demo
@PostMapping("myUploadExcelEntityTest")
public String myUploadExcelEntityTest(@RequestParam("fileName")MultipartFile fileName) throws Exception {
if (fileName.isEmpty()) {
return "文件为空,请重新上传";
}
if (!fileName.getOriginalFilename().endsWith(".xls")&&!fileName.getOriginalFilename().endsWith(".xlsx")) {
return "上传文件不是excel类型";
}
InputStream fis = new BufferedInputStream(fileName.getInputStream());
Workbook workbook = WorkbookFactory.create(fis);
List<Student> studentList = readExcel(Student.class, workbook);
List<String> pic = getPic(workbook);
for (int i=0;i<studentList.size();i++){
studentList.get(i).setPicpath(pic.get(i));
System.out.println(studentList.get(i));
}
return "good";
}
public List<String> getPic(Workbook workbook) throws IOException {
List<? extends PictureData> allPictures = workbook.getAllPictures();
List<String> picPath = new ArrayList<>();
for (PictureData pic:allPictures ) {
String ext = pic.suggestFileExtension();
byte[] data = pic.getData();
String path = "D:/tmpCanDel/"+new Date().getTime() + "." + ext;
File file = new File(path);
FileOutputStream out = new FileOutputStream(file);
picPath.add(path);
out.write(data);
out.close();
}
return picPath;
}
private static <T> List<T> readExcel(Class<T> classzz,Workbook workbook) throws Exception {
Sheet sheet = workbook.getSheetAt(0);
int lastRowNum = sheet.getLastRowNum()+1;
int lastCellNum = sheet.getRow(0).getLastCellNum();
Field[] fields = classzz.getDeclaredFields();
Row row = null;
List<String> headList = new ArrayList<>();
List<T> beans = new ArrayList<T>();
for (int j=0;j<lastCellNum;j++){
row = sheet.getRow(0);
Cell cell = row.getCell(j);
DataFormatter formatter = new DataFormatter();
String value = formatter.formatCellValue(cell);
headList.add(value);
}
for (int i=1;i<lastRowNum;i++){
T instance = classzz.newInstance();
for (int j=0;j<lastCellNum;j++){
row = sheet.getRow(i);
Cell cell = row.getCell(j);
DataFormatter formatter = new DataFormatter();
String value = formatter.formatCellValue(cell);
String headName = headList.get(j);
for (Field field : fields){
if (headName.equalsIgnoreCase(field.getName())){
String methodName = MethodUtils.setMethodName(field.getName());
Method method = classzz.getMethod(methodName,field.getType());
method.invoke(instance,value);
}
}
}
beans.add(instance);
}
return beans;
}
static class MethodUtils {
private static final String SET_PREFIX = "set";
private static final String GET_PREFIX = "get";
private static String capitalize(String name) {
if (name == null || name.length() == 0) {
return name;
}
return name.substring(0, 1).toUpperCase() + name.substring(1);
}
public static String setMethodName(String propertyName) {
return SET_PREFIX + capitalize(propertyName);
}
public static String getMethodName(String propertyName) {
return GET_PREFIX + capitalize(propertyName);
}
}
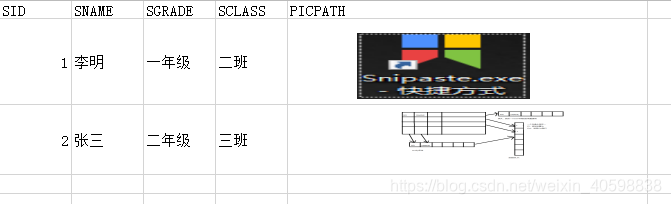

pom文件
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.9</version>
</dependency>