;
OLED_GRAM[x][i]=~OLED_GRAM[x][i];
}
}
//»Ïß
//x1,y1:Æðµã×ø±ê
//x2,y2:½áÊø×ø±ê
void OLED_DrawLine(u8 x1,u8 y1,u8 x2,u8 y2,u8 mode)
{
u16 t;
int xerr=0,yerr=0,delta_x,delta_y,distance;
int incx,incy,uRow,uCol;
delta_x=x2-x1; //¼ÆËã×ø±êÔöÁ¿
delta_y=y2-y1;
uRow=x1;//»Ï߯ðµã×ø±ê
uCol=y1;
if(delta_x>0)incx=1; //ÉèÖõ¥²½·½Ïò
else if (delta_x==0)incx=0;//´¹Ö±Ïß
else {incx=-1;delta_x=-delta_x;}
if(delta_y>0)incy=1;
else if (delta_y==0)incy=0;//ˮƽÏß
else {incy=-1;delta_y=-delta_x;}
if(delta_x>delta_y)distance=delta_x; //ѡȡ»ù±¾ÔöÁ¿×ø±êÖá
else distance=delta_y;
for(t=0;t<distance+1;t++)
{
OLED_DrawPoint(uRow,uCol,mode);//»µã
xerr+=delta_x;
yerr+=delta_y;
if(xerr>distance)
{
xerr-=distance;
uRow+=incx;
}
if(yerr>distance)
{
yerr-=distance;
uCol+=incy;
}
}
}
//x,y:Ô²ÐÄ×ø±ê
//r:Ô²µÄ°ë¾¶
void OLED_DrawCircle(u8 x,u8 y,u8 r)
{
int a, b,num;
a = 0;
b = r;
while(2 * b * b >= r * r)
{
OLED_DrawPoint(x + a, y - b,1);
OLED_DrawPoint(x - a, y - b,1);
OLED_DrawPoint(x - a, y + b,1);
OLED_DrawPoint(x + a, y + b,1);
OLED_DrawPoint(x + b, y + a,1);
OLED_DrawPoint(x + b, y - a,1);
OLED_DrawPoint(x - b, y - a,1);
OLED_DrawPoint(x - b, y + a,1);
a++;
num = (a * a + b * b) - r*r;//¼ÆË㻵ĵãÀëÔ²ÐĵľàÀë
if(num > 0)
{
b--;
a--;
}
}
}
//ÔÚÖ¸¶¨Î»ÖÃÏÔʾһ¸ö×Ö·û,°üÀ¨²¿·Ö×Ö·û
//x:0~127
//y:0~63
//size1:Ñ¡Ôñ×ÖÌå 6x8/6x12/8x16/12x24
//mode:0,·´É«ÏÔʾ;1,Õý³£ÏÔʾ
void OLED_ShowChar(u8 x,u8 y,u8 chr,u8 size1,u8 mode)
{
u8 i,m,temp,size2,chr1;
u8 x0=x,y0=y;
if(size1==8)size2=6;
else size2=(size1/8+((size1%8)?1:0))*(size1/2); //µÃµ½×ÖÌåÒ»¸ö×Ö·û¶ÔÓ¦µãÕó¼¯ËùÕ¼µÄ×Ö½ÚÊý
chr1=chr-' '; //¼ÆËãÆ«ÒÆºóµÄÖµ
for(i=0;i<size2;i++)
{
if(size1==8)
{temp=asc2_0806[chr1][i];} //µ÷ÓÃ0806×ÖÌå
else if(size1==12)
{temp=asc2_1206[chr1][i];} //µ÷ÓÃ1206×ÖÌå
else if(size1==16)
{temp=asc2_1608[chr1][i];} //µ÷ÓÃ1608×ÖÌå
else if(size1==24)
{temp=asc2_2412[chr1][i];} //µ÷ÓÃ2412×ÖÌå
else return;
for(m=0;m<8;m++)
{
if(temp&0x01)OLED_DrawPoint(x,y,mode);
else OLED_DrawPoint(x,y,!mode);
temp>>=1;
y++;
}
x++;
if((size1!=8)&&((x-x0)==size1/2))
{x=x0;y0=y0+8;}
y=y0;
}
}
//ÏÔʾ×Ö·û´®
//x,y:Æðµã×ø±ê
//size1:×ÖÌå´óС
//*chr:×Ö·û´®ÆðʼµØÖ·
//mode:0,·´É«ÏÔʾ;1,Õý³£ÏÔʾ
void OLED_ShowString(u8 x,u8 y,u8 *chr,u8 size1,u8 mode)
{
while((*chr>=' ')&&(*chr<='~'))//ÅжÏÊDz»ÊÇ·Ç·¨×Ö·û!
{
OLED_ShowChar(x,y,*chr,size1,mode);
if(size1==8)x+=6;
else x+=size1/2;
chr++;
}
}
//m^n
u32 OLED_Pow(u8 m,u8 n)
{
u32 result=1;
while(n--)
{
result*=m;
}
return result;
}
//ÏÔʾÊý×Ö
//x,y :Æðµã×ø±ê
//num :ÒªÏÔʾµÄÊý×Ö
//len :Êý×ÖµÄλÊý
//size:×ÖÌå´óС
//mode:0,·´É«ÏÔʾ;1,Õý³£ÏÔʾ
void OLED_ShowNum(u8 x,u8 y,u32 num,u8 len,u8 size1,u8 mode)
{
u8 t,temp,m=0;
if(size1==8)m=2;
for(t=0;t<len;t++)
{
temp=(num/OLED_Pow(10,len-t-1))%10;
if(temp==0)
{
OLED_ShowChar(x+(size1/2+m)*t,y,'0',size1,mode);
}
else
{
OLED_ShowChar(x+(size1/2+m)*t,y,temp+'0',size1,mode);
}
}
}
//ÏÔʾºº×Ö
//x,y:Æðµã×ø±ê
//num:ºº×Ö¶ÔÓ¦µÄÐòºÅ
//mode:0,·´É«ÏÔʾ;1,Õý³£ÏÔʾ
void OLED_ShowChinese(u8 x,u8 y,u8 num,u8 size1,u8 mode)
{
u8 m,temp;
u8 x0=x,y0=y;
u16 i,size3=(size1/8+((size1%8)?1:0))*size1; //µÃµ½×ÖÌåÒ»¸ö×Ö·û¶ÔÓ¦µãÕó¼¯ËùÕ¼µÄ×Ö½ÚÊý
for(i=0;i<size3;i++)
{
if(size1==16)
{temp=Hzk1[num][i];}//µ÷ÓÃ16*16×ÖÌå
else if(size1==24)
{temp=Hzk2[num][i];}//µ÷ÓÃ24*24×ÖÌå
else if(size1==32)
{temp=Hzk3[num][i];}//µ÷ÓÃ32*32×ÖÌå
else if(size1==64)
{temp=Hzk4[num][i];}//µ÷ÓÃ64*64×ÖÌå
else return;
for(m=0;m<8;m++)
{
if(temp&0x01)OLED_DrawPoint(x,y,mode);
else OLED_DrawPoint(x,y,!mode);
temp>>=1;
y++;
}
x++;
if((x-x0)==size1)
{x=x0;y0=y0+8;}
y=y0;
}
}
//num ÏÔʾºº×ֵĸöÊý
//space ÿһ±éÏÔʾµÄ¼ä¸ô
//mode:0,·´É«ÏÔʾ;1,Õý³£ÏÔʾ
void OLED_ScrollDisplay(u8 num,u8 space,u8 mode)
{
u8 i,n,t=0,m=0,r;
while(1)
{
if(m==0)
{
OLED_ShowChinese(128,24,t,16,mode); //дÈëÒ»¸öºº×Ö±£´æÔÚOLED_GRAM[][]Êý×éÖÐ
t++;
}
if(t==num)
{
for(r=0;r<16*space;r++) //ÏÔʾ¼ä¸ô
{
for(i=1;i<144;i++)
{
for(n=0;n<8;n++)
{
OLED_GRAM[i-1][n]=OLED_GRAM[i][n];
}
}
OLED_Refresh();
}
t=0;
}
m++;
if(m==16){m=0;}
for(i=1;i<144;i++) //ʵÏÖ×óÒÆ
{
for(n=0;n<8;n++)
{
OLED_GRAM[i-1][n]=OLED_GRAM[i][n];
}
}
OLED_Refresh();
}
}
//x,y£ºÆðµã×ø±ê
//sizex,sizey,ͼƬ³¤¿í
//BMP[]£ºÒªÐ´ÈëµÄͼƬÊý×é
//mode:0,·´É«ÏÔʾ;1,Õý³£ÏÔʾ
void OLED_ShowPicture(u8 x,u8 y,u8 sizex,u8 sizey,u8 BMP[],u8 mode)
{
u16 j=0;
u8 i,n,temp,m;
u8 x0=x,y0=y;
sizey=sizey/8+((sizey%8)?1:0);
for(n=0;n<sizey;n++)
{
for(i=0;i<sizex;i++)
{
temp=BMP[j];
j++;
for(m=0;m<8;m++)
{
if(temp&0x01)OLED_DrawPoint(x,y,mode);
else OLED_DrawPoint(x,y,!mode);
temp>>=1;
y++;
}
x++;
if((x-x0)==sizex)
{
x=x0;
y0=y0+8;
}
y=y0;
}
}
}
//OLEDµÄ³õʼ»¯
void OLED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); //ʹÄÜB¶Ë¿ÚʱÖÓ
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //ÍÆÍìÊä³ö
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;//ËÙ¶È50MHz
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_SetBits(GPIOB,GPIO_Pin_6);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //ÍÆÍìÊä³ö
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;//ËÙ¶È50MHz
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_SetBits(GPIOB,GPIO_Pin_7);
OLED_WR_Byte(0xAE,OLED_CMD);//--turn off oled panel
OLED_WR_Byte(0x00,OLED_CMD);//---set low column address
OLED_WR_Byte(0x10,OLED_CMD);//---set high column address
OLED_WR_Byte(0x40,OLED_CMD);//--set start line address Set Mapping RAM Display Start Line (0x00~0x3F)
OLED_WR_Byte(0x81,OLED_CMD);//--set contrast control register
OLED_WR_Byte(0xCF,OLED_CMD);// Set SEG Output Current Brightness
OLED_WR_Byte(0xA1,OLED_CMD);//--Set SEG/Column Mapping 0xa0×óÓÒ·´ÖÃ 0xa1Õý³£
OLED_WR_Byte(0xC8,OLED_CMD);//Set COM/Row Scan Direction 0xc0ÉÏÏ·´Öà 0xc8Õý³£
OLED_WR_Byte(0xA6,OLED_CMD);//--set normal display
OLED_WR_Byte(0xA8,OLED_CMD);//--set multiplex ratio(1 to 64)
OLED_WR_Byte(0x3f,OLED_CMD);//--1/64 duty
OLED_WR_Byte(0xD3,OLED_CMD);//-set display offset Shift Mapping RAM Counter (0x00~0x3F)
OLED_WR_Byte(0x00,OLED_CMD);//-not offset
OLED_WR_Byte(0xd5,OLED_CMD);//--set display clock divide ratio/oscillator frequency
OLED_WR_Byte(0x80,OLED_CMD);//--set divide ratio, Set Clock as 100 Frames/Sec
OLED_WR_Byte(0xD9,OLED_CMD);//--set pre-charge period
OLED_WR_Byte(0xF1,OLED_CMD);//Set Pre-Charge as 15 Clocks & Discharge as 1 Clock
OLED_WR_Byte(0xDA,OLED_CMD);//--set com pins hardware configuration
OLED_WR_Byte(0x12,OLED_CMD);
OLED_WR_Byte(0xDB,OLED_CMD);//--set vcomh
OLED_WR_Byte(0x40,OLED_CMD);//Set VCOM Deselect Level
OLED_WR_Byte(0x20,OLED_CMD);//-Set Page Addressing Mode (0x00/0x01/0x02)
OLED_WR_Byte(0x02,OLED_CMD);//
OLED_WR_Byte(0x8D,OLED_CMD);//--set Charge Pump enable/disable
OLED_WR_Byte(0x14,OLED_CMD);//--set(0x10) disable
OLED_WR_Byte(0xA4,OLED_CMD);// Disable Entire Display On (0xa4/0xa5)
OLED_WR_Byte(0xA6,OLED_CMD);// Disable Inverse Display On (0xa6/a7)
OLED_Clear();
OLED_WR_Byte(0xAF,OLED_CMD);
}
void SDA_OUT(void) //????
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_11;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_Out_PP; //????
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void SDA_IN(void) //????
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin= GPIO_Pin_11;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_IPU; //??????
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
oled.h
#ifndef __OLED_H
#define __OLED_H
#include "sys.h"
#include "stdlib.h"
//-----------------LED¶Ë¿Ú¶¨Òå----------------
#define LED_ON GPIO_ResetBits(GPIOB,GPIO_Pin_5)//DC
#define LED_OFF GPIO_SetBits(GPIOB,GPIO_Pin_5)
//-----------------OLED¶Ë¿Ú¶¨Òå----------------
#define Read_IIC_SDA GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_7) //Read_IIC_SDA??PB7??
#define OLED_SCL_Clr() GPIO_ResetBits(GPIOB,GPIO_Pin_6)//D0
#define OLED_SCL_Set() GPIO_SetBits(GPIOB,GPIO_Pin_6)
#define OLED_SDA_Clr() GPIO_ResetBits(GPIOB,GPIO_Pin_7)//D1
#define OLED_SDA_Set() GPIO_SetBits(GPIOB,GPIO_Pin_7)
#define OLED_CMD 0 //дÃüÁî
#define OLED_DATA 1 //дÊý¾Ý
#define OLED_MODE 0
#define OLED_SDA 0
#define OLED_CLK 1
void OLED_RES_Set(void);
void OLED_RES_Clr(void);
void OLED_ClearPoint(u8 x,u8 y);
void OLED_ColorTurn(u8 i);
void OLED_DisplayTurn(u8 i);
void I2C_Start(void);
void I2C_Stop(void);
void I2C_WaitAck(void);
void Send_Byte(u8 dat);
void OLED_WR_Byte(u8 dat,u8 mode);
void OLED_DisPlay_On(void);
void OLED_DisPlay_Off(void);
void OLED_Refresh(void);
void OLED_Clear(void);
void OLED_DrawPoint(u8 x,u8 y,u8 t);
void OLED_DrawLine(u8 x1,u8 y1,u8 x2,u8 y2,u8 mode);
void OLED_DrawCircle(u8 x,u8 y,u8 r);
void OLED_ShowChar(u8 x,u8 y,u8 chr,u8 size1,u8 mode);
void OLED_ShowChar6x8(u8 x,u8 y,u8 chr,u8 mode);
void OLED_ShowString(u8 x,u8 y,u8 *chr,u8 size1,u8 mode);
void OLED_ShowNum(u8 x,u8 y,u32 num,u8 len,u8 size1,u8 mode);
void OLED_ShowChinese(u8 x,u8 y,u8 num,u8 size1,u8 mode);
void OLED_ScrollDisplay(u8 num,u8 space,u8 mode);
void OLED_ShowPicture(u8 x,u8 y,u8 sizex,u8 sizey,u8 BMP[],u8 mode);
void OLED_Init(void);
#endif
sys.h
#ifndef __SYS_H
#define __SYS_H
#include "stm32f10x.h"
//0,²»Ö§³Öucos
//1,Ö§³Öucos
#define SYSTEM_SUPPORT_UCOS 0 //¶¨ÒåϵͳÎļþ¼ÐÊÇ·ñÖ§³ÖUCOS
//λ´ø²Ù×÷,ʵÏÖ51ÀàËÆµÄGPIO¿ØÖƹ¦ÄÜ
//¾ßÌåʵÏÖ˼Ïë,²Î¿¼<<CM3ȨÍþÖ¸ÄÏ>>µÚÎåÕÂ(87Ò³~92Ò³).
