
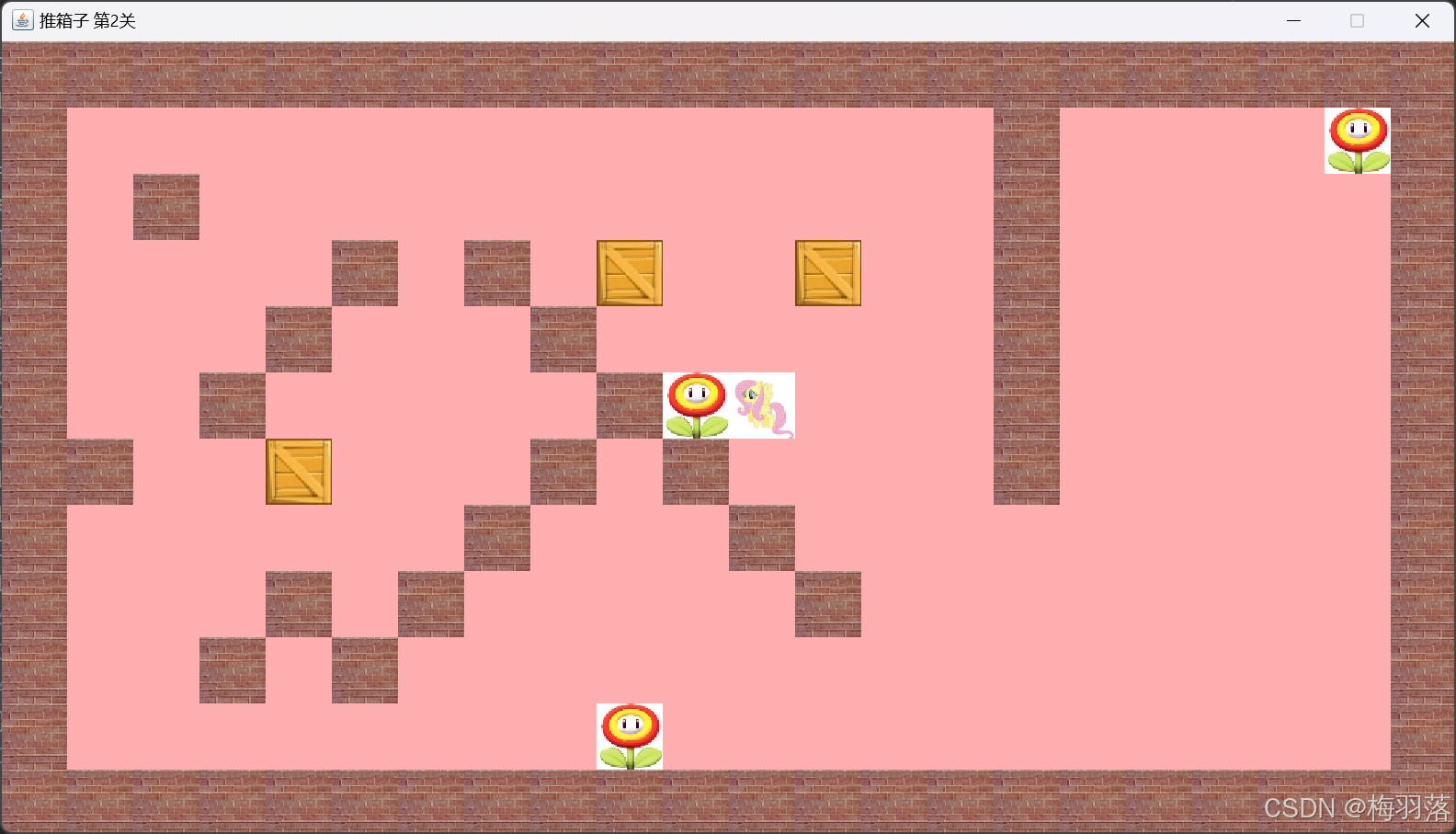
package com.meilingluo.TXZ_3;
import javax.swing.*;
import java.awt.*;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class GameFrame extends JFrame {
private JPanel panel;
private JLabel worker;
private JLabel[] boxes;
private JLabel[] goals;
private JLabel[] walls;
private String[] workerImgPaths;
private int index = 1;//默认第一关
public GameFrame() {
super.setSize(22 * 48 + 12, 12 * 48 + 35);
super.setResizable(false);//大小不可改
super.setLocationRelativeTo(null);//居中
super.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);//关闭时结束进程
initPanel();
addEvent();
}
private void addEvent() {
addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
int x = 0, y = 0;
String imgPath = workerImgPaths[0];
if (keyCode == KeyEvent.VK_A || keyCode == KeyEvent.VK_LEFT) {
x = -48;
} else if (keyCode == KeyEvent.VK_W || keyCode == KeyEvent.VK_UP) {
y = -48;
imgPath = workerImgPaths[1];
} else if (keyCode == KeyEvent.VK_S || keyCode == KeyEvent.VK_DOWN) {
y = 48;
imgPath = workerImgPaths[2];
} else if (keyCode == KeyEvent.VK_D || keyCode == KeyEvent.VK_RIGHT) {
x = 48;
imgPath = workerImgPaths[3];
}
//让工人移动
worker.setBounds(worker.getBounds().x + x, worker.getBounds().y + y, 48, 48);
ImageIcon img = new ImageIcon("imgs/" + imgPath);
worker.setIcon(img);
//防止撞墙
for (int i = 0; i < walls.length; i++) {
//如果位置重合
if (worker.getBounds().contains(walls[i].getBounds())) {
worker.setBounds(worker.getBounds().x - x, worker.getBounds().y - y, 48, 48);
break;
}
}
//柔柔推箱子
int moveIndex = -1;//记录移动的箱子的下标
for (int i = 0; i < boxes.length; i++) {
if (worker.getBounds().contains(boxes[i].getBounds())) {
boxes[i].setBounds(boxes[i].getBounds().x + x, boxes[i].getBounds().y + y, 48, 48);
moveIndex = i;
break;
}
}
//箱子不重合
if (moveIndex > -1) {
//箱子移动了
for (int i = 0; i < boxes.length; i++) {
if (i == moveIndex) continue;
if (boxes[moveIndex].getBounds().contains(boxes[i].getBounds())) {
boxes[i].setBounds(boxes[i].getBounds().x - x, boxes[i].getBounds().y - y, 48, 48);
worker.setBounds(worker.getBounds().x - x, worker.getBounds().y - y, 48, 48);
break;
}
}
}
// for(int i=0;i<boxes.length;i++){
// for (int j=0;j<boxes.length;j++){
// if(i == j)continue;
// if(boxes[i].getBounds().contains(boxes[j].getBounds())){
// boxes[i].setBounds(boxes[i].getBounds().x-x,boxes[i].getBounds().y-y,48,48);
// worker.setBounds(worker.getBounds().x-x,worker.getBounds().y-y,48,48);
// i=boxes.length;//可有可无
