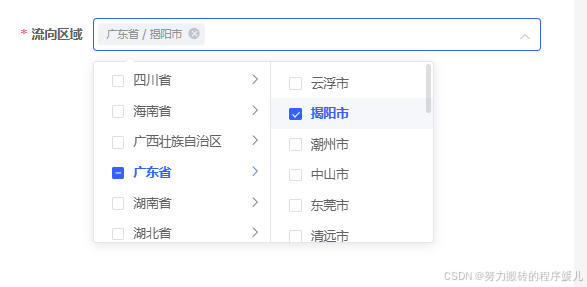
<template>
<div v-if="visible" ref="pageTotal" class="page-total" style="overflow-y: auto">
<div ref="pageHead" class="page-head">
<el-container>
<el-main>
<el-form
ref="dataForm"
:model="dataForm"
:rules="dataRule"
label-width="auto"
@keyup.enter.native="dataFormSubmit()"
>
<div class="title padding-top-df">
<div class="left" />
<span>创建统计报表</span>
</div>
<el-row>
<el-col :span="8">
<el-form-item label="统计报表名称" prop="reportName">
<el-input v-model="dataForm.reportName" :disabled="watchEdit == 'watch'" />
</el-form-item>
</el-col>
<el-col :span="8">
<el-form-item label="业务类型" prop="businessType">
<el-select v-model="dataForm.businessType" placeholder="请选择" clearable :disabled="watchEdit == 'watch'" multiple>
<el-option
v-for="item in businessTypeList"
:key="item.dictCode"
:label="item.dictName"
:value="item.dictCode"
/>
</el-select>
</el-form-item>
</el-col>
<el-col :span="8">
<el-form-item label="制造基地" prop="manufactureBase">
<el-select v-model="dataForm.manufactureBase" placeholder="请选择" filterable clearable :disabled="watchEdit == 'watch'" multiple>
<el-option
v-for="item in manufacturingSiteList"
:key="item"
:label="item"
:value="item"
/>
</el-select>
</el-form-item>
</el-col>
<el-col :span="8">
<el-form-item label="运输方式" prop="transportType">
<el-select v-model="dataForm.transportType" placeholder="请选择" clearable :disabled="watchEdit == 'watch'" multiple>
<el-option
v-for="item in transportList"
:key="item.dictName"
:label="item.dictName"
:value="item.dictName"
/>
</el-select>
</el-form-item>
</el-col>
<el-col :span="8">
<el-form-item label="产品大类" prop="productCategory">
<el-select v-model="dataForm.productCategory" filterable multiple placeholder="请选择" :disabled="watchEdit == 'watch'">
<el-option
v-for="item in productCategoryList"
:key="item"
:label="item"
:value="item"
/>
</el-select>
</el-form-item>
</el-col>
<el-col :span="8">
<el-form-item label="流向区域" prop="direction">
<el-cascader
ref="endCityArr"
v-model="dataForm.direction"
:props="cityProps"
style="width: 100%;"
collapse-tags
collapse-tags-tooltip
:disabled="watchEdit == 'watch'"
clearable
@change="handleChangeM"
/>
</el-form-item>
</el-col>
</el-row>
</el-form>
</el-main>
</el-container>
<div class="footer-bottom">
<span slot="footer" class="footer">
<el-button @click="cancel">取 消</el-button>
<el-button v-if="watchEdit != 'watch'" type="primary" :loading="addLoading" @click="dataFormSubmit()">提 交
</el-button>
</span>
</div>
</div>
</div>
</template>
<script>
import addressApi from '@/api/logisticsbase/common/address/address'
import saleOrderFulfillment from '@/api/logisticsplan/salesOrderFulfillment/saleOrderFulfillment'
import Api from '@/api/logisticsplan/salesOrderFulfillment/saleOrderFulfillment'
import DictApi from '@/api/dict'
import MaterialBase from '@/api/logisticsbase/material/materialBase'
export default {
name: 'SalesOrderFulfillmentAdd',
components: {
},
props: {
watchEdit: {
type: String,
default: 'edit'
}
},
data() {
return {
isError: '',
visible: false,
dataForm: {
id: '',
reportName: '',
businessType: '',
manufactureBase: '',
transportType: '',
productCategory: '',
direction: []
},
dataRule: {
reportName: [