//IO¿Ú²Ù×÷ºê¶¨Òå
#define BITBAND(addr, bitnum) ((addr & 0xF0000000)+0x2000000+((addr &0xFFFFF)<<5)+(bitnum<<2))
#define MEM_ADDR(addr) *((volatile unsigned long *)(addr))
#define BIT_ADDR(addr, bitnum) MEM_ADDR(BITBAND(addr, bitnum))
//IO¿ÚµØÖ·Ó³Éä
#define GPIOA_ODR_Addr (GPIOA_BASE+12) //0x4001080C
#define GPIOB_ODR_Addr (GPIOB_BASE+12) //0x40010C0C
#define GPIOC_ODR_Addr (GPIOC_BASE+12) //0x4001100C
#define GPIOD_ODR_Addr (GPIOD_BASE+12) //0x4001140C
#define GPIOE_ODR_Addr (GPIOE_BASE+12) //0x4001180C
#define GPIOF_ODR_Addr (GPIOF_BASE+12) //0x40011A0C
#define GPIOG_ODR_Addr (GPIOG_BASE+12) //0x40011E0C
#define GPIOA_IDR_Addr (GPIOA_BASE+8) //0x40010808
#define GPIOB_IDR_Addr (GPIOB_BASE+8) //0x40010C08
#define GPIOC_IDR_Addr (GPIOC_BASE+8) //0x40011008
#define GPIOD_IDR_Addr (GPIOD_BASE+8) //0x40011408
#define GPIOE_IDR_Addr (GPIOE_BASE+8) //0x40011808
#define GPIOF_IDR_Addr (GPIOF_BASE+8) //0x40011A08
#define GPIOG_IDR_Addr (GPIOG_BASE+8) //0x40011E08
//IO¿Ú²Ù×÷,Ö»¶Ôµ¥Ò»µÄIO¿Ú!
//È·±£nµÄֵСÓÚ16!
#define PAout(n) BIT_ADDR(GPIOA_ODR_Addr,n) //Êä³ö
#define PAin(n) BIT_ADDR(GPIOA_IDR_Addr,n) //ÊäÈë
#define PBout(n) BIT_ADDR(GPIOB_ODR_Addr,n) //Êä³ö
#define PBin(n) BIT_ADDR(GPIOB_IDR_Addr,n) //ÊäÈë
#define PCout(n) BIT_ADDR(GPIOC_ODR_Addr,n) //Êä³ö
#define PCin(n) BIT_ADDR(GPIOC_IDR_Addr,n) //ÊäÈë
#define PDout(n) BIT_ADDR(GPIOD_ODR_Addr,n) //Êä³ö
#define PDin(n) BIT_ADDR(GPIOD_IDR_Addr,n) //ÊäÈë
#define PEout(n) BIT_ADDR(GPIOE_ODR_Addr,n) //Êä³ö
#define PEin(n) BIT_ADDR(GPIOE_IDR_Addr,n) //ÊäÈë
#define PFout(n) BIT_ADDR(GPIOF_ODR_Addr,n) //Êä³ö
#define PFin(n) BIT_ADDR(GPIOF_IDR_Addr,n) //ÊäÈë
#define PGout(n) BIT_ADDR(GPIOG_ODR_Addr,n) //Êä³ö
#define PGin(n) BIT_ADDR(GPIOG_IDR_Addr,n) //ÊäÈë
void NVIC_Configuration(void);
#endif
sys.c
#include "sys.h"
void NVIC_Configuration(void)
{
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //ÉèÖÃNVICÖжϷÖ×é2:2λÇÀÕ¼ÓÅÏȼ¶£¬2λÏìÓ¦ÓÅÏȼ¶
}
oledfont.h
#ifndef __OLEDFONT_H
#define __OLEDFONT_H
const unsigned char asc2_0806[][6] =
{
{0x00, 0x00, 0x00, 0x00, 0x00, 0x00},// sp
{0x00, 0x00, 0x00, 0x2f, 0x00, 0x00},// !
{0x00, 0x00, 0x07, 0x00, 0x07, 0x00},// "
{0x00, 0x14, 0x7f, 0x14, 0x7f, 0x14},// #
{0x00, 0x24, 0x2a, 0x7f, 0x2a, 0x12},// $
{0x00, 0x62, 0x64, 0x08, 0x13, 0x23},// %
{0x00, 0x36, 0x49, 0x55, 0x22, 0x50},// &
{0x00, 0x00, 0x05, 0x03, 0x00, 0x00},// '
{0x00, 0x00, 0x1c, 0x22, 0x41, 0x00},// (
{0x00, 0x00, 0x41, 0x22, 0x1c, 0x00},// )
{0x00, 0x14, 0x08, 0x3E, 0x08, 0x14},// *
{0x00, 0x08, 0x08, 0x3E, 0x08, 0x08},// +
{0x00, 0x00, 0x00, 0xA0, 0x60, 0x00},// ,
{0x00, 0x08, 0x08, 0x08, 0x08, 0x08},// -
{0x00, 0x00, 0x60, 0x60, 0x00, 0x00},// .
{0x00, 0x20, 0x10, 0x08, 0x04, 0x02},// /
{0x00, 0x3E, 0x51, 0x49, 0x45, 0x3E},// 0
{0x00, 0x00, 0x42, 0x7F, 0x40, 0x00},// 1
{0x00, 0x42, 0x61, 0x51, 0x49, 0x46},// 2
{0x00, 0x21, 0x41, 0x45, 0x4B, 0x31},// 3
{0x00, 0x18, 0x14, 0x12, 0x7F, 0x10},// 4
{0x00, 0x27, 0x45, 0x45, 0x45, 0x39},// 5
{0x00, 0x3C, 0x4A, 0x49, 0x49, 0x30},// 6
{0x00, 0x01, 0x71, 0x09, 0x05, 0x03},// 7
{0x00, 0x36, 0x49, 0x49, 0x49, 0x36},// 8
{0x00, 0x06, 0x49, 0x49, 0x29, 0x1E},// 9
{0x00, 0x00, 0x36, 0x36, 0x00, 0x00},// :
{0x00, 0x00, 0x56, 0x36, 0x00, 0x00},// ;
{0x00, 0x08, 0x14, 0x22, 0x41, 0x00},// <
{0x00, 0x14, 0x14, 0x14, 0x14, 0x14},// =
{0x00, 0x00, 0x41, 0x22, 0x14, 0x08},// >
{0x00, 0x02, 0x01, 0x51, 0x09, 0x06},// ?
{0x00, 0x32, 0x49, 0x59, 0x51, 0x3E},// @
{0x00, 0x7C, 0x12, 0x11, 0x12, 0x7C},// A
{0x00, 0x7F, 0x49, 0x49, 0x49, 0x36},// B
{0x00, 0x3E, 0x41, 0x41, 0x41, 0x22},// C
{0x00, 0x7F, 0x41, 0x41, 0x22, 0x1C},// D
{0x00, 0x7F, 0x49, 0x49, 0x49, 0x41},// E
{0x00, 0x7F, 0x09, 0x09, 0x09, 0x01},// F
{0x00, 0x3E, 0x41, 0x49, 0x49, 0x7A},// G
{0x00, 0x7F, 0x08, 0x08, 0x08, 0x7F},// H
{0x00, 0x00, 0x41, 0x7F, 0x41, 0x00},// I
{0x00, 0x20, 0x40, 0x41, 0x3F, 0x01},// J
{0x00, 0x7F, 0x08, 0x14, 0x22, 0x41},// K
{0x00, 0x7F, 0x40, 0x40, 0x40, 0x40},// L
{0x00, 0x7F, 0x02, 0x0C, 0x02, 0x7F},// M
{0x00, 0x7F, 0x04, 0x08, 0x10, 0x7F},// N
{0x00, 0x3E, 0x41, 0x41, 0x41, 0x3E},// O
{0x00, 0x7F, 0x09, 0x09, 0x09, 0x06},// P
{0x00, 0x3E, 0x41, 0x51, 0x21, 0x5E},// Q
{0x00, 0x7F, 0x09, 0x19, 0x29, 0x46},// R
{0x00, 0x46, 0x49, 0x49, 0x49, 0x31},// S
{0x00, 0x01, 0x01, 0x7F, 0x01, 0x01},// T
{0x00, 0x3F, 0x40, 0x40, 0x40, 0x3F},// U
{0x00, 0x1F, 0x20, 0x40, 0x20, 0x1F},// V
{0x00, 0x3F, 0x40, 0x38, 0x40, 0x3F},// W
{0x00, 0x63, 0x14, 0x08, 0x14, 0x63},// X
{0x00, 0x07, 0x08, 0x70, 0x08, 0x07},// Y
{0x00, 0x61, 0x51, 0x49, 0x45, 0x43},// Z
{0x00, 0x00, 0x7F, 0x41, 0x41, 0x00},// [
{0x00, 0x55, 0x2A, 0x55, 0x2A, 0x55},// 55
{0x00, 0x00, 0x41, 0x41, 0x7F, 0x00},// ]
{0x00, 0x04, 0x02, 0x01, 0x02, 0x04},// ^
{0x00, 0x40, 0x40, 0x40, 0x40, 0x40},// _
{0x00, 0x00, 0x01, 0x02, 0x04, 0x00},// '
{0x00, 0x20, 0x54, 0x54, 0x54, 0x78},// a
{0x00, 0x7F, 0x48, 0x44, 0x44, 0x38},// b
{0x00, 0x38, 0x44, 0x44, 0x44, 0x20},// c
{0x00, 0x38, 0x44, 0x44, 0x48, 0x7F},// d
{0x00, 0x38, 0x54, 0x54, 0x54, 0x18},// e
{0x00, 0x08, 0x7E, 0x09, 0x01, 0x02},// f
{0x00, 0x18, 0xA4, 0xA4, 0xA4, 0x7C},// g
{0x00, 0x7F, 0x08, 0x04, 0x04, 0x78},// h
{0x00, 0x00, 0x44, 0x7D, 0x40, 0x00},// i
{0x00, 0x40, 0x80, 0x84, 0x7D, 0x00},// j
{0x00, 0x7F, 0x10, 0x28, 0x44, 0x00},// k
{0x00, 0x00, 0x41, 0x7F, 0x40, 0x00},// l
{0x00, 0x7C, 0x04, 0x18, 0x04, 0x78},// m
{0x00, 0x7C, 0x08, 0x04, 0x04, 0x78},// n
{0x00, 0x38, 0x44, 0x44, 0x44, 0x38},// o
{0x00, 0xFC, 0x24, 0x24, 0x24, 0x18},// p
{0x00, 0x18, 0x24, 0x24, 0x18, 0xFC},// q
{0x00, 0x7C, 0x08, 0x04, 0x04, 0x08},// r
{0x00, 0x48, 0x54, 0x54, 0x54, 0x20},// s
{0x00, 0x04, 0x3F, 0x44, 0x40, 0x20},// t
{0x00, 0x3C, 0x40, 0x40, 0x20, 0x7C},// u
{0x00, 0x1C, 0x20, 0x40, 0x20, 0x1C},// v
{0x00, 0x3C, 0x40, 0x30, 0x40, 0x3C},// w
{0x00, 0x44, 0x28, 0x10, 0x28, 0x44},// x
{0x00, 0x1C, 0xA0, 0xA0, 0xA0, 0x7C},// y
{0x00, 0x44, 0x64, 0x54, 0x4C, 0x44},// z
{0x14, 0x14, 0x14, 0x14, 0x14, 0x14},// horiz lines
};
//12*12 ASCII×Ö·û¼¯µãÕó
const unsigned char asc2_1206[95][12]={
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*" ",0*/
{0x00,0x00,0xFC,0x00,0x00,0x00,0x00,0x00,0x02,0x00,0x00,0x00},/*"!",1*/
{0x00,0x0C,0x02,0x0C,0x02,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*""",2*/
{0x90,0xD0,0xBC,0xD0,0xBC,0x90,0x00,0x03,0x00,0x03,0x00,0x00},/*"#",3*/
{0x18,0x24,0xFE,0x44,0x8C,0x00,0x03,0x02,0x07,0x02,0x01,0x00},/*"$",4*/
{0x18,0x24,0xD8,0xB0,0x4C,0x80,0x00,0x03,0x00,0x01,0x02,0x01},/*"%",5*/
{0xC0,0x38,0xE4,0x38,0xE0,0x00,0x01,0x02,0x02,0x01,0x02,0x02},/*"&",6*/
{0x08,0x06,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"'",7*/
{0x00,0x00,0x00,0xF8,0x04,0x02,0x00,0x00,0x00,0x01,0x02,0x04},/*"(",8*/
{0x00,0x02,0x04,0xF8,0x00,0x00,0x00,0x04,0x02,0x01,0x00,0x00},/*")",9*/
{0x90,0x60,0xF8,0x60,0x90,0x00,0x00,0x00,0x01,0x00,0x00,0x00},/*"*",10*/
{0x20,0x20,0xFC,0x20,0x20,0x00,0x00,0x00,0x01,0x00,0x00,0x00},/*"+",11*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x08,0x06,0x00,0x00,0x00,0x00},/*",",12*/
{0x20,0x20,0x20,0x20,0x20,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"-",13*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x02,0x00,0x00,0x00,0x00},/*".",14*/
{0x00,0x80,0x60,0x1C,0x02,0x00,0x04,0x03,0x00,0x00,0x00,0x00},/*"/",15*/
{0xF8,0x04,0x04,0x04,0xF8,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"0",16*/
{0x00,0x08,0xFC,0x00,0x00,0x00,0x00,0x02,0x03,0x02,0x00,0x00},/*"1",17*/
{0x18,0x84,0x44,0x24,0x18,0x00,0x03,0x02,0x02,0x02,0x02,0x00},/*"2",18*/
{0x08,0x04,0x24,0x24,0xD8,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"3",19*/
{0x40,0xB0,0x88,0xFC,0x80,0x00,0x00,0x00,0x00,0x03,0x02,0x00},/*"4",20*/
{0x3C,0x24,0x24,0x24,0xC4,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"5",21*/
{0xF8,0x24,0x24,0x2C,0xC0,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"6",22*/
{0x0C,0x04,0xE4,0x1C,0x04,0x00,0x00,0x00,0x03,0x00,0x00,0x00},/*"7",23*/
{0xD8,0x24,0x24,0x24,0xD8,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"8",24*/
{0x38,0x44,0x44,0x44,0xF8,0x00,0x00,0x03,0x02,0x02,0x01,0x00},/*"9",25*/
{0x00,0x00,0x10,0x00,0x00,0x00,0x00,0x00,0x02,0x00,0x00,0x00},/*":",26*/
{0x00,0x00,0x20,0x00,0x00,0x00,0x00,0x00,0x06,0x00,0x00,0x00},/*";",27*/
{0x00,0x20,0x50,0x88,0x04,0x02,0x00,0x00,0x00,0x00,0x01,0x02},/*"<",28*/
{0x90,0x90,0x90,0x90,0x90,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"=",29*/
{0x00,0x02,0x04,0x88,0x50,0x20,0x00,0x02,0x01,0x00,0x00,0x00},/*">",30*/
{0x18,0x04,0xC4,0x24,0x18,0x00,0x00,0x00,0x02,0x00,0x00,0x00},/*"?",31*/
{0xF8,0x04,0xE4,0x94,0xF8,0x00,0x01,0x02,0x02,0x02,0x02,0x00},/*"@",32*/
{0x00,0xE0,0x9C,0xF0,0x80,0x00,0x02,0x03,0x00,0x00,0x03,0x02},/*"A",33*/
{0x04,0xFC,0x24,0x24,0xD8,0x00,0x02,0x03,0x02,0x02,0x01,0x00},/*"B",34*/
{0xF8,0x04,0x04,0x04,0x0C,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"C",35*/
{0x04,0xFC,0x04,0x04,0xF8,0x00,0x02,0x03,0x02,0x02,0x01,0x00},/*"D",36*/
{0x04,0xFC,0x24,0x74,0x0C,0x00,0x02,0x03,0x02,0x02,0x03,0x00},/*"E",37*/
{0x04,0xFC,0x24,0x74,0x0C,0x00,0x02,0x03,0x02,0x00,0x00,0x00},/*"F",38*/
{0xF0,0x08,0x04,0x44,0xCC,0x40,0x00,0x01,0x02,0x02,0x01,0x00},/*"G",39*/
{0x04,0xFC,0x20,0x20,0xFC,0x04,0x02,0x03,0x00,0x00,0x03,0x02},/*"H",40*/
{0x04,0x04,0xFC,0x04,0x04,0x00,0x02,0x02,0x03,0x02,0x02,0x00},/*"I",41*/
{0x00,0x04,0x04,0xFC,0x04,0x04,0x06,0x04,0x04,0x03,0x00,0x00},/*"J",42*/
{0x04,0xFC,0x24,0xD0,0x0C,0x04,0x02,0x03,0x02,0x00,0x03,0x02},/*"K",43*/
{0x04,0xFC,0x04,0x00,0x00,0x00,0x02,0x03,0x02,0x02,0x02,0x03},/*"L",44*/
{0xFC,0x3C,0xC0,0x3C,0xFC,0x00,0x03,0x00,0x03,0x00,0x03,0x00},/*"M",45*/
{0x04,0xFC,0x30,0xC4,0xFC,0x04,0x02,0x03,0x02,0x00,0x03,0x00},/*"N",46*/
{0xF8,0x04,0x04,0x04,0xF8,0x00,0x01,0x02,0x02,0x02,0x01,0x00},/*"O",47*/
{0x04,0xFC,0x24,0x24,0x18,0x00,0x02,0x03,0x02,0x00,0x00,0x00},/*"P",48*/
{0xF8,0x84,0x84,0x04,0xF8,0x00,0x01,0x02,0x02,0x07,0x05,0x00},/*"Q",49*/