// break;
// }
// }
// }
for (int i = 0; i < boxes.length; i++) {//箱子是否穿墙
for (int j = 0; j < walls.length; j++) {
if (boxes[i].getBounds().contains(walls[j].getBounds())) {
boxes[i].setBounds(boxes[i].getBounds().x - x, boxes[i].getBounds().y - y, 48, 48);
worker.setBounds(worker.getBounds().x - x, worker.getBounds().y - y, 48, 48);
break;
}
}
}
//判断输赢
int containsCount = 0;
for (int i = 0;i < boxes.length;i++){
for(int j = 0;j<goals.length;j++){
if(boxes[i].getBounds().contains(goals[j].getBounds())){
containsCount++;
break;
}
}
}
if(containsCount == boxes.length){//全部重合
LevelData data = LevelDataManager.getNowLevelData(index+1);
if(data == null){
JOptionPane.showMessageDialog(null,"通关了");
System.exit(0);
}else {
String msg = "第" + index + "关通关,是否玩下一关 "+data.getTitle();
int a = JOptionPane.showConfirmDialog(null,msg,"请确认",JOptionPane.YES_NO_OPTION,JOptionPane.NO_OPTION);
if(a == JOptionPane.YES_NO_OPTION){
index++;
loadLevelData(data);
}else if(a == JOptionPane.NO_OPTION){
JOptionPane.showMessageDialog(null,"拜拜");
System.exit(0);
}
}
//JOptionPane.showMessageDialog(null,"赢了");
}
}
@Override
public void keyReleased(KeyEvent e) {
}
});
}
public void loadLevelData(LevelData data) {
panel.removeAll();
super.setTitle("推箱子 " + data.getTitle());
panel.setBackground(data.getBgColor());
this.workerImgPaths = data.getWorkerImgPath();
worker = initLabel(this.workerImgPaths[0], data.getWorkerLocation());
// int[][]boxLocations = data.getBoxLocation();
// boxes = new JLabel[boxLocations.length]; //箱子的个数就是二维数组的行数
// for(int i=0;i<boxes.length;i++){
// boxes[i] = initLabel(data.getBoxImgPath(),boxLocations[i]);
// }
boxes = initLabels(data.getBoxImgPath(), data.getBoxLocation());//效果同前5行
// int[][] goalLocation = data.getGoalLocation();
// goals = new JLabel[goalLocation.length];
// for(int i=0;i<goals.length;i++){
// goals[i] = initLabel(data.getGoalImgPath(),goalLocation[i]);
// }
goals = initLabels(data.getGoalImgPath(), data.getGoalLocation());//效果同前5行
initWalls(data.getWallImgPath(), data.getWallLocation());
panel.repaint();
}
private void initWalls(String wallImgPath, int[][] wallLocations) {
ImageIcon wallImg = new ImageIcon("imgs/" + wallImgPath);
this.walls = new JLabel[22 * 2 + 10 * 2 + wallLocations.length];
for (int i = 0; i < walls.length; i++) {
walls[i] = new JLabel(wallImg);
}
int index = 0;
//铺上下墙
for (int i = 0; i < 22; i++) {
panel.add(walls[index]);
walls[index++].setBounds(i * 48, 0, 48, 48);