{ required: true, message: '统计报表名称不能为空', trigger: 'blur' }
],
businessType: [
{ required: true, message: '业务类型不能为空', trigger: 'blur' }
],
manufactureBase: [
{ required: true, message: '制造基地不能为空', trigger: 'blur' }
],
transportType: [
{ required: true, message: '运输方式不能为空', trigger: 'blur' }
],
productCategory: [
{ required: true, message: '产品大类不能为空', trigger: 'blur' }
],
direction: [
{ required: true, message: '流向区域不能为空', trigger: 'blur' }
]
},
transportList: [],
manufacturingSiteList: [],
productCategoryList: [],
businessTypeList: [
{
dictName: '销售配送',
dictCode: '一票制'
}, {
dictName: '销售自提',
dictCode: '需方自付'
}
],
addLoading: false,
cityProps: {
multiple: true,
lazy: true,
// emitPath: true,
checkStrictly: false,
// checkStrictly: false,
lazyLoad(node, resolve) {
const level = node.level
if (level === 0) {
// 省
addressApi.getAddressList({ parentCode: 'CN' }).then((res) => {
const list = res.data
list.map(item => {
item.value = item.name
item.label = item.name
item.leaf = false
})
resolve(list)
})
} else if (level === 1) {
// 市
addressApi.getAddressList({ parentCode: node.data.code }).then((res) => {
const list = res.data
list.map(item => {
item.value = item.name
item.label = item.name
item.leaf = true
})
resolve(list)
})
} else {
resolve([])
}
// else if (level === 2) {
// 区县
// resolve([])
// addressApi.getAddressList({ parentCode: node.data.code }).then((res) => {
// const list = res.data
// list.map(item => {
// item.value = item.name
// item.label = item.name
// item.leaf = true
// })
// resolve(list)
// })
// }
}
}
}
},
methods: {
queryProductCategoryList() {
Api.queryProductCategoryList().then((res) => {
this.productCategoryList = res.data
})
},
initOptions() {
DictApi.fetchList('programme_transport_name').then((res) => {
this.transportList = res.data
})
},
queryManufacturingSiteList() {
Api.queryManufacturingSiteList().then((res) => {
this.manufacturingSiteList = res.data
})
},
init(id) {
this.dataForm.id = id || ''
this.visible = true
this.queryManufacturingSiteList()
this.queryProductCategoryList()
this.initOptions()
this.$nextTick(() => {
if (id) {
saleOrderFulfillment.fetchDetailById({
id: id
}).then((res) => {
const data = res.data
if (res && res.success) {
this.dataForm = data
// 把以,分割的字符转转成数组
this.dataForm.direction = data.directionArea.split(',')
for (let i = 0; i < this.dataForm.direction.length; i++) {
this.dataForm.direction[i] = this.dataForm.direction[i].split('/')
}
this.dataForm.productCategory = data.productCategory.split(',')
this.dataForm.transportType = data.transportType.split(',')
this.dataForm.manufactureBase = data.manufactureBase.split(',')
this.dataForm.businessType = data.businessType.split(',')
}
})
}
})
},
// 表单提交
dataFormSubmit() {
this.addLoading = true
this.$refs['dataForm'].validate((valid) => {
if (valid) {
console.log(this.dataForm)
this.addLoading = false
for (let i = 0; i < this.dataForm.direction.length; i++) {
this.dataForm.direction[i] = this.dataForm.direction[i].join('/')
}
// 把数据转成以,分割的字段
this.dataForm.directionArea = this.dataForm.direction.join(',')
this.dataForm.productCategory = this.dataForm.productCategory.join(',')
this.dataForm.transportType = this.dataForm.transportType.join(',')