{0x04,0xFC,0x24,0x64,0x98,0x00,0x02,0x03,0x02,0x00,0x03,0x02},/*"R",50*/
{0x18,0x24,0x24,0x44,0x8C,0x00,0x03,0x02,0x02,0x02,0x01,0x00},/*"S",51*/
{0x0C,0x04,0xFC,0x04,0x0C,0x00,0x00,0x02,0x03,0x02,0x00,0x00},/*"T",52*/
{0x04,0xFC,0x00,0x00,0xFC,0x04,0x00,0x01,0x02,0x02,0x01,0x00},/*"U",53*/
{0x04,0x7C,0x80,0xE0,0x1C,0x04,0x00,0x00,0x03,0x00,0x00,0x00},/*"V",54*/
{0x1C,0xE0,0x3C,0xE0,0x1C,0x00,0x00,0x03,0x00,0x03,0x00,0x00},/*"W",55*/
{0x04,0x9C,0x60,0x9C,0x04,0x00,0x02,0x03,0x00,0x03,0x02,0x00},/*"X",56*/
{0x04,0x1C,0xE0,0x1C,0x04,0x00,0x00,0x02,0x03,0x02,0x00,0x00},/*"Y",57*/
{0x0C,0x84,0x64,0x1C,0x04,0x00,0x02,0x03,0x02,0x02,0x03,0x00},/*"Z",58*/
{0x00,0x00,0xFE,0x02,0x02,0x00,0x00,0x00,0x07,0x04,0x04,0x00},/*"[",59*/
{0x00,0x0E,0x30,0xC0,0x00,0x00,0x00,0x00,0x00,0x01,0x02,0x00},/*"\",60*/
{0x00,0x02,0x02,0xFE,0x00,0x00,0x00,0x04,0x04,0x07,0x00,0x00},/*"]",61*/
{0x00,0x04,0x02,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"^",62*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x08,0x08,0x08,0x08,0x08,0x08},/*"_",63*/
{0x00,0x00,0x02,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"`",64*/
{0x00,0x40,0xA0,0xA0,0xC0,0x00,0x00,0x01,0x02,0x02,0x03,0x02},/*"a",65*/
{0x04,0xFC,0x20,0x20,0xC0,0x00,0x00,0x03,0x02,0x02,0x01,0x00},/*"b",66*/
{0x00,0xC0,0x20,0x20,0x60,0x00,0x00,0x01,0x02,0x02,0x02,0x00},/*"c",67*/
{0x00,0xC0,0x20,0x24,0xFC,0x00,0x00,0x01,0x02,0x02,0x03,0x02},/*"d",68*/
{0x00,0xC0,0xA0,0xA0,0xC0,0x00,0x00,0x01,0x02,0x02,0x02,0x00},/*"e",69*/
{0x00,0x20,0xF8,0x24,0x24,0x04,0x00,0x02,0x03,0x02,0x02,0x00},/*"f",70*/
{0x00,0x40,0xA0,0xA0,0x60,0x20,0x00,0x07,0x0A,0x0A,0x0A,0x04},/*"g",71*/
{0x04,0xFC,0x20,0x20,0xC0,0x00,0x02,0x03,0x02,0x00,0x03,0x02},/*"h",72*/
{0x00,0x20,0xE4,0x00,0x00,0x00,0x00,0x02,0x03,0x02,0x00,0x00},/*"i",73*/
{0x00,0x00,0x20,0xE4,0x00,0x00,0x08,0x08,0x08,0x07,0x00,0x00},/*"j",74*/
{0x04,0xFC,0x80,0xE0,0x20,0x20,0x02,0x03,0x02,0x00,0x03,0x02},/*"k",75*/
{0x04,0x04,0xFC,0x00,0x00,0x00,0x02,0x02,0x03,0x02,0x02,0x00},/*"l",76*/
{0xE0,0x20,0xE0,0x20,0xC0,0x00,0x03,0x00,0x03,0x00,0x03,0x00},/*"m",77*/
{0x20,0xE0,0x20,0x20,0xC0,0x00,0x02,0x03,0x02,0x00,0x03,0x02},/*"n",78*/
{0x00,0xC0,0x20,0x20,0xC0,0x00,0x00,0x01,0x02,0x02,0x01,0x00},/*"o",79*/
{0x20,0xE0,0x20,0x20,0xC0,0x00,0x08,0x0F,0x0A,0x02,0x01,0x00},/*"p",80*/
{0x00,0xC0,0x20,0x20,0xE0,0x00,0x00,0x01,0x02,0x0A,0x0F,0x08},/*"q",81*/
{0x20,0xE0,0x40,0x20,0x20,0x00,0x02,0x03,0x02,0x00,0x00,0x00},/*"r",82*/
{0x00,0x60,0xA0,0xA0,0x20,0x00,0x00,0x02,0x02,0x02,0x03,0x00},/*"s",83*/
{0x00,0x20,0xF8,0x20,0x00,0x00,0x00,0x00,0x01,0x02,0x02,0x00},/*"t",84*/
{0x20,0xE0,0x00,0x20,0xE0,0x00,0x00,0x01,0x02,0x02,0x03,0x02},/*"u",85*/
{0x20,0xE0,0x20,0x80,0x60,0x20,0x00,0x00,0x03,0x01,0x00,0x00},/*"v",86*/
{0x60,0x80,0xE0,0x80,0x60,0x00,0x00,0x03,0x00,0x03,0x00,0x00},/*"w",87*/
{0x20,0x60,0x80,0x60,0x20,0x00,0x02,0x03,0x00,0x03,0x02,0x00},/*"x",88*/
{0x20,0xE0,0x20,0x80,0x60,0x20,0x08,0x08,0x07,0x01,0x00,0x00},/*"y",89*/
{0x00,0x20,0xA0,0x60,0x20,0x00,0x00,0x02,0x03,0x02,0x02,0x00},/*"z",90*/
{0x00,0x00,0x20,0xDE,0x02,0x00,0x00,0x00,0x00,0x07,0x04,0x00},/*"{",91*/
{0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00,0x0F,0x00,0x00},/*"|",92*/
{0x00,0x02,0xDE,0x20,0x00,0x00,0x00,0x04,0x07,0x00,0x00,0x00},/*"}",93*/
{0x02,0x01,0x02,0x04,0x04,0x02,0x00,0x00,0x00,0x00,0x00,0x00},/*"~",94*/
};
//16*16 ASCII×Ö·û¼¯µãÕó
const unsigned char asc2_1608[][16]={
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*" ",0*/
{0x00,0x00,0x00,0xF8,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x33,0x30,0x00,0x00,0x00},/*"!",1*/
{0x00,0x10,0x0C,0x06,0x10,0x0C,0x06,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*""",2*/
{0x40,0xC0,0x78,0x40,0xC0,0x78,0x40,0x00,0x04,0x3F,0x04,0x04,0x3F,0x04,0x04,0x00},/*"#",3*/
{0x00,0x70,0x88,0xFC,0x08,0x30,0x00,0x00,0x00,0x18,0x20,0xFF,0x21,0x1E,0x00,0x00},/*"$",4*/
{0xF0,0x08,0xF0,0x00,0xE0,0x18,0x00,0x00,0x00,0x21,0x1C,0x03,0x1E,0x21,0x1E,0x00},/*"%",5*/
{0x00,0xF0,0x08,0x88,0x70,0x00,0x00,0x00,0x1E,0x21,0x23,0x24,0x19,0x27,0x21,0x10},/*"&",6*/
{0x10,0x16,0x0E,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"'",7*/
{0x00,0x00,0x00,0xE0,0x18,0x04,0x02,0x00,0x00,0x00,0x00,0x07,0x18,0x20,0x40,0x00},/*"(",8*/
{0x00,0x02,0x04,0x18,0xE0,0x00,0x00,0x00,0x00,0x40,0x20,0x18,0x07,0x00,0x00,0x00},/*")",9*/
{0x40,0x40,0x80,0xF0,0x80,0x40,0x40,0x00,0x02,0x02,0x01,0x0F,0x01,0x02,0x02,0x00},/*"*",10*/
{0x00,0x00,0x00,0xF0,0x00,0x00,0x00,0x00,0x01,0x01,0x01,0x1F,0x01,0x01,0x01,0x00},/*"+",11*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0xB0,0x70,0x00,0x00,0x00,0x00,0x00},/*",",12*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x01,0x01,0x01,0x01,0x01,0x01},/*"-",13*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x30,0x30,0x00,0x00,0x00,0x00,0x00},/*".",14*/
{0x00,0x00,0x00,0x00,0x80,0x60,0x18,0x04,0x00,0x60,0x18,0x06,0x01,0x00,0x00,0x00},/*"/",15*/
{0x00,0xE0,0x10,0x08,0x08,0x10,0xE0,0x00,0x00,0x0F,0x10,0x20,0x20,0x10,0x0F,0x00},/*"0",16*/
{0x00,0x10,0x10,0xF8,0x00,0x00,0x00,0x00,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00},/*"1",17*/
{0x00,0x70,0x08,0x08,0x08,0x88,0x70,0x00,0x00,0x30,0x28,0x24,0x22,0x21,0x30,0x00},/*"2",18*/
{0x00,0x30,0x08,0x88,0x88,0x48,0x30,0x00,0x00,0x18,0x20,0x20,0x20,0x11,0x0E,0x00},/*"3",19*/
{0x00,0x00,0xC0,0x20,0x10,0xF8,0x00,0x00,0x00,0x07,0x04,0x24,0x24,0x3F,0x24,0x00},/*"4",20*/
{0x00,0xF8,0x08,0x88,0x88,0x08,0x08,0x00,0x00,0x19,0x21,0x20,0x20,0x11,0x0E,0x00},/*"5",21*/
{0x00,0xE0,0x10,0x88,0x88,0x18,0x00,0x00,0x00,0x0F,0x11,0x20,0x20,0x11,0x0E,0x00},/*"6",22*/
{0x00,0x38,0x08,0x08,0xC8,0x38,0x08,0x00,0x00,0x00,0x00,0x3F,0x00,0x00,0x00,0x00},/*"7",23*/
{0x00,0x70,0x88,0x08,0x08,0x88,0x70,0x00,0x00,0x1C,0x22,0x21,0x21,0x22,0x1C,0x00},/*"8",24*/
{0x00,0xE0,0x10,0x08,0x08,0x10,0xE0,0x00,0x00,0x00,0x31,0x22,0x22,0x11,0x0F,0x00},/*"9",25*/
{0x00,0x00,0x00,0xC0,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0x30,0x30,0x00,0x00,0x00},/*":",26*/
{0x00,0x00,0x00,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x60,0x00,0x00,0x00,0x00},/*";",27*/
{0x00,0x00,0x80,0x40,0x20,0x10,0x08,0x00,0x00,0x01,0x02,0x04,0x08,0x10,0x20,0x00},/*"<",28*/
{0x40,0x40,0x40,0x40,0x40,0x40,0x40,0x00,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0x00},/*"=",29*/
{0x00,0x08,0x10,0x20,0x40,0x80,0x00,0x00,0x00,0x20,0x10,0x08,0x04,0x02,0x01,0x00},/*">",30*/
{0x00,0x70,0x48,0x08,0x08,0x08,0xF0,0x00,0x00,0x00,0x00,0x30,0x36,0x01,0x00,0x00},/*"?",31*/
{0xC0,0x30,0xC8,0x28,0xE8,0x10,0xE0,0x00,0x07,0x18,0x27,0x24,0x23,0x14,0x0B,0x00},/*"@",32*/
{0x00,0x00,0xC0,0x38,0xE0,0x00,0x00,0x00,0x20,0x3C,0x23,0x02,0x02,0x27,0x38,0x20},/*"A",33*/
{0x08,0xF8,0x88,0x88,0x88,0x70,0x00,0x00,0x20,0x3F,0x20,0x20,0x20,0x11,0x0E,0x00},/*"B",34*/
{0xC0,0x30,0x08,0x08,0x08,0x08,0x38,0x00,0x07,0x18,0x20,0x20,0x20,0x10,0x08,0x00},/*"C",35*/
{0x08,0xF8,0x08,0x08,0x08,0x10,0xE0,0x00,0x20,0x3F,0x20,0x20,0x20,0x10,0x0F,0x00},/*"D",36*/
{0x08,0xF8,0x88,0x88,0xE8,0x08,0x10,0x00,0x20,0x3F,0x20,0x20,0x23,0x20,0x18,0x00},/*"E",37*/
{0x08,0xF8,0x88,0x88,0xE8,0x08,0x10,0x00,0x20,0x3F,0x20,0x00,0x03,0x00,0x00,0x00},/*"F",38*/
{0xC0,0x30,0x08,0x08,0x08,0x38,0x00,0x00,0x07,0x18,0x20,0x20,0x22,0x1E,0x02,0x00},/*"G",39*/
{0x08,0xF8,0x08,0x00,0x00,0x08,0xF8,0x08,0x20,0x3F,0x21,0x01,0x01,0x21,0x3F,0x20},/*"H",40*/
{0x00,0x08,0x08,0xF8,0x08,0x08,0x00,0x00,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00},/*"I",41*/
{0x00,0x00,0x08,0x08,0xF8,0x08,0x08,0x00,0xC0,0x80,0x80,0x80,0x7F,0x00,0x00,0x00},/*"J",42*/
{0x08,0xF8,0x88,0xC0,0x28,0x18,0x08,0x00,0x20,0x3F,0x20,0x01,0x26,0x38,0x20,0x00},/*"K",43*/
{0x08,0xF8,0x08,0x00,0x00,0x00,0x00,0x00,0x20,0x3F,0x20,0x20,0x20,0x20,0x30,0x00},/*"L",44*/
{0x08,0xF8,0xF8,0x00,0xF8,0xF8,0x08,0x00,0x20,0x3F,0x00,0x3F,0x00,0x3F,0x20,0x00},/*"M",45*/
{0x08,0xF8,0x30,0xC0,0x00,0x08,0xF8,0x08,0x20,0x3F,0x20,0x00,0x07,0x18,0x3F,0x00},/*"N",46*/
{0xE0,0x10,0x08,0x08,0x08,0x10,0xE0,0x00,0x0F,0x10,0x20,0x20,0x20,0x10,0x0F,0x00},/*"O",47*/
{0x08,0xF8,0x08,0x08,0x08,0x08,0xF0,0x00,0x20,0x3F,0x21,0x01,0x01,0x01,0x00,0x00},/*"P",48*/
{0xE0,0x10,0x08,0x08,0x08,0x10,0xE0,0x00,0x0F,0x18,0x24,0x24,0x38,0x50,0x4F,0x00},/*"Q",49*/
{0x08,0xF8,0x88,0x88,0x88,0x88,0x70,0x00,0x20,0x3F,0x20,0x00,0x03,0x0C,0x30,0x20},/*"R",50*/
{0x00,0x70,0x88,0x08,0x08,0x08,0x38,0x00,0x00,0x38,0x20,0x21,0x21,0x22,0x1C,0x00},/*"S",51*/
{0x18,0x08,0x08,0xF8,0x08,0x08,0x18,0x00,0x00,0x00,0x20,0x3F,0x20,0x00,0x00,0x00},/*"T",52*/
{0x08,0xF8,0x08,0x00,0x00,0x08,0xF8,0x08,0x00,0x1F,0x20,0x20,0x20,0x20,0x1F,0x00},/*"U",53*/
{0x08,0x78,0x88,0x00,0x00,0xC8,0x38,0x08,0x00,0x00,0x07,0x38,0x0E,0x01,0x00,0x00},/*"V",54*/
{0xF8,0x08,0x00,0xF8,0x00,0x08,0xF8,0x00,0x03,0x3C,0x07,0x00,0x07,0x3C,0x03,0x00},/*"W",55*/
{0x08,0x18,0x68,0x80,0x80,0x68,0x18,0x08,0x20,0x30,0x2C,0x03,0x03,0x2C,0x30,0x20},/*"X",56*/
{0x08,0x38,0xC8,0x00,0xC8,0x38,0x08,0x00,0x00,0x00,0x20,0x3F,0x20,0x00,0x00,0x00},/*"Y",57*/
{0x10,0x08,0x08,0x08,0xC8,0x38,0x08,0x00,0x20,0x38,0x26,0x21,0x20,0x20,0x18,0x00},/*"Z",58*/
{0x00,0x00,0x00,0xFE,0x02,0x02,0x02,0x00,0x00,0x00,0x00,0x7F,0x40,0x40,0x40,0x00},/*"[",59*/
{0x00,0x0C,0x30,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x06,0x38,0xC0,0x00},/*"\",60*/
{0x00,0x02,0x02,0x02,0xFE,0x00,0x00,0x00,0x00,0x40,0x40,0x40,0x7F,0x00,0x00,0x00},/*"]",61*/
{0x00,0x00,0x04,0x02,0x02,0x02,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"^",62*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x80},/*"_",63*/
{0x00,0x02,0x02,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"`",64*/
{0x00,0x00,0x80,0x80,0x80,0x80,0x00,0x00,0x00,0x19,0x24,0x22,0x22,0x22,0x3F,0x20},/*"a",65*/
{0x08,0xF8,0x00,0x80,0x80,0x00,0x00,0x00,0x00,0x3F,0x11,0x20,0x20,0x11,0x0E,0x00},/*"b",66*/
{0x00,0x00,0x00,0x80,0x80,0x80,0x00,0x00,0x00,0x0E,0x11,0x20,0x20,0x20,0x11,0x00},/*"c",67*/