panel.add(walls[index]);
walls[index++].setBounds(i * 48, 11 * 48, 48, 48);
}
//铺左右墙
for (int i = 1; i <= 10; i++) {
panel.add(walls[index]);
walls[index++].setBounds(0, i * 48, 48, 48);
panel.add(walls[index]);
walls[index++].setBounds(21 * 48, i * 48, 48, 48);
}
//添加障碍物
for (int i = 0; i < wallLocations.length; i++) {
int[] wallLocation = wallLocations[i];
panel.add(walls[index]);
walls[index++].setBounds(wallLocation[0] * 48, wallLocation[1] * 48, 48, 48);
}
}
private void initPanel() {
this.panel = new JPanel();
panel.setLayout(null);
super.setContentPane(panel);
}
private JLabel initLabel(String imgPath, int[] location) {//把图片放入面板
ImageIcon img = new ImageIcon("imgs/" + imgPath);//创建图片对象
JLabel label = new JLabel(img);//创建标签对象并利用构造方法传参标签中显示图片
panel.add(label);//标签加入面板
label.setBounds(location[0] * 48, location[1] * 48, 48, 48);//设置标签在面板中的位置与大小
return label;//返回加入的标签
}
private JLabel[] initLabels(String imgPath, int[][] locations) {
ImageIcon img = new ImageIcon("imgs/" + imgPath);
JLabel[] labels = new JLabel[locations.length];
for (int i = 0; i < labels.length; i++) {
labels[i] = new JLabel(img);
panel.add(labels[i]);
labels[i].setBounds(locations[i][0] * 48, locations[i][1] * 48, 48, 48);
}
return labels;
}
}
package com.meilingluo.TXZ_3;
import javax.swing.*;
import java.awt.*;
public class LevelData {
private String title;//关卡标题
private Color bgColor;//关卡颜色
private int[] workerLocation;
private String[] workerImgPath;
private int[][] boxLocation;
private String boxImgPath;
private int [][] goalLocation;
private String goalImgPath;
private int [][] wallLocation;
private String wallImgPath;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Color getBgColor() {
return bgColor;
}
public void setBgColor(Color bgColor) {
this.bgColor = bgColor;
}
public int[] getWorkerLocation() {
return workerLocation;
}
public void setWorkerLocation(int[] workerLocation) {
this.workerLocation = workerLocation;
}
public String[] getWorkerImgPath() {
return workerImgPath;
}
public void setWorkerImgPath(String[] workerImgPath) {
this.workerImgPath = workerImgPath;
}
public int[][] getBoxLocation() {
return boxLocation;
}
public void setBoxLocation(int[][] boxLocation) {
this.boxLocation = boxLocation;
}
public String getBoxImgPath() {
return boxImgPath;
}
public void setBoxImgPath(String boxImgPath) {
this.boxImgPath = boxImgPath;
}
public int[][] getGoalLocation() {
return goalLocation;
}