this.dataForm.manufactureBase = this.dataForm.manufactureBase.join(',')
this.dataForm.businessType = this.dataForm.businessType.join(',')
console.log(this.dataForm)
saleOrderFulfillment.add(this.dataForm).then((res) => {
if (res && res.success) {
this.$message({
message: '操作成功',
type: 'success',
duration: 500,
onClose: () => {
this.visible = false
this.$emit('refreshDataList')
this.$emit('changeVisible')
}
})
} else {
this.$message.error(res.message)
}
this.addLoading = false
}).catch((e) => {
this.addLoading = false
})
} else {
this.addLoading = false
}
})
},
cancel() { // 取消
this.visible = false
this.$emit('changeVisible')
this.$emit('refreshDataList')
this.$refs['dataForm'].resetFields()
},
// 禁用标识初始化
changeDisable() {
this.options.forEach((e) => {
e.disabled = false
// 此处也可使用递归处理
if (e.hasOwnProperty('children')) {
e.children.forEach((h) => {
h.disabled = false
if (h.hasOwnProperty('children')) {
h.children.forEach((k) => {
k.disabled = false
})
}
})
}
})
},
// 改变级联选项时触发的事件
handleChangeM(value) {
this.changeDisable()
// 需将选中的下一级禁选,否则展示的下一级仍会存在
value.forEach((e) => {
// 省级选中,市区禁用
// if (e.length === 1) {
// this.options.forEach((h) => {
// if (h.hasOwnProperty('children') && h.value === e[0]) {
// h.children.forEach((j) => {
// j.disabled = true
// if (j.hasOwnProperty('children')) {
// j.children.forEach((k) => {
// k.disabled = true
// })
// }
// })
// }
// })
// }
// 市级选中,区级禁用
// if (e.length === 2) {
// this.options.forEach((h) => {
// if (h.hasOwnProperty('children') && h.value === e[0]) {
// h.children.forEach((j) => {
// if (j.hasOwnProperty('children') && j.value === e[1]) {
// j.children.forEach((k) => {
// k.disabled = true
// })
// }
// })
// }
// })
// }
})
// 省覆盖市,市覆盖区(重点在于处理级联组件上绑定的value值)
for (let i = 0; i < value.length - 1; i++) {
// if (value[i].length === 1) {
// for (let j = 0; j < value.length; j++) {
// if (value[j].length > 1 && value[i][0] === value[j][0]) {
// delete value[j]// 使用delete并不会移除下标而是将该项设置为undefined,可以避免数组下标变化引起的遍历数组的问题
// }
// }
// }
// if (value[i].length === 2) {
// for (let j = 0; j < value.length; j++) {
// if (value[j].length > 2 && value[i][0] === value[j][0] && value[i][1] === value[j][1]) {
// delete value[j]
// }
// }
// }
}
this.$refs.form.validateField('regions', (valid) => {
this.isError = valid
})
}
}
}
</script>
<style lang="less" scoped>
@import '../../../../../styles/page.less';
.title {
width: 100%;
height: 40px;
display: flex;
}
.left {
width: 4px;
height: 20px;
background-color: #1890ff;
margin-right: 10px;
}
.kuang-input {
width: 100%;
}
.el-date-editor.el-input {
width: 100%;
}
.common-upload {
margin-bottom: 20px;
}
.table-coln {
width: 100%;
height: 35px;
.del {
float: right;
width: auto;
color: red;
cursor: pointer;
}
.copy {
float: right;
width: auto;
color: #1890ff;
margin-right: 20px;
cursor: pointer;
}
}
.page-total {
padding: 0px !important;
}
.table-colmn {
width: 100%;
height: 35px;
.del {
float: right;
width: auto;
color: red;
cursor: pointer;
}
.other {
float: right;
width: auto;
color: #1890ff;
margin-right: 20px;
cursor: pointer;
}
}
.tableColomStyle {
margin-bottom: 0px !important;
}
.padding-top-df {
padding-top: 10px;
}
</style>
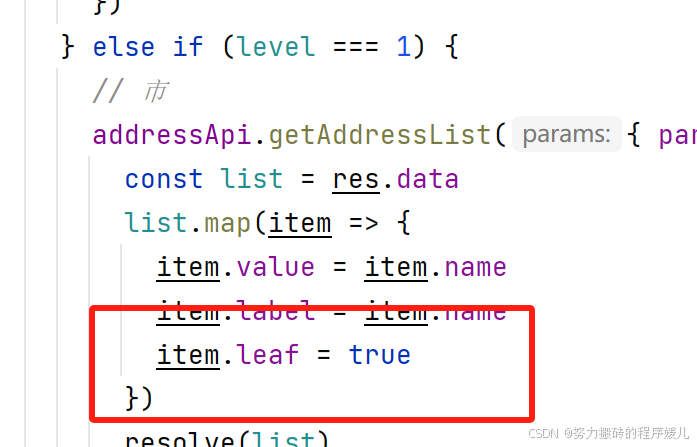