{0x00,0x00,0x00,0x80,0x80,0x88,0xF8,0x00,0x00,0x0E,0x11,0x20,0x20,0x10,0x3F,0x20},/*"d",68*/
{0x00,0x00,0x80,0x80,0x80,0x80,0x00,0x00,0x00,0x1F,0x22,0x22,0x22,0x22,0x13,0x00},/*"e",69*/
{0x00,0x80,0x80,0xF0,0x88,0x88,0x88,0x18,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00},/*"f",70*/
{0x00,0x00,0x80,0x80,0x80,0x80,0x80,0x00,0x00,0x6B,0x94,0x94,0x94,0x93,0x60,0x00},/*"g",71*/
{0x08,0xF8,0x00,0x80,0x80,0x80,0x00,0x00,0x20,0x3F,0x21,0x00,0x00,0x20,0x3F,0x20},/*"h",72*/
{0x00,0x80,0x98,0x98,0x00,0x00,0x00,0x00,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00},/*"i",73*/
{0x00,0x00,0x00,0x80,0x98,0x98,0x00,0x00,0x00,0xC0,0x80,0x80,0x80,0x7F,0x00,0x00},/*"j",74*/
{0x08,0xF8,0x00,0x00,0x80,0x80,0x80,0x00,0x20,0x3F,0x24,0x02,0x2D,0x30,0x20,0x00},/*"k",75*/
{0x00,0x08,0x08,0xF8,0x00,0x00,0x00,0x00,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00},/*"l",76*/
{0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x00,0x20,0x3F,0x20,0x00,0x3F,0x20,0x00,0x3F},/*"m",77*/
{0x80,0x80,0x00,0x80,0x80,0x80,0x00,0x00,0x20,0x3F,0x21,0x00,0x00,0x20,0x3F,0x20},/*"n",78*/
{0x00,0x00,0x80,0x80,0x80,0x80,0x00,0x00,0x00,0x1F,0x20,0x20,0x20,0x20,0x1F,0x00},/*"o",79*/
{0x80,0x80,0x00,0x80,0x80,0x00,0x00,0x00,0x80,0xFF,0xA1,0x20,0x20,0x11,0x0E,0x00},/*"p",80*/
{0x00,0x00,0x00,0x80,0x80,0x80,0x80,0x00,0x00,0x0E,0x11,0x20,0x20,0xA0,0xFF,0x80},/*"q",81*/
{0x80,0x80,0x80,0x00,0x80,0x80,0x80,0x00,0x20,0x20,0x3F,0x21,0x20,0x00,0x01,0x00},/*"r",82*/
{0x00,0x00,0x80,0x80,0x80,0x80,0x80,0x00,0x00,0x33,0x24,0x24,0x24,0x24,0x19,0x00},/*"s",83*/
{0x00,0x80,0x80,0xE0,0x80,0x80,0x00,0x00,0x00,0x00,0x00,0x1F,0x20,0x20,0x00,0x00},/*"t",84*/
{0x80,0x80,0x00,0x00,0x00,0x80,0x80,0x00,0x00,0x1F,0x20,0x20,0x20,0x10,0x3F,0x20},/*"u",85*/
{0x80,0x80,0x80,0x00,0x00,0x80,0x80,0x80,0x00,0x01,0x0E,0x30,0x08,0x06,0x01,0x00},/*"v",86*/
{0x80,0x80,0x00,0x80,0x00,0x80,0x80,0x80,0x0F,0x30,0x0C,0x03,0x0C,0x30,0x0F,0x00},/*"w",87*/
{0x00,0x80,0x80,0x00,0x80,0x80,0x80,0x00,0x00,0x20,0x31,0x2E,0x0E,0x31,0x20,0x00},/*"x",88*/
{0x80,0x80,0x80,0x00,0x00,0x80,0x80,0x80,0x80,0x81,0x8E,0x70,0x18,0x06,0x01,0x00},/*"y",89*/
{0x00,0x80,0x80,0x80,0x80,0x80,0x80,0x00,0x00,0x21,0x30,0x2C,0x22,0x21,0x30,0x00},/*"z",90*/
{0x00,0x00,0x00,0x00,0x80,0x7C,0x02,0x02,0x00,0x00,0x00,0x00,0x00,0x3F,0x40,0x40},/*"{",91*/
{0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00},/*"|",92*/
{0x00,0x02,0x02,0x7C,0x80,0x00,0x00,0x00,0x00,0x40,0x40,0x3F,0x00,0x00,0x00,0x00},/*"}",93*/
{0x00,0x06,0x01,0x01,0x02,0x02,0x04,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"~",94*/
};
//24*24 ASICII×Ö·û¼¯µãÕó
const unsigned char asc2_2412[][36]={
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*" ",0*/
{0x00,0x00,0x00,0x00,0x00,0xF0,0xF0,0xF0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x7F,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1C,0x1C,0x1C,0x00,0x00,0x00,0x00},/*"!",1*/
{0x00,0x00,0x80,0x60,0x30,0x1C,0x8C,0x60,0x30,0x1C,0x0C,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*""",2*/
{0x00,0x00,0x00,0xE0,0x00,0x00,0x00,0x00,0x00,0xE0,0x00,0x00,0x00,0x86,0xE6,0x9F,0x86,0x86,0x86,0x86,0xE6,0x9F,0x86,0x00,0x00,0x01,0x1F,0x01,0x01,0x01,0x01,0x01,0x1F,0x01,0x01,0x00},/*"#",3*/
{0x00,0x00,0x80,0xC0,0x60,0x20,0xF8,0x20,0xE0,0xC0,0x00,0x00,0x00,0x00,0x03,0x07,0x0C,0x18,0xFF,0x70,0xE1,0x81,0x00,0x00,0x00,0x00,0x07,0x0F,0x10,0x10,0x7F,0x10,0x0F,0x07,0x00,0x00},/*"$",4*/
{0x80,0x60,0x20,0x60,0x80,0x00,0x00,0x00,0xE0,0x20,0x00,0x00,0x0F,0x30,0x20,0x30,0x9F,0x70,0xDC,0x37,0x10,0x30,0xC0,0x00,0x00,0x00,0x10,0x0E,0x03,0x00,0x07,0x18,0x10,0x18,0x07,0x00},/*"%",5*/
{0x00,0x00,0xC0,0x20,0x20,0xE0,0xC0,0x00,0x00,0x00,0x00,0x00,0x80,0xE0,0x1F,0x38,0xE8,0x87,0x03,0xC4,0x3C,0x04,0x00,0x00,0x07,0x0F,0x18,0x10,0x10,0x0B,0x07,0x0D,0x10,0x10,0x08,0x00},/*"&",6*/
{0x00,0x80,0x8C,0x4C,0x38,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"'",7*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x80,0xE0,0x30,0x08,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0xFE,0xFF,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x03,0x0F,0x18,0x20,0x40,0x00},/*"(",8*/
{0x00,0x04,0x08,0x30,0xE0,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0xFF,0xFE,0x00,0x00,0x00,0x00,0x00,0x00,0x40,0x20,0x18,0x0F,0x03,0x00,0x00,0x00,0x00,0x00,0x00},/*")",9*/
{0x00,0x00,0x00,0x00,0x00,0x00,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0x42,0x66,0x66,0x3C,0x18,0xFF,0x18,0x3C,0x66,0x66,0x42,0x00,0x00,0x00,0x00,0x00,0x00,0x03,0x00,0x00,0x00,0x00,0x00},/*"*",10*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x10,0x10,0x10,0xFF,0x10,0x10,0x10,0x10,0x10,0x00,0x00,0x00,0x00,0x00,0x00,0x03,0x00,0x00,0x00,0x00,0x00},/*"+",11*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x8C,0x4C,0x38,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*",",12*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x10,0x10,0x10,0x10,0x10,0x10,0x10,0x10,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"-",13*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1C,0x1C,0x1C,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*".",14*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xE0,0x38,0x0C,0x00,0x00,0x00,0x00,0x00,0x80,0x70,0x1C,0x03,0x00,0x00,0x00,0x00,0x00,0x60,0x38,0x0E,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"/",15*/
{0x00,0x00,0x80,0xC0,0x60,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x00,0xFE,0xFF,0x01,0x00,0x00,0x00,0x00,0x01,0xFF,0xFE,0x00,0x00,0x01,0x07,0x0E,0x18,0x10,0x10,0x18,0x0E,0x07,0x01,0x00},/*"0",16*/
{0x00,0x00,0x80,0x80,0x80,0xC0,0xE0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x10,0x1F,0x1F,0x10,0x10,0x10,0x00,0x00},/*"1",17*/
{0x00,0x80,0x40,0x20,0x20,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x00,0x03,0x03,0x00,0x80,0x40,0x20,0x38,0x1F,0x07,0x00,0x00,0x00,0x1C,0x1A,0x19,0x18,0x18,0x18,0x18,0x18,0x1F,0x00,0x00},/*"2",18*/
{0x00,0x80,0xC0,0x20,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x00,0x00,0x03,0x03,0x00,0x10,0x10,0x18,0x2F,0xE7,0x80,0x00,0x00,0x00,0x07,0x0F,0x10,0x10,0x10,0x10,0x18,0x0F,0x07,0x00,0x00},/*"3",19*/
{0x00,0x00,0x00,0x00,0x00,0x00,0xC0,0xE0,0xF0,0x00,0x00,0x00,0x00,0xC0,0xB0,0x88,0x86,0x81,0x80,0xFF,0xFF,0x80,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x1F,0x1F,0x10,0x10,0x00},/*"4",20*/
{0x00,0x00,0xE0,0x60,0x60,0x60,0x60,0x60,0x60,0x60,0x00,0x00,0x00,0x00,0x3F,0x10,0x08,0x08,0x08,0x18,0xF0,0xE0,0x00,0x00,0x00,0x07,0x0B,0x10,0x10,0x10,0x10,0x1C,0x0F,0x03,0x00,0x00},/*"5",21*/
{0x00,0x00,0x80,0xC0,0x40,0x20,0x20,0x20,0xE0,0xC0,0x00,0x00,0x00,0xFC,0xFF,0x21,0x10,0x08,0x08,0x08,0x18,0xF0,0xE0,0x00,0x00,0x01,0x07,0x0C,0x18,0x10,0x10,0x10,0x08,0x0F,0x03,0x00},/*"6",22*/
{0x00,0x00,0xC0,0xE0,0x60,0x60,0x60,0x60,0x60,0xE0,0x60,0x00,0x00,0x00,0x03,0x00,0x00,0x00,0xE0,0x18,0x07,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1F,0x1F,0x00,0x00,0x00,0x00,0x00},/*"7",23*/
{0x00,0x80,0xC0,0x60,0x20,0x20,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x87,0xEF,0x2C,0x18,0x18,0x30,0x30,0x68,0xCF,0x83,0x00,0x00,0x07,0x0F,0x08,0x10,0x10,0x10,0x10,0x18,0x0F,0x07,0x00},/*"8",24*/
{0x00,0x00,0xC0,0xC0,0x20,0x20,0x20,0x20,0xC0,0x80,0x00,0x00,0x00,0x1F,0x3F,0x60,0x40,0x40,0x40,0x20,0x10,0xFF,0xFE,0x00,0x00,0x00,0x0C,0x1C,0x10,0x10,0x10,0x08,0x0F,0x03,0x00,0x00},/*"9",25*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x0E,0x0E,0x0E,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1C,0x1C,0x1C,0x00,0x00,0x00,0x00},/*":",26*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x0C,0x0C,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x58,0x38,0x00,0x00,0x00,0x00,0x00},/*";",27*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x40,0x20,0x10,0x00,0x00,0x00,0x10,0x28,0x44,0x82,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x02,0x04,0x08,0x10,0x00},/*"<",28*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x84,0x84,0x84,0x84,0x84,0x84,0x84,0x84,0x84,0x84,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"=",29*/
{0x00,0x00,0x10,0x20,0x40,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x82,0x44,0x28,0x10,0x00,0x00,0x00,0x10,0x08,0x04,0x02,0x01,0x00,0x00,0x00,0x00,0x00},/*">",30*/
{0x00,0xC0,0x20,0x20,0x10,0x10,0x10,0x10,0x30,0xE0,0xC0,0x00,0x00,0x03,0x03,0x00,0x00,0xF0,0x10,0x08,0x0C,0x07,0x03,0x00,0x00,0x00,0x00,0x00,0x1C,0x1C,0x1C,0x00,0x00,0x00,0x00,0x00},/*"?",31*/
{0x00,0x00,0x00,0xC0,0x40,0x60,0x20,0x20,0x20,0x40,0xC0,0x00,0x00,0xFC,0xFF,0x01,0xF0,0x0E,0x03,0xC1,0xFE,0x03,0x80,0x7F,0x00,0x01,0x07,0x0E,0x08,0x11,0x11,0x10,0x11,0x09,0x04,0x02},/*"@",32*/
{0x00,0x00,0x00,0x00,0x80,0xE0,0xE0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x7C,0x43,0x40,0x47,0x7F,0xF8,0x80,0x00,0x00,0x10,0x18,0x1F,0x10,0x00,0x00,0x00,0x00,0x13,0x1F,0x1C,0x10},/*"A",33*/
{0x20,0xE0,0xE0,0x20,0x20,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x00,0xFF,0xFF,0x10,0x10,0x10,0x10,0x18,0x2F,0xE7,0x80,0x00,0x10,0x1F,0x1F,0x10,0x10,0x10,0x10,0x10,0x18,0x0F,0x07,0x00},/*"B",34*/
{0x00,0x00,0x80,0xC0,0x40,0x20,0x20,0x20,0x20,0x60,0xE0,0x00,0x00,0xFC,0xFF,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x00,0x00,0x01,0x07,0x0E,0x18,0x10,0x10,0x10,0x08,0x04,0x03,0x00},/*"C",35*/
{0x20,0xE0,0xE0,0x20,0x20,0x20,0x20,0x40,0xC0,0x80,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x01,0xFF,0xFE,0x00,0x10,0x1F,0x1F,0x10,0x10,0x10,0x18,0x08,0x0E,0x07,0x01,0x00},/*"D",36*/
{0x20,0xE0,0xE0,0x20,0x20,0x20,0x20,0x20,0x20,0x60,0x80,0x00,0x00,0xFF,0xFF,0x10,0x10,0x10,0x10,0x7C,0x00,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10,0x10,0x10,0x10,0x10,0x10,0x18,0x06,0x00},/*"E",37*/
{0x20,0xE0,0xE0,0x20,0x20,0x20,0x20,0x20,0x60,0x60,0x80,0x00,0x00,0xFF,0xFF,0x10,0x10,0x10,0x10,0x7C,0x00,0x00,0x01,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"F",38*/
{0x00,0x00,0x80,0xC0,0x60,0x20,0x20,0x20,0x40,0xE0,0x00,0x00,0x00,0xFC,0xFF,0x01,0x00,0x00,0x40,0x40,0xC0,0xC1,0x40,0x40,0x00,0x01,0x07,0x0E,0x18,0x10,0x10,0x10,0x0F,0x0F,0x00,0x00},/*"G",39*/