public void setGoalLocation(int[][] goalLocation) {
this.goalLocation = goalLocation;
}
public String getGoalImgPath() {
return goalImgPath;
}
public void setGoalImgPath(String goalImgPath) {
this.goalImgPath = goalImgPath;
}
public int[][] getWallLocation() {
return wallLocation;
}
public void setWallLocation(int[][] wallLocation) {
this.wallLocation = wallLocation;
}
public String getWallImgPath() {
return wallImgPath;
}
public void setWallImgPath(String wallImgPath) {
this.wallImgPath = wallImgPath;
}
}
package com.meilingluo.TXZ_3;
import java.awt.*;
public class LevelDataManager {
public static LevelData getNowLevelData(int index){
LevelData data = new LevelData();
if(index == 1){
data.setTitle("第一关");
data.setBgColor(Color.pink);
data.setWorkerLocation(new int[]{11,5});
String[] workerImgPaths= {"workerLeft4.png","workerUp4.png","workerDown4.png","workerRight4.png"};
data.setWorkerImgPath(workerImgPaths);
int[][] boxLocation = {{16, 2},{7, 9}, {9, 8}};
data.setBoxLocation(boxLocation);
data.setBoxImgPath("box3.png");
int[][] goalLocation = {{8, 2}, {3, 9}, {16, 6}};
data.setGoalLocation(goalLocation);
data.setGoalImgPath("goal2.png");
int[][] wallLocation = {
{3, 7}, {4, 6}, {5, 5},{6, 4},
{7, 3},
{8, 4}, {9, 5}, {10, 6}, {11, 7},{12,8},
{5,9},{6,8},{7,7},{8,6},
{15,1},{15,2},{15, 3}, {15, 4}, {15, 5},{15, 6}
};
data.setWallImgPath("wall2.png");
data.setWallLocation(wallLocation);
}else if(index == 2){
data.setTitle("第2关");
data.setBgColor(Color.pink);
data.setWorkerLocation(new int[]{11,5});
String[] workerImgPaths= {"workerLeft4.png","workerUp4.png","workerDown4.png","workerRight4.png"};
data.setWorkerImgPath(workerImgPaths);
int[][] boxLocation = {{4, 6},{9, 3}, {12, 3}};
data.setBoxLocation(boxLocation);
data.setBoxImgPath("box3.png");
int[][] goalLocation = {{9, 10}, {10, 5}, {20, 1}};
data.setGoalLocation(goalLocation);
data.setGoalImgPath("goal2.png");
int[][] wallLocation = {
{1, 6}, {2, 2}, {3, 5},{3, 9},{4,4},{4,8},{5,3},
{7, 3},
{8, 4}, {9, 5}, {10, 6}, {11, 7},{12,8},
{5,9},{6,8},{7,7},{8,6},
{15,1},{15,2},{15, 3}, {15, 4}, {15, 5},{15, 6}
};
data.setWallImgPath("wall.png");
data.setWallLocation(wallLocation);
} else {
return null;
}
return data;
}
}
package com.meilingluo.TXZ_3;
public class Main {
public static void main(String[] args) {
GameFrame gameFrame = new GameFrame();
LevelData data = LevelDataManager.getNowLevelData(2);
gameFrame.loadLevelData(data);
gameFrame.setVisible(true);
}
}