{0x20,0xE0,0xE0,0x20,0x00,0x00,0x00,0x00,0x20,0xE0,0xE0,0x20,0x00,0xFF,0xFF,0x10,0x10,0x10,0x10,0x10,0x10,0xFF,0xFF,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10},/*"H",40*/
{0x00,0x00,0x20,0x20,0x20,0xE0,0xE0,0x20,0x20,0x20,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x10,0x1F,0x1F,0x10,0x10,0x10,0x00,0x00},/*"I",41*/
{0x00,0x00,0x00,0x00,0x20,0x20,0x20,0xE0,0xE0,0x20,0x20,0x20,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x60,0xE0,0x80,0x80,0x80,0xC0,0x7F,0x3F,0x00,0x00,0x00},/*"J",42*/
{0x20,0xE0,0xE0,0x20,0x00,0x00,0x20,0xA0,0x60,0x20,0x20,0x00,0x00,0xFF,0xFF,0x30,0x18,0x7C,0xE3,0xC0,0x00,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x01,0x13,0x1F,0x1C,0x18,0x10},/*"K",43*/
{0x20,0xE0,0xE0,0x20,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10,0x10,0x10,0x10,0x10,0x10,0x18,0x06,0x00},/*"L",44*/
{0x20,0xE0,0xE0,0xE0,0x00,0x00,0x00,0x00,0xE0,0xE0,0xE0,0x20,0x00,0xFF,0x01,0x3F,0xFE,0xC0,0xE0,0x1E,0x01,0xFF,0xFF,0x00,0x10,0x1F,0x10,0x00,0x03,0x1F,0x03,0x00,0x10,0x1F,0x1F,0x10},/*"M",45*/
{0x20,0xE0,0xE0,0xC0,0x00,0x00,0x00,0x00,0x00,0x20,0xE0,0x20,0x00,0xFF,0x00,0x03,0x07,0x1C,0x78,0xE0,0x80,0x00,0xFF,0x00,0x10,0x1F,0x10,0x00,0x00,0x00,0x00,0x00,0x03,0x0F,0x1F,0x00},/*"N",46*/
{0x00,0x00,0x80,0xC0,0x60,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x00,0xFE,0xFF,0x01,0x00,0x00,0x00,0x00,0x00,0xFF,0xFE,0x00,0x00,0x01,0x07,0x0E,0x18,0x10,0x10,0x18,0x0C,0x07,0x01,0x00},/*"O",47*/
{0x20,0xE0,0xE0,0x20,0x20,0x20,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0xFF,0xFF,0x20,0x20,0x20,0x20,0x20,0x30,0x1F,0x0F,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"P",48*/
{0x00,0x00,0x80,0xC0,0x60,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0x00,0xFE,0xFF,0x01,0x00,0x00,0x00,0x00,0x00,0xFF,0xFE,0x00,0x00,0x01,0x07,0x0E,0x11,0x11,0x13,0x3C,0x7C,0x67,0x21,0x00},/*"Q",49*/
{0x20,0xE0,0xE0,0x20,0x20,0x20,0x20,0x20,0x60,0xC0,0x80,0x00,0x00,0xFF,0xFF,0x10,0x10,0x30,0xF0,0xD0,0x08,0x0F,0x07,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x00,0x03,0x0F,0x1C,0x10,0x10},/*"R",50*/
{0x00,0x80,0xC0,0x60,0x20,0x20,0x20,0x20,0x40,0x40,0xE0,0x00,0x00,0x07,0x0F,0x0C,0x18,0x18,0x30,0x30,0x60,0xE0,0x81,0x00,0x00,0x1F,0x0C,0x08,0x10,0x10,0x10,0x10,0x18,0x0F,0x07,0x00},/*"S",51*/
{0x80,0x60,0x20,0x20,0x20,0xE0,0xE0,0x20,0x20,0x20,0x60,0x80,0x01,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x01,0x00,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x00,0x00},/*"T",52*/
{0x20,0xE0,0xE0,0x20,0x00,0x00,0x00,0x00,0x00,0x20,0xE0,0x20,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x07,0x0F,0x18,0x10,0x10,0x10,0x10,0x10,0x08,0x07,0x00},/*"U",53*/
{0x20,0x60,0xE0,0xE0,0x20,0x00,0x00,0x00,0x20,0xE0,0x60,0x20,0x00,0x00,0x07,0x7F,0xF8,0x80,0x00,0x80,0x7C,0x03,0x00,0x00,0x00,0x00,0x00,0x00,0x07,0x1F,0x1C,0x07,0x00,0x00,0x00,0x00},/*"V",54*/
{0x20,0xE0,0xE0,0x20,0x00,0xE0,0xE0,0x20,0x00,0x20,0xE0,0x20,0x00,0x07,0xFF,0xF8,0xE0,0x1F,0xFF,0xFC,0xE0,0x1F,0x00,0x00,0x00,0x00,0x03,0x1F,0x03,0x00,0x01,0x1F,0x03,0x00,0x00,0x00},/*"W",55*/
{0x00,0x20,0x60,0xE0,0xA0,0x00,0x00,0x20,0xE0,0x60,0x20,0x00,0x00,0x00,0x00,0x03,0x8F,0x7C,0xF8,0xC6,0x01,0x00,0x00,0x00,0x00,0x10,0x18,0x1E,0x13,0x00,0x01,0x17,0x1F,0x18,0x10,0x00},/*"X",56*/
{0x20,0x60,0xE0,0xE0,0x20,0x00,0x00,0x00,0x20,0xE0,0x60,0x20,0x00,0x00,0x01,0x07,0x3E,0xF8,0xE0,0x18,0x07,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x1F,0x1F,0x10,0x10,0x00,0x00,0x00},/*"Y",57*/
{0x00,0x80,0x60,0x20,0x20,0x20,0x20,0xA0,0xE0,0xE0,0x20,0x00,0x00,0x00,0x00,0x00,0xC0,0xF0,0x3E,0x0F,0x03,0x00,0x00,0x00,0x00,0x10,0x1C,0x1F,0x17,0x10,0x10,0x10,0x10,0x18,0x06,0x00},/*"Z",58*/
{0x00,0x00,0x00,0x00,0x00,0xFC,0x04,0x04,0x04,0x04,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x7F,0x40,0x40,0x40,0x40,0x40,0x00},/*"[",59*/
{0x00,0x00,0x10,0xE0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x03,0x1C,0x60,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x03,0x0C,0x70,0x80,0x00},/*"\",60*/
{0x00,0x00,0x04,0x04,0x04,0x04,0x04,0xFC,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x40,0x40,0x40,0x40,0x40,0x7F,0x00,0x00,0x00,0x00},/*"]",61*/
{0x00,0x00,0x00,0x10,0x08,0x0C,0x04,0x0C,0x08,0x10,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"^",62*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x80},/*"_",63*/
{0x00,0x00,0x00,0x04,0x04,0x08,0x08,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"`",64*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x98,0xD8,0x44,0x64,0x24,0x24,0xFC,0xF8,0x00,0x00,0x00,0x0F,0x1F,0x18,0x10,0x10,0x10,0x08,0x1F,0x1F,0x10,0x18},/*"a",65*/
{0x00,0x20,0xE0,0xF0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x18,0x08,0x04,0x04,0x0C,0xF8,0xF0,0x00,0x00,0x00,0x1F,0x0F,0x18,0x10,0x10,0x10,0x18,0x0F,0x03,0x00},/*"b",66*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xE0,0xF8,0x18,0x04,0x04,0x04,0x3C,0x38,0x00,0x00,0x00,0x00,0x03,0x0F,0x0C,0x10,0x10,0x10,0x10,0x08,0x06,0x00,0x00},/*"c",67*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x20,0xE0,0xF0,0x00,0x00,0x00,0xE0,0xF8,0x1C,0x04,0x04,0x04,0x08,0xFF,0xFF,0x00,0x00,0x00,0x03,0x0F,0x18,0x10,0x10,0x10,0x08,0x1F,0x0F,0x08,0x00},/*"d",68*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xE0,0xF8,0x48,0x44,0x44,0x44,0x4C,0x78,0x70,0x00,0x00,0x00,0x03,0x0F,0x0C,0x18,0x10,0x10,0x10,0x08,0x04,0x00},/*"e",69*/
{0x00,0x00,0x00,0x00,0x80,0xC0,0x60,0x20,0x20,0xE0,0xC0,0x00,0x00,0x04,0x04,0x04,0xFF,0xFF,0x04,0x04,0x04,0x04,0x00,0x00,0x00,0x00,0x10,0x10,0x1F,0x1F,0x10,0x10,0x10,0x00,0x00,0x00},/*"f",70*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x70,0xF8,0x8C,0x04,0x04,0x8C,0xF8,0x74,0x04,0x0C,0x00,0x70,0x76,0xCF,0x8D,0x8D,0x8D,0x89,0xC8,0x78,0x70,0x00},/*"g",71*/
{0x00,0x20,0xE0,0xF0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x08,0x04,0x04,0x04,0xFC,0xF8,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x10,0x1F,0x1F,0x10,0x00},/*"h",72*/
{0x00,0x00,0x00,0x00,0x00,0x60,0x60,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x04,0x04,0xFC,0xFC,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x10,0x1F,0x1F,0x10,0x10,0x10,0x00,0x00},/*"i",73*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x60,0x60,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x04,0x04,0xFC,0xFC,0x00,0x00,0x00,0x00,0x00,0xC0,0xC0,0x80,0x80,0xC0,0x7F,0x3F,0x00,0x00,0x00},/*"j",74*/
{0x00,0x20,0xE0,0xF0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x80,0xC0,0xF4,0x1C,0x04,0x04,0x00,0x00,0x00,0x10,0x1F,0x1F,0x11,0x00,0x03,0x1F,0x1C,0x10,0x10,0x00},/*"k",75*/
{0x00,0x00,0x20,0x20,0x20,0xE0,0xF0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x10,0x10,0x1F,0x1F,0x10,0x10,0x10,0x00,0x00},/*"l",76*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0xFC,0xFC,0x08,0x04,0xFC,0xFC,0x08,0x04,0xFC,0xFC,0x00,0x10,0x1F,0x1F,0x10,0x00,0x1F,0x1F,0x10,0x00,0x1F,0x1F,0x10},/*"m",77*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0xFC,0xFC,0x08,0x08,0x04,0x04,0xFC,0xF8,0x00,0x00,0x00,0x10,0x1F,0x1F,0x10,0x00,0x00,0x10,0x1F,0x1F,0x10,0x00},/*"n",78*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xE0,0xF0,0x18,0x0C,0x04,0x04,0x0C,0x18,0xF0,0xE0,0x00,0x00,0x03,0x0F,0x0C,0x10,0x10,0x10,0x10,0x0C,0x0F,0x03,0x00},/*"o",79*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0xFC,0xFC,0x08,0x04,0x04,0x04,0x0C,0xF8,0xF0,0x00,0x00,0x80,0xFF,0xFF,0x88,0x90,0x10,0x10,0x1C,0x0F,0x03,0x00},/*"p",80*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xE0,0xF8,0x1C,0x04,0x04,0x04,0x08,0xF8,0xFC,0x00,0x00,0x00,0x03,0x0F,0x18,0x10,0x10,0x90,0x88,0xFF,0xFF,0x80,0x00},/*"q",81*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x04,0x04,0xFC,0xFC,0x10,0x08,0x04,0x04,0x0C,0x0C,0x00,0x10,0x10,0x10,0x1F,0x1F,0x10,0x10,0x10,0x00,0x00,0x00,0x00},/*"r",82*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x30,0x78,0xCC,0xC4,0x84,0x84,0x84,0x0C,0x1C,0x00,0x00,0x00,0x1E,0x18,0x10,0x10,0x10,0x11,0x19,0x0F,0x06,0x00},/*"s",83*/
{0x00,0x00,0x00,0x00,0x00,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x04,0x04,0xFF,0xFF,0x04,0x04,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x0F,0x1F,0x10,0x10,0x10,0x0C,0x00,0x00},/*"t",84*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0xFC,0xFE,0x00,0x00,0x00,0x04,0xFC,0xFE,0x00,0x00,0x00,0x00,0x0F,0x1F,0x18,0x10,0x10,0x08,0x1F,0x0F,0x08,0x00},/*"u",85*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x0C,0x3C,0xFC,0xC4,0x00,0x00,0xC4,0x3C,0x0C,0x04,0x00,0x00,0x00,0x00,0x01,0x0F,0x1E,0x0E,0x01,0x00,0x00,0x00},/*"v",86*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x3C,0xFC,0xC4,0x00,0xE4,0x7C,0xFC,0x84,0x80,0x7C,0x04,0x00,0x00,0x07,0x1F,0x07,0x00,0x00,0x07,0x1F,0x07,0x00,0x00},/*"w",87*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x04,0x1C,0x7C,0xE4,0xC0,0x34,0x1C,0x04,0x04,0x00,0x00,0x10,0x10,0x1C,0x16,0x01,0x13,0x1F,0x1C,0x18,0x10,0x00},/*"x",88*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x04,0x0C,0x3C,0xFC,0xC4,0x00,0xC4,0x3C,0x04,0x04,0x00,0x00,0x00,0xC0,0x80,0xC1,0x37,0x0E,0x01,0x00,0x00,0x00,0x00},/*"y",89*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1C,0x04,0x04,0xC4,0xF4,0x7C,0x1C,0x04,0x00,0x00,0x00,0x00,0x10,0x1C,0x1F,0x17,0x11,0x10,0x10,0x18,0x0E,0x00},/*"z",90*/
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xF8,0x0C,0x04,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x10,0x28,0xEF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x3F,0x60,0x40,0x00,0x00},/*"{",91*/
{0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x00,0x00},/*"|",92*/
{0x00,0x00,0x04,0x0C,0xF8,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xEF,0x28,0x10,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x40,0x60,0x3F,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"}",93*/
{0x00,0x18,0x06,0x02,0x02,0x04,0x08,0x10,0x20,0x20,0x30,0x08,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"~",94*/
};
const unsigned char Hzk1[][32]={
/*-- ??: zhao --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x02,0x04,0x78,0x52,0xD2,0x52,0x72,0x07,0x22,0xCA,0x6A,0x53,0x68,0x48,0x48,0x00,//0
0x82,0x82,0x8A,0xAA,0xAA,0xAA,0xAA,0xFF,0xAA,0xAA,0xAA,0xEA,0x8A,0x82,0x82,0x00,
/*-- ??: qing --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x00,0x00,0x3F,0x20,0x21,0x21,0x21,0xA1,0x6F,0x21,0x21,0x21,0x21,0x21,0x20,0x00,//1
0x02,0x0C,0xF0,0x01,0x02,0x04,0x18,0x60,0x80,0x60,0x18,0x04,0x02,0x01,0x01,0x00,
/*-- ??: xue --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x02,0x0C,0x88,0x69,0x09,0x09,0x89,0x69,0x09,0x09,0x19,0x28,0xC8,0x0A,0x0C,0x00,//2
0x20,0x20,0x20,0x20,0x20,0x22,0x21,0x7E,0x60,0xA0,0x20,0x20,0x20,0x20,0x20,0x00,
/*-- ??: yuan --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x00,0x7F,0x44,0x5A,0x61,0x08,0x30,0x24,0x24,0xA4,0x64,0x24,0x24,0x28,0x30,0x00,//3
0x00,0xFF,0x20,0x10,0xE0,0x01,0x82,0x8C,0xF0,0x80,0x80,0xFC,0x82,0x82,0x8E,0x00,
/*-- ??: wang --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x00,0x40,0x41,0x41,0x41,0x41,0x41,0x7F,0x41,0x41,0x41,0x41,0x41,0x40,0x00,0x00,//4
0x02,0x02,0x02,0x02,0x02,0x02,0x02,0xFE,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0x00,
/*-- ??: bai --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x40,0x40,0x47,0x44,0x44,0x4C,0x54,0x64,0x44,0x44,0x44,0x44,0x47,0x40,0x40,0x00,//5
0x00,0x00,0xFF,0x42,0x42,0x42,0x42,0x42,0x42,0x42,0x42,0x42,0xFF,0x00,0x00,0x00,
/*-- ??: he --*/
/*-- ??12; ??????????:?x?=16x16 --*/
0x02,0x02,0x04,0x04,0x0A,0x12,0x22,0xC2,0x22,0x12,0x0A,0x04,0x04,0x02,0x02,0x00,//6
0x00,0x00,0x00,0x7F,0x42,0x42,0x42,0x42,0x42,0x42,0x42,0x7F,0x00,0x00,0x00,0x00,
};
const unsigned char Hzk2[][72]={
{0x00,0x00,0x00,0xC0,0x80,0x80,0x80,0x80,0x80,0x80,0x80,0xFC,0x84,0x80,0x80,0x80,0x80,0x80,0x80,0xC0,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0x7F,0x40,0x40,0x40,0x40,0x40,0x40,0xFF,0x40,0x40,0x40,0x40,0x40,0x40,0x40,0xFF,0x7F,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x7F,0x70,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"ÖÐ",0*/
};
const unsigned char Hzk3[][128]={
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFC,0xF8,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFE,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0xFF,0xFF,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0x02,0xFF,0x03,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1F,0x1F,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0xFF,0xFF,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0x04,0x1F,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x7F,0x3F,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"ÖÐ",0*/
};
const unsigned char Hzk4[][512]={
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xF8,0xF8,0xF0,0xF0,0x70,0x20,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0x80,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFE,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0xFF,0xFF,0xFF,0xFF,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0x06,0xFF,0xFF,0xFF,0xFF,0x07,0x02,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0xFF,0xFF,0xFF,0xFF,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0x18,0xFF,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x02,0x01,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x01,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1F,0x1F,0x0F,0x0F,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},/*"ÖÐ",0*/
};
#endif
delay.c
#include "delay.h"
#include "sys.h"
//
//Èç¹ûʹÓÃucos,Ôò°üÀ¨ÏÂÃæµÄÍ·Îļþ¼´¿É.
#if SYSTEM_SUPPORT_UCOS
#include "includes.h" //ucos ʹÓÃ
#endif
static u8 fac_us=0;//usÑÓʱ±¶³ËÊý
static u16 fac_ms=0;//msÑÓʱ±¶³ËÊý
#ifdef OS_CRITICAL_METHOD //Èç¹ûOS_CRITICAL_METHOD¶¨ÒåÁË,˵Ã÷ʹÓÃucosIIÁË.
//systickÖжϷþÎñº¯Êý,ʹÓÃucosʱÓõ½
void SysTick_Handler(void)
{
OSIntEnter(); //½øÈëÖжÏ
OSTimeTick(); //µ÷ÓÃucosµÄʱÖÓ·þÎñ³ÌÐò
OSIntExit(); //´¥·¢ÈÎÎñÇл»ÈíÖжÏ
}
#endif
//³õʼ»¯ÑÓ³Ùº¯Êý
//µ±Ê¹ÓÃucosµÄʱºò,´Ëº¯Êý»á³õʼ»¯ucosµÄʱÖÓ½ÚÅÄ
//SYSTICKµÄʱÖӹ̶¨ÎªHCLKʱÖÓµÄ1/8
//SYSCLK:ϵͳʱÖÓ
void delay_init()
{
#ifdef OS_CRITICAL_METHOD //Èç¹ûOS_CRITICAL_METHOD¶¨ÒåÁË,˵Ã÷ʹÓÃucosIIÁË.
u32 reload;
#endif
SysTick_CLKSourceConfig(SysTick_CLKSource_HCLK_Div8); //Ñ¡ÔñÍⲿʱÖÓ HCLK/8
fac_us=SystemCoreClock/8000000; //ΪϵͳʱÖÓµÄ1/8
#ifdef OS_CRITICAL_METHOD //Èç¹ûOS_CRITICAL_METHOD¶¨ÒåÁË,˵Ã÷ʹÓÃucosIIÁË.
reload=SystemCoreClock/8000000; //ÿÃëÖӵļÆÊý´ÎÊý µ¥Î»ÎªK
reload*=1000000/OS_TICKS_PER_SEC;//¸ù¾ÝOS_TICKS_PER_SECÉ趨Òç³öʱ¼ä
//reloadΪ24λ¼Ä´æÆ÷,×î´óÖµ:16777216,ÔÚ72MÏÂ,Ô¼ºÏ1.86s×óÓÒ
fac_ms=1000/OS_TICKS_PER_SEC;//´ú±íucos¿ÉÒÔÑÓʱµÄ×îÉÙµ¥Î»
SysTick->CTRL|=SysTick_CTRL_TICKINT_Msk; //¿ªÆôSYSTICKÖжÏ
SysTick->LOAD=reload; //ÿ1/OS_TICKS_PER_SECÃëÖжÏÒ»´Î
SysTick->CTRL|=SysTick_CTRL_ENABLE_Msk; //¿ªÆôSYSTICK
#else
fac_ms=(u16)fac_us*1000;//·ÇucosÏÂ,´ú±íÿ¸ömsÐèÒªµÄsystickʱÖÓÊý
#endif
}
#ifdef OS_CRITICAL_METHOD //ʹÓÃÁËucos
//ÑÓʱnus
//nusΪҪÑÓʱµÄusÊý.
void delay_us(u32 nus)
{
u32 ticks;
u32 told,tnow,tcnt=0;
u32 reload=SysTick->LOAD; //LOADµÄÖµ
ticks=nus*fac_us; //ÐèÒªµÄ½ÚÅÄÊý
tcnt=0;
told=SysTick->VAL; //¸Õ½øÈëʱµÄ¼ÆÊýÆ÷Öµ
while(1)
{
tnow=SysTick->VAL;
if(tnow!=told)
{
if(tnow<told)tcnt+=told-tnow;//ÕâÀï×¢ÒâÒ»ÏÂSYSTICKÊÇÒ»¸öµÝ¼õµÄ¼ÆÊýÆ÷¾Í¿ÉÒÔÁË.
else tcnt+=reload-tnow+told;
told=tnow;
if(tcnt>=ticks)break;//ʱ¼ä³¬¹ý/µÈÓÚÒªÑÓ³ÙµÄʱ¼ä,ÔòÍ˳ö.
}
};
}
//ÑÓʱnms
//nms:ÒªÑÓʱµÄmsÊý
void delay_ms(u16 nms)
{
if(OSRunning==TRUE)//Èç¹ûosÒѾÔÚÅÜÁË
{
if(nms>=fac_ms)//ÑÓʱµÄʱ¼ä´óÓÚucosµÄ×îÉÙʱ¼äÖÜÆÚ
{
OSTimeDly(nms/fac_ms);//ucosÑÓʱ
}
nms%=fac_ms; //ucosÒѾÎÞ·¨ÌṩÕâôСµÄÑÓʱÁË,²ÉÓÃÆÕͨ·½Ê½ÑÓʱ
}
delay_us((u32)(nms*1000)); //ÆÕͨ·½Ê½ÑÓʱ,´ËʱucosÎÞ·¨Æô¶¯µ÷¶È.
}
#else//²»ÓÃucosʱ
//ÑÓʱnus
//nusΪҪÑÓʱµÄusÊý.
void delay_us(u32 nus)
{
u32 temp;
SysTick->LOAD=nus*fac_us; //ʱ¼ä¼ÓÔØ
SysTick->VAL=0x00; //Çå¿Õ¼ÆÊýÆ÷
SysTick->CTRL|=SysTick_CTRL_ENABLE_Msk ; //¿ªÊ¼µ¹Êý
do
{
temp=SysTick->CTRL;
}
while(temp&0x01&&!(temp&(1<<16)));//µÈ´ýʱ¼äµ½´ï
SysTick->CTRL&=~SysTick_CTRL_ENABLE_Msk; //¹Ø±Õ¼ÆÊýÆ÷
SysTick->VAL =0X00; //Çå¿Õ¼ÆÊýÆ÷
}
//ÑÓʱnms
//×¢ÒânmsµÄ·¶Î§
//SysTick->LOADΪ24λ¼Ä´æÆ÷,ËùÒÔ,×î´óÑÓʱΪ:
//nms<=0xffffff*8*1000/SYSCLK
//SYSCLKµ¥Î»ÎªHz,nmsµ¥Î»Îªms
//¶Ô72MÌõ¼þÏÂ,nms<=1864
void delay_ms(u16 nms)
{
u32 temp;
SysTick->LOAD=(u32)nms*fac_ms;//ʱ¼ä¼ÓÔØ(SysTick->LOADΪ24bit)
SysTick->VAL =0x00; //Çå¿Õ¼ÆÊýÆ÷
SysTick->CTRL|=SysTick_CTRL_ENABLE_Msk ; //¿ªÊ¼µ¹Êý
do
{
temp=SysTick->CTRL;
}
while(temp&0x01&&!(temp&(1<<16)));//µÈ´ýʱ¼äµ½´ï
SysTick->CTRL&=~SysTick_CTRL_ENABLE_Msk; //¹Ø±Õ¼ÆÊýÆ÷
SysTick->VAL =0X00; //Çå¿Õ¼ÆÊýÆ÷
}
#endif
delay.h
#ifndef __DELAY_H
#define __DELAY_H
#include "sys.h"
void delay_init(void);
void delay_ms(u16 nms);
void delay_us(u32 nus);
#endif
stdlib.h
/* stdlib.h: ANSI draft (X3J11 May 88) library header, section 4.10 */
/* Copyright (C) Codemist Ltd., 1988-1993. */
/* Copyright 1991-1998,2014 ARM Limited. All rights reserved. */
/*
* RCS $Revision$
* Checkin $Date$
* Revising $Author: agrant $
*/
/*
* stdlib.h declares four types, several general purpose functions,
* and defines several macros.
*/
#ifndef __stdlib_h
#define __stdlib_h
#define __ARMCLIB_VERSION 5060037
#if defined(__clang__) || (defined(__ARMCC_VERSION) && !defined(__STRICT_ANSI__))
/* armclang and non-strict armcc allow 'long long' in system headers */
#define __LONGLONG long long
#else
/* strict armcc has '__int64' */
#define __LONGLONG __int64
#endif
#define _ARMABI __declspec(__nothrow)
#define _ARMABI_PURE __declspec(__nothrow) __attribute__((const))
#define _ARMABI_NORETURN __declspec(__nothrow) __declspec(__noreturn)
#define _ARMABI_THROW
#ifndef __STDLIB_DECLS
#define __STDLIB_DECLS
/*
* Some of these declarations are new in C99. To access them in C++
* you can use -D__USE_C99_STDLIB (or -D__USE_C99ALL).
*/
#ifndef __USE_C99_STDLIB
#if defined(__USE_C99_ALL) || (defined(__STDC_VERSION__) && 199901L <= __STDC_VERSION__) || (defined(__cplusplus) && 201103L <= __cplusplus)
#define __USE_C99_STDLIB 1
#endif
#endif
#undef __CLIBNS
#ifdef __cplusplus
namespace std {
#define __CLIBNS ::std::
extern "C" {
#else
#define __CLIBNS
#endif /* __cplusplus */
#if defined(__cplusplus) || !defined(__STRICT_ANSI__)
/* unconditional in C++ and non-strict C for consistency of debug info */
#if __sizeof_ptr == 8
typedef unsigned long size_t; /* see <stddef.h> */
#else
typedef unsigned int size_t; /* see <stddef.h> */
#endif
#elif !defined(__size_t)
#define __size_t 1
#if __sizeof_ptr == 8
typedef unsigned long size_t; /* see <stddef.h> */
#else
typedef unsigned int size_t; /* see <stddef.h> */
#endif
#endif
#undef NULL
#define NULL 0 /* see <stddef.h> */
#ifndef __cplusplus /* wchar_t is a builtin type for C++ */
#if !defined(__STRICT_ANSI__)
/* unconditional in non-strict C for consistency of debug info */
#if defined(__WCHAR32) || (defined(__ARM_SIZEOF_WCHAR_T) && __ARM_SIZEOF_WCHAR_T == 4)
typedef unsigned int wchar_t; /* see <stddef.h> */
#else
typedef unsigned short wchar_t; /* see <stddef.h> */
#endif
#elif !defined(__wchar_t)
#define __wchar_t 1
#if defined(__WCHAR32) || (defined(__ARM_SIZEOF_WCHAR_T) && __ARM_SIZEOF_WCHAR_T == 4)
typedef unsigned int wchar_t; /* see <stddef.h> */
#else
typedef unsigned short wchar_t; /* see <stddef.h> */
#endif
#endif
#endif
typedef struct div_t { int quot, rem; } div_t;
/* type of the value returned by the div function. */
typedef struct ldiv_t { long int quot, rem; } ldiv_t;
/* type of the value returned by the ldiv function. */
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
typedef struct lldiv_t { __LONGLONG quot, rem; } lldiv_t;
/* type of the value returned by the lldiv function. */
#endif
#ifdef __EXIT_FAILURE
# define EXIT_FAILURE __EXIT_FAILURE
/*
* an integral expression which may be used as an argument to the exit
* function to return unsuccessful termination status to the host
* environment.
*/
#else
# define EXIT_FAILURE 1 /* unixoid */
#endif
#define EXIT_SUCCESS 0
/*
* an integral expression which may be used as an argument to the exit
* function to return successful termination status to the host
* environment.
*/
/*
* Defining __USE_ANSI_EXAMPLE_RAND at compile time switches to
* the example implementation of rand() and srand() provided in
* the ANSI C standard. This implementation is very poor, but is
* provided for completeness.
*/
#ifdef __USE_ANSI_EXAMPLE_RAND
#define srand _ANSI_srand
#define rand _ANSI_rand
#define RAND_MAX 0x7fff
#else
#define RAND_MAX 0x7fffffff
#endif
/*
* RAND_MAX: an integral constant expression, the value of which
* is the maximum value returned by the rand function.
*/
extern _ARMABI int __aeabi_MB_CUR_MAX(void);
#define MB_CUR_MAX ( __aeabi_MB_CUR_MAX() )
/*
* a positive integer expression whose value is the maximum number of bytes
* in a multibyte character for the extended character set specified by the
* current locale (category LC_CTYPE), and whose value is never greater
* than MB_LEN_MAX.
*/
/*
* If the compiler supports signalling nans as per N965 then it
* will define __SUPPORT_SNAN__, in which case a user may define
* _WANT_SNAN in order to obtain a compliant version of the strtod
* family of functions.
*/
#if defined(__SUPPORT_SNAN__) && defined(_WANT_SNAN)
#pragma import(__use_snan)
#endif
extern _ARMABI double atof(const char * /*nptr*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to double
* representation.
* Returns: the converted value.
*/
extern _ARMABI int atoi(const char * /*nptr*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to int
* representation.
* Returns: the converted value.
*/
extern _ARMABI long int atol(const char * /*nptr*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to long int
* representation.
* Returns: the converted value.
*/
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
extern _ARMABI __LONGLONG atoll(const char * /*nptr*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to
* long long int representation.
* Returns: the converted value.
*/
#endif
extern _ARMABI double strtod(const char * __restrict /*nptr*/, char ** __restrict /*endptr*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to double
* representation. First it decomposes the input string into three parts:
* an initial, possibly empty, sequence of white-space characters (as
* specified by the isspace function), a subject sequence resembling a
* floating point constant; and a final string of one or more unrecognised
* characters, including the terminating null character of the input string.
* Then it attempts to convert the subject sequence to a floating point
* number, and returns the result. A pointer to the final string is stored
* in the object pointed to by endptr, provided that endptr is not a null
* pointer.
* Returns: the converted value if any. If no conversion could be performed,
* zero is returned. If the correct value is outside the range of
* representable values, plus or minus HUGE_VAL is returned
* (according to the sign of the value), and the value of the macro
* ERANGE is stored in errno. If the correct value would cause
* underflow, zero is returned and the value of the macro ERANGE is
* stored in errno.
*/
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
extern _ARMABI float strtof(const char * __restrict /*nptr*/, char ** __restrict /*endptr*/) __attribute__((__nonnull__(1)));
extern _ARMABI long double strtold(const char * __restrict /*nptr*/, char ** __restrict /*endptr*/) __attribute__((__nonnull__(1)));
/*
* same as strtod, but return float and long double respectively.
*/
#endif
extern _ARMABI long int strtol(const char * __restrict /*nptr*/,
char ** __restrict /*endptr*/, int /*base*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to long int
* representation. First it decomposes the input string into three parts:
* an initial, possibly empty, sequence of white-space characters (as
* specified by the isspace function), a subject sequence resembling an
* integer represented in some radix determined by the value of base, and a
* final string of one or more unrecognised characters, including the
* terminating null character of the input string. Then it attempts to
* convert the subject sequence to an integer, and returns the result.
* If the value of base is 0, the expected form of the subject sequence is
* that of an integer constant (described in ANSI Draft, section 3.1.3.2),
* optionally preceded by a '+' or '-' sign, but not including an integer
* suffix. If the value of base is between 2 and 36, the expected form of
* the subject sequence is a sequence of letters and digits representing an
* integer with the radix specified by base, optionally preceded by a plus
* or minus sign, but not including an integer suffix. The letters from a
* (or A) through z (or Z) are ascribed the values 10 to 35; only letters
* whose ascribed values are less than that of the base are permitted. If
* the value of base is 16, the characters 0x or 0X may optionally precede
* the sequence of letters and digits following the sign if present.
* A pointer to the final string is stored in the object
* pointed to by endptr, provided that endptr is not a null pointer.
* Returns: the converted value if any. If no conversion could be performed,
* zero is returned and nptr is stored in *endptr.
* If the correct value is outside the range of
* representable values, LONG_MAX or LONG_MIN is returned
* (according to the sign of the value), and the value of the
* macro ERANGE is stored in errno.
*/
extern _ARMABI unsigned long int strtoul(const char * __restrict /*nptr*/,
char ** __restrict /*endptr*/, int /*base*/) __attribute__((__nonnull__(1)));
/*
* converts the initial part of the string pointed to by nptr to unsigned
* long int representation. First it decomposes the input string into three
* parts: an initial, possibly empty, sequence of white-space characters (as
* determined by the isspace function), a subject sequence resembling an
* unsigned integer represented in some radix determined by the value of
* base, and a final string of one or more unrecognised characters,
* including the terminating null character of the input string. Then it
* attempts to convert the subject sequence to an unsigned integer, and
* returns the result. If the value of base is zero, the expected form of
* the subject sequence is that of an integer constant (described in ANSI
* Draft, section 3.1.3.2), optionally preceded by a '+' or '-' sign, but
* not including an integer suffix. If the value of base is between 2 and
* 36, the expected form of the subject sequence is a sequence of letters
* and digits representing an integer with the radix specified by base,
* optionally preceded by a '+' or '-' sign, but not including an integer
* suffix. The letters from a (or A) through z (or Z) stand for the values
* 10 to 35; only letters whose ascribed values are less than that of the
* base are permitted. If the value of base is 16, the characters 0x or 0X
* may optionally precede the sequence of letters and digits following the
* sign, if present. A pointer to the final string is stored in the object
* pointed to by endptr, provided that endptr is not a null pointer.
* Returns: the converted value if any. If no conversion could be performed,
* zero is returned and nptr is stored in *endptr.
* If the correct value is outside the range of
* representable values, ULONG_MAX is returned, and the value of
* the macro ERANGE is stored in errno.
*/
/* C90 reserves all names beginning with 'str' */
extern _ARMABI __LONGLONG strtoll(const char * __restrict /*nptr*/,
char ** __restrict /*endptr*/, int /*base*/)
__attribute__((__nonnull__(1)));
/*
* as strtol but returns a long long int value. If the correct value is
* outside the range of representable values, LLONG_MAX or LLONG_MIN is
* returned (according to the sign of the value), and the value of the
* macro ERANGE is stored in errno.
*/
extern _ARMABI unsigned __LONGLONG strtoull(const char * __restrict /*nptr*/,
char ** __restrict /*endptr*/, int /*base*/)
__attribute__((__nonnull__(1)));
/*
* as strtoul but returns an unsigned long long int value. If the correct
* value is outside the range of representable values, ULLONG_MAX is returned,
* and the value of the macro ERANGE is stored in errno.
*/
extern _ARMABI int rand(void);
/*
* Computes a sequence of pseudo-random integers in the range 0 to RAND_MAX.
* Uses an additive generator (Mitchell & Moore) of the form:
* Xn = (X[n-24] + X[n-55]) MOD 2^31
* This is described in section 3.2.2 of Knuth, vol 2. It's period is
* in excess of 2^55 and its randomness properties, though unproven, are
* conjectured to be good. Empirical testing since 1958 has shown no flaws.
* Returns: a pseudo-random integer.
*/
extern _ARMABI void srand(unsigned int /*seed*/);
/*
* uses its argument as a seed for a new sequence of pseudo-random numbers
* to be returned by subsequent calls to rand. If srand is then called with
* the same seed value, the sequence of pseudo-random numbers is repeated.
* If rand is called before any calls to srand have been made, the same
* sequence is generated as when srand is first called with a seed value
* of 1.
*/
struct _rand_state { int __x[57]; };
extern _ARMABI int _rand_r(struct _rand_state *);
extern _ARMABI void _srand_r(struct _rand_state *, unsigned int);
struct _ANSI_rand_state { int __x[1]; };
extern _ARMABI int _ANSI_rand_r(struct _ANSI_rand_state *);
extern _ARMABI void _ANSI_srand_r(struct _ANSI_rand_state *, unsigned int);
/*
* Re-entrant variants of both flavours of rand, which operate on
* an explicitly supplied state buffer.
*/
extern _ARMABI void *calloc(size_t /*nmemb*/, size_t /*size*/);
/*
* allocates space for an array of nmemb objects, each of whose size is
* 'size'. The space is initialised to all bits zero.
* Returns: either a null pointer or a pointer to the allocated space.
*/
extern _ARMABI void free(void * /*ptr*/);
/*
* causes the space pointed to by ptr to be deallocated (i.e., made
* available for further allocation). If ptr is a null pointer, no action
* occurs. Otherwise, if ptr does not match a pointer earlier returned by
* calloc, malloc or realloc or if the space has been deallocated by a call
* to free or realloc, the behaviour is undefined.
*/
extern _ARMABI void *malloc(size_t /*size*/);
/*
* allocates space for an object whose size is specified by 'size' and whose
* value is indeterminate.
* Returns: either a null pointer or a pointer to the allocated space.
*/
extern _ARMABI void *realloc(void * /*ptr*/, size_t /*size*/);
/*
* changes the size of the object pointed to by ptr to the size specified by
* size. The contents of the object shall be unchanged up to the lesser of
* the new and old sizes. If the new size is larger, the value of the newly
* allocated portion of the object is indeterminate. If ptr is a null
* pointer, the realloc function behaves like a call to malloc for the
* specified size. Otherwise, if ptr does not match a pointer earlier
* returned by calloc, malloc or realloc, or if the space has been
* deallocated by a call to free or realloc, the behaviour is undefined.
* If the space cannot be allocated, the object pointed to by ptr is
* unchanged. If size is zero and ptr is not a null pointer, the object it
* points to is freed.
* Returns: either a null pointer or a pointer to the possibly moved
* allocated space.
*/
#if !defined(__STRICT_ANSI__)
extern _ARMABI int posix_memalign(void ** /*ret*/, size_t /*alignment*/, size_t /*size*/);
/*
* allocates space for an object of size 'size', aligned to a
* multiple of 'alignment' (which must be a power of two and at
* least 4).
*
* On success, a pointer to the allocated object is stored in
* *ret, and zero is returned. On failure, the return value is
* either ENOMEM (allocation failed because no suitable piece of
* memory was available) or EINVAL (the 'alignment' parameter was
* invalid).
*/
#endif
typedef int (*__heapprt)(void *, char const *, ...);
extern _ARMABI void __heapstats(int (* /*dprint*/)(void * /*param*/,
char const * /*format*/, ...),
void * /*param*/) __attribute__((__nonnull__(1)));
/*
* reports current heap statistics (eg. number of free blocks in
* the free-list). Output is as implementation-defined free-form
* text, provided via the dprint function. `param' gives an
* extra data word to pass to dprint. You can call
* __heapstats(fprintf,stdout) by casting fprintf to the above
* function type; the typedef `__heapprt' is provided for this
* purpose.
*
* `dprint' will not be called while the heap is being examined,
* so it can allocate memory itself without trouble.
*/
extern _ARMABI int __heapvalid(int (* /*dprint*/)(void * /*param*/,
char const * /*format*/, ...),
void * /*param*/, int /*verbose*/) __attribute__((__nonnull__(1)));
/*
* performs a consistency check on the heap. Errors are reported
* through dprint, like __heapstats. If `verbose' is nonzero,
* full diagnostic information on the heap state is printed out.
*
* This routine probably won't work if the heap isn't a
* contiguous chunk (for example, if __user_heap_extend has been
* overridden).
*
* `dprint' may be called while the heap is being examined or
* even in an invalid state, so it must perform no memory
* allocation. In particular, if `dprint' calls (or is) a stdio
* function, the stream it outputs to must already have either
* been written to or been setvbuf'ed, or else the system will
* allocate buffer space for it on the first call to dprint.
*/
extern _ARMABI_NORETURN void abort(void);
/*
* causes abnormal program termination to occur, unless the signal SIGABRT
* is being caught and the signal handler does not return. Whether open
* output streams are flushed or open streams are closed or temporary
* files removed is implementation-defined.
* An implementation-defined form of the status 'unsuccessful termination'
* is returned to the host environment by means of a call to
* raise(SIGABRT).
*/
extern _ARMABI int atexit(void (* /*func*/)(void)) __attribute__((__nonnull__(1)));
/*
* registers the function pointed to by func, to be called without its
* arguments at normal program termination. It is possible to register at
* least 32 functions.
* Returns: zero if the registration succeeds, nonzero if it fails.
*/
#if defined(__EDG__) && !defined(__GNUC__)
#define __LANGUAGE_LINKAGE_CHANGES_FUNCTION_TYPE
#endif
#if defined(__cplusplus) && defined(__LANGUAGE_LINKAGE_CHANGES_FUNCTION_TYPE)
/* atexit that takes a ptr to a function with C++ linkage
* but not in GNU mode
*/
typedef void (* __C_exitfuncptr)();
extern "C++"
inline int atexit(void (* __func)()) {
return atexit((__C_exitfuncptr)__func);
}
#endif
extern _ARMABI_NORETURN void exit(int /*status*/);
/*
* causes normal program termination to occur. If more than one call to the
* exit function is executed by a program, the behaviour is undefined.
* First, all functions registered by the atexit function are called, in the
* reverse order of their registration.
* Next, all open output streams are flushed, all open streams are closed,
* and all files created by the tmpfile function are removed.
* Finally, control is returned to the host environment. If the value of
* status is zero or EXIT_SUCCESS, an implementation-defined form of the
* status 'successful termination' is returned. If the value of status is
* EXIT_FAILURE, an implementation-defined form of the status
* 'unsuccessful termination' is returned. Otherwise the status returned
* is implementation-defined.
*/
extern _ARMABI_NORETURN void _Exit(int /*status*/);
/*
* causes normal program termination to occur. No functions registered
* by the atexit function are called.
* In this implementation, all open output streams are flushed, all
* open streams are closed, and all files created by the tmpfile function
* are removed.
* Control is returned to the host environment. The status returned to
* the host environment is determined in the same way as for 'exit'.
*/
extern _ARMABI char *getenv(const char * /*name*/) __attribute__((__nonnull__(1)));
/*
* searches the environment list, provided by the host environment, for a
* string that matches the string pointed to by name. The set of environment
* names and the method for altering the environment list are
* implementation-defined.
* Returns: a pointer to a string associated with the matched list member.
* The array pointed to shall not be modified by the program, but
* may be overwritten by a subsequent call to the getenv function.
* If the specified name cannot be found, a null pointer is
* returned.
*/
extern _ARMABI int system(const char * /*string*/);
/*
* passes the string pointed to by string to the host environment to be
* executed by a command processor in an implementation-defined manner.
* A null pointer may be used for string, to inquire whether a command
* processor exists.
*
* Returns: If the argument is a null pointer, the system function returns
* non-zero only if a command processor is available. If the
* argument is not a null pointer, the system function returns an
* implementation-defined value.
*/
extern _ARMABI_THROW void *bsearch(const void * /*key*/, const void * /*base*/,
size_t /*nmemb*/, size_t /*size*/,
int (* /*compar*/)(const void *, const void *)) __attribute__((__nonnull__(1,2,5)));
/*
* searches an array of nmemb objects, the initial member of which is
* pointed to by base, for a member that matches the object pointed to by
* key. The size of each member of the array is specified by size.
* The contents of the array shall be in ascending sorted order according to
* a comparison function pointed to by compar, which is called with two
* arguments that point to the key object and to an array member, in that
* order. The function shall return an integer less than, equal to, or
* greater than zero if the key object is considered, respectively, to be
* less than, to match, or to be greater than the array member.
* Returns: a pointer to a matching member of the array, or a null pointer
* if no match is found. If two members compare as equal, which
* member is matched is unspecified.
*/
#if defined(__cplusplus) && defined(__LANGUAGE_LINKAGE_CHANGES_FUNCTION_TYPE)
/* bsearch that takes a ptr to a function with C++ linkage
* but not in GNU mode
*/
typedef int (* __C_compareprocptr)(const void *, const void *);
extern "C++"
void *bsearch(const void * __key, const void * __base,
size_t __nmemb, size_t __size,
int (* __compar)(const void *, const void *)) __attribute__((__nonnull__(1,2,5)));
extern "C++"
inline void *bsearch(const void * __key, const void * __base,
size_t __nmemb, size_t __size,
int (* __compar)(const void *, const void *)) {
return bsearch(__key, __base, __nmemb, __size, (__C_compareprocptr)__compar);
}
#endif
extern _ARMABI_THROW void qsort(void * /*base*/, size_t /*nmemb*/, size_t /*size*/,
int (* /*compar*/)(const void *, const void *)) __attribute__((__nonnull__(1,4)));
/*
* sorts an array of nmemb objects, the initial member of which is pointed
* to by base. The size of each object is specified by size.
* The contents of the array shall be in ascending order according to a
* comparison function pointed to by compar, which is called with two
* arguments that point to the objects being compared. The function shall
* return an integer less than, equal to, or greater than zero if the first
* argument is considered to be respectively less than, equal to, or greater
* than the second. If two members compare as equal, their order in the
* sorted array is unspecified.
*/
#if defined(__cplusplus) && defined(__LANGUAGE_LINKAGE_CHANGES_FUNCTION_TYPE)
/* qsort that takes a ptr to a function with C++ linkage
* but not in GNU mode
*/
extern "C++"
void qsort(void * __base, size_t __nmemb, size_t __size,
int (* __compar)(const void *, const void *)) __attribute__((__nonnull__(1,4)));
extern "C++"
inline void qsort(void * __base, size_t __nmemb, size_t __size,
int (* __compar)(const void *, const void *)) {
qsort(__base, __nmemb, __size, (__C_compareprocptr)__compar);
}
#endif
extern _ARMABI_PURE int abs(int /*j*/);
/*
* computes the absolute value of an integer j. If the result cannot be
* represented, the behaviour is undefined.
* Returns: the absolute value.
*/
extern _ARMABI_PURE div_t div(int /*numer*/, int /*denom*/);
/*
* computes the quotient and remainder of the division of the numerator
* numer by the denominator denom. If the division is inexact, the resulting
* quotient is the integer of lesser magnitude that is the nearest to the
* algebraic quotient. If the result cannot be represented, the behaviour is
* undefined; otherwise, quot * denom + rem shall equal numer.
* Returns: a structure of type div_t, comprising both the quotient and the
* remainder. the structure shall contain the following members,
* in either order.
* int quot; int rem;
*/
extern _ARMABI_PURE long int labs(long int /*j*/);
/*
* computes the absolute value of an long integer j. If the result cannot be
* represented, the behaviour is undefined.
* Returns: the absolute value.
*/
#ifdef __cplusplus
extern "C++" inline _ARMABI_PURE long abs(long int x) { return labs(x); }
#endif
extern _ARMABI_PURE ldiv_t ldiv(long int /*numer*/, long int /*denom*/);
/*
* computes the quotient and remainder of the division of the numerator
* numer by the denominator denom. If the division is inexact, the sign of
* the resulting quotient is that of the algebraic quotient, and the
* magnitude of the resulting quotient is the largest integer less than the
* magnitude of the algebraic quotient. If the result cannot be represented,
* the behaviour is undefined; otherwise, quot * denom + rem shall equal
* numer.
* Returns: a structure of type ldiv_t, comprising both the quotient and the
* remainder. the structure shall contain the following members,
* in either order.
* long int quot; long int rem;
*/
#ifdef __cplusplus
extern "C++" inline _ARMABI_PURE ldiv_t div(long int __numer, long int __denom) {
return ldiv(__numer, __denom);
}
#endif
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
extern _ARMABI_PURE __LONGLONG llabs(__LONGLONG /*j*/);
/*
* computes the absolute value of a long long integer j. If the
* result cannot be represented, the behaviour is undefined.
* Returns: the absolute value.
*/
#ifdef __cplusplus
extern "C++" inline _ARMABI_PURE __LONGLONG abs(__LONGLONG x) { return llabs(x); }
#endif
extern _ARMABI_PURE lldiv_t lldiv(__LONGLONG /*numer*/, __LONGLONG /*denom*/);
/*
* computes the quotient and remainder of the division of the numerator
* numer by the denominator denom. If the division is inexact, the sign of
* the resulting quotient is that of the algebraic quotient, and the
* magnitude of the resulting quotient is the largest integer less than the
* magnitude of the algebraic quotient. If the result cannot be represented,
* the behaviour is undefined; otherwise, quot * denom + rem shall equal
* numer.
* Returns: a structure of type lldiv_t, comprising both the quotient and the
* remainder. the structure shall contain the following members,
* in either order.
* long long quot; long long rem;
*/
#ifdef __cplusplus
extern "C++" inline _ARMABI_PURE lldiv_t div(__LONGLONG __numer, __LONGLONG __denom) {
return lldiv(__numer, __denom);
}
#endif
#endif
#if !(__ARM_NO_DEPRECATED_FUNCTIONS)
/*
* ARM real-time divide functions for guaranteed performance
*/
typedef struct __sdiv32by16 { int quot, rem; } __sdiv32by16;
typedef struct __udiv32by16 { unsigned int quot, rem; } __udiv32by16;
/* used int so that values return in separate regs, although 16-bit */
typedef struct __sdiv64by32 { int rem, quot; } __sdiv64by32;
__value_in_regs extern _ARMABI_PURE __sdiv32by16 __rt_sdiv32by16(
int /*numer*/,
short int /*denom*/);
/*
* Signed divide: (16-bit quot), (16-bit rem) = (32-bit) / (16-bit)
*/
__value_in_regs extern _ARMABI_PURE __udiv32by16 __rt_udiv32by16(
unsigned int /*numer*/,
unsigned short /*denom*/);
/*
* Unsigned divide: (16-bit quot), (16-bit rem) = (32-bit) / (16-bit)
*/
__value_in_regs extern _ARMABI_PURE __sdiv64by32 __rt_sdiv64by32(
int /*numer_h*/, unsigned int /*numer_l*/,
int /*denom*/);
/*
* Signed divide: (32-bit quot), (32-bit rem) = (64-bit) / (32-bit)
*/
#endif
/*
* ARM floating-point mask/status function (for both hardfp and softfp)
*/
extern _ARMABI unsigned int __fp_status(unsigned int /*mask*/, unsigned int /*flags*/);
/*
* mask and flags are bit-fields which correspond directly to the
* floating point status register in the FPE/FPA and fplib.
* __fp_status returns the current value of the status register,
* and also sets the writable bits of the word
* (the exception control and flag bytes) to:
*
* new = (old & ~mask) ^ flags;
*/
#define __fpsr_IXE 0x100000
#define __fpsr_UFE 0x80000
#define __fpsr_OFE 0x40000
#define __fpsr_DZE 0x20000
#define __fpsr_IOE 0x10000
#define __fpsr_IXC 0x10
#define __fpsr_UFC 0x8
#define __fpsr_OFC 0x4
#define __fpsr_DZC 0x2
#define __fpsr_IOC 0x1
/*
* Multibyte Character Functions.
* The behaviour of the multibyte character functions is affected by the
* LC_CTYPE category of the current locale. For a state-dependent encoding,
* each function is placed into its initial state by a call for which its
* character pointer argument, s, is a null pointer. Subsequent calls with s
* as other than a null pointer cause the internal state of the function to be
* altered as necessary. A call with s as a null pointer causes these functions
* to return a nonzero value if encodings have state dependency, and a zero
* otherwise. After the LC_CTYPE category is changed, the shift state of these
* functions is indeterminate.
*/
extern _ARMABI int mblen(const char * /*s*/, size_t /*n*/);
/*
* If s is not a null pointer, the mblen function determines the number of
* bytes compromising the multibyte character pointed to by s. Except that
* the shift state of the mbtowc function is not affected, it is equivalent
* to mbtowc((wchar_t *)0, s, n);
* Returns: If s is a null pointer, the mblen function returns a nonzero or
* zero value, if multibyte character encodings, respectively, do
* or do not have state-dependent encodings. If s is not a null
* pointer, the mblen function either returns a 0 (if s points to a
* null character), or returns the number of bytes that compromise
* the multibyte character (if the next n of fewer bytes form a
* valid multibyte character), or returns -1 (they do not form a
* valid multibyte character).
*/
extern _ARMABI int mbtowc(wchar_t * __restrict /*pwc*/,
const char * __restrict /*s*/, size_t /*n*/);
/*
* If s is not a null pointer, the mbtowc function determines the number of
* bytes that compromise the multibyte character pointed to by s. It then
* determines the code for value of type wchar_t that corresponds to that
* multibyte character. (The value of the code corresponding to the null
* character is zero). If the multibyte character is valid and pwc is not a
* null pointer, the mbtowc function stores the code in the object pointed
* to by pwc. At most n bytes of the array pointed to by s will be examined.
* Returns: If s is a null pointer, the mbtowc function returns a nonzero or
* zero value, if multibyte character encodings, respectively, do
* or do not have state-dependent encodings. If s is not a null
* pointer, the mbtowc function either returns a 0 (if s points to
* a null character), or returns the number of bytes that
* compromise the converted multibyte character (if the next n of
* fewer bytes form a valid multibyte character), or returns -1
* (they do not form a valid multibyte character).
*/
extern _ARMABI int wctomb(char * /*s*/, wchar_t /*wchar*/);
/*
* determines the number of bytes need to represent the multibyte character
* corresponding to the code whose value is wchar (including any change in
* shift state). It stores the multibyte character representation in the
* array object pointed to by s (if s is not a null pointer). At most
* MB_CUR_MAX characters are stored. If the value of wchar is zero, the
* wctomb function is left in the initial shift state).
* Returns: If s is a null pointer, the wctomb function returns a nonzero or
* zero value, if multibyte character encodings, respectively, do
* or do not have state-dependent encodings. If s is not a null
* pointer, the wctomb function returns a -1 if the value of wchar
* does not correspond to a valid multibyte character, or returns
* the number of bytes that compromise the multibyte character
* corresponding to the value of wchar.
*/
/*
* Multibyte String Functions.
* The behaviour of the multibyte string functions is affected by the LC_CTYPE
* category of the current locale.
*/
extern _ARMABI size_t mbstowcs(wchar_t * __restrict /*pwcs*/,
const char * __restrict /*s*/, size_t /*n*/) __attribute__((__nonnull__(2)));
/*
* converts a sequence of multibyte character that begins in the initial
* shift state from the array pointed to by s into a sequence of
* corresponding codes and stores not more than n codes into the array
* pointed to by pwcs. No multibyte character that follow a null character
* (which is converted into a code with value zero) will be examined or
* converted. Each multibyte character is converted as if by a call to
* mbtowc function, except that the shift state of the mbtowc function is
* not affected. No more than n elements will be modified in the array
* pointed to by pwcs. If copying takes place between objects that overlap,
* the behaviour is undefined.
* Returns: If an invalid multibyte character is encountered, the mbstowcs
* function returns (size_t)-1. Otherwise, the mbstowcs function
* returns the number of array elements modified, not including
* a terminating zero code, if any.
*/
extern _ARMABI size_t wcstombs(char * __restrict /*s*/,
const wchar_t * __restrict /*pwcs*/, size_t /*n*/) __attribute__((__nonnull__(2)));
/*
* converts a sequence of codes that correspond to multibyte characters
* from the array pointed to by pwcs into a sequence of multibyte
* characters that begins in the initial shift state and stores these
* multibyte characters into the array pointed to by s, stopping if a
* multibyte character would exceed the limit of n total bytes or if a
* null character is stored. Each code is converted as if by a call to the
* wctomb function, except that the shift state of the wctomb function is
* not affected. No more than n elements will be modified in the array
* pointed to by s. If copying takes place between objects that overlap,
* the behaviour is undefined.
* Returns: If a code is encountered that does not correspond to a valid
* multibyte character, the wcstombs function returns (size_t)-1.
* Otherwise, the wcstombs function returns the number of bytes
* modified, not including a terminating null character, if any.
*/
extern _ARMABI void __use_realtime_heap(void);
extern _ARMABI void __use_realtime_division(void);
extern _ARMABI void __use_two_region_memory(void);
extern _ARMABI void __use_no_heap(void);
extern _ARMABI void __use_no_heap_region(void);
extern _ARMABI char const *__C_library_version_string(void);
extern _ARMABI int __C_library_version_number(void);
#ifdef __cplusplus
} /* extern "C" */
} /* namespace std */
#endif /* __cplusplus */
#endif /* __STDLIB_DECLS */
#if _AEABI_PORTABILITY_LEVEL != 0 && !defined _AEABI_PORTABLE
#define _AEABI_PORTABLE
#endif
#ifdef __cplusplus
#ifndef __STDLIB_NO_EXPORTS
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
using ::std::atoll;
using ::std::lldiv_t;
#endif /* !defined(__STRICT_ANSI__) || __USE_C99_STDLIB */
using ::std::div_t;
using ::std::ldiv_t;
using ::std::atof;
using ::std::atoi;
using ::std::atol;
using ::std::strtod;
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
using ::std::strtof;
using ::std::strtold;
#endif
using ::std::strtol;
using ::std::strtoul;
using ::std::strtoll;
using ::std::strtoull;
using ::std::rand;
using ::std::srand;
using ::std::_rand_state;
using ::std::_rand_r;
using ::std::_srand_r;
using ::std::_ANSI_rand_state;
using ::std::_ANSI_rand_r;
using ::std::_ANSI_srand_r;
using ::std::calloc;
using ::std::free;
using ::std::malloc;
using ::std::realloc;
#if !defined(__STRICT_ANSI__)
using ::std::posix_memalign;
#endif
using ::std::__heapprt;
using ::std::__heapstats;
using ::std::__heapvalid;
using ::std::abort;
using ::std::atexit;
using ::std::exit;
using ::std::_Exit;
using ::std::getenv;
using ::std::system;
using ::std::bsearch;
using ::std::qsort;
using ::std::abs;
using ::std::div;
using ::std::labs;
using ::std::ldiv;
#if !defined(__STRICT_ANSI__) || __USE_C99_STDLIB
using ::std::llabs;
using ::std::lldiv;
#endif /* !defined(__STRICT_ANSI__) || __USE_C99_STDLIB */
#if !(__ARM_NO_DEPRECATED_FUNCTIONS)
using ::std::__sdiv32by16;
using ::std::__udiv32by16;
using ::std::__sdiv64by32;
using ::std::__rt_sdiv32by16;
using ::std::__rt_udiv32by16;
using ::std::__rt_sdiv64by32;
#endif
using ::std::__fp_status;
using ::std::mblen;
using ::std::mbtowc;
using ::std::wctomb;
using ::std::mbstowcs;
using ::std::wcstombs;
using ::std::__use_realtime_heap;
using ::std::__use_realtime_division;
using ::std::__use_two_region_memory;
using ::std::__use_no_heap;
using ::std::__use_no_heap_region;
using ::std::__C_library_version_string;
using ::std::__C_library_version_number;
using ::std::size_t;
using ::std::__aeabi_MB_CUR_MAX;
#endif /* __STDLIB_NO_EXPORTS */
#endif /* __cplusplus */
#undef __LONGLONG
#endif /* __stdlib_h */
/* end of stdlib.h */