PCM编码
编码过程:符号位,段落码,段内码。符号位简单,段内码转为bin就可以
段内码下图就自己看看就好
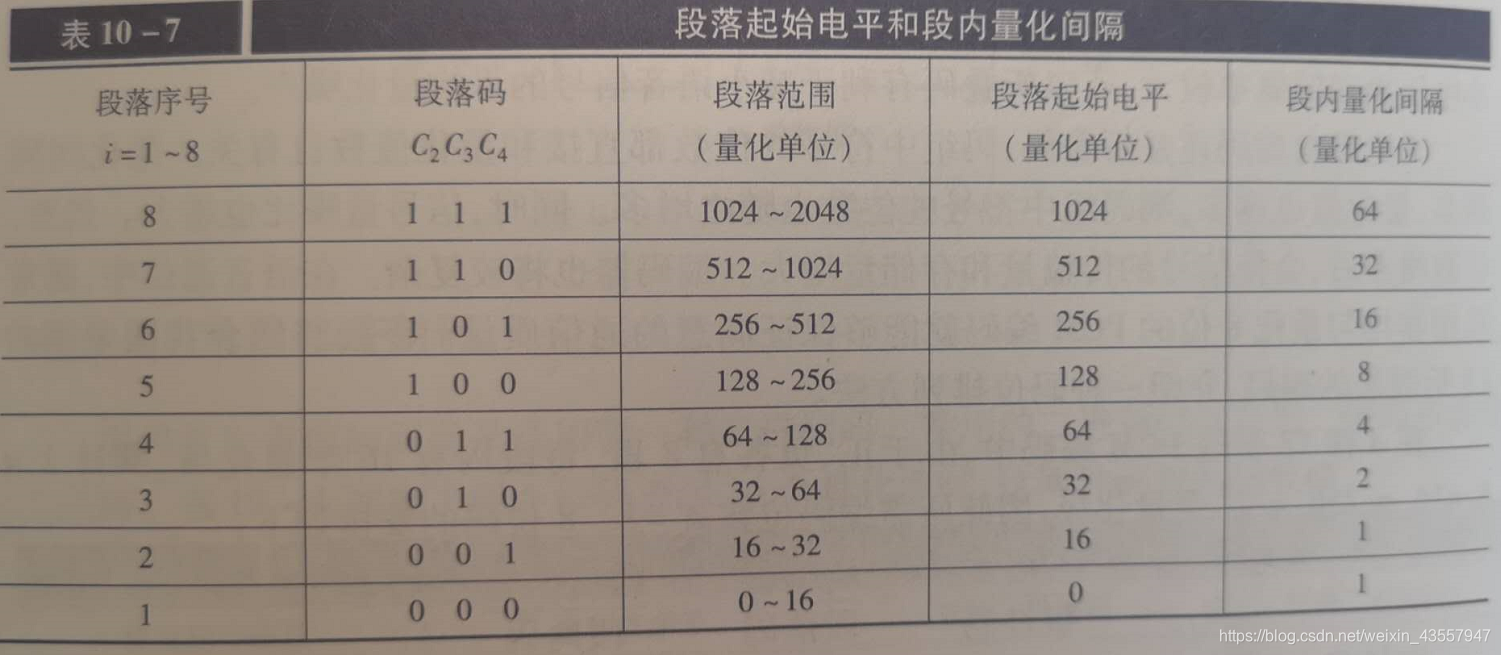
import numpy as np
def PCM_encode(x):
#输入全部得是int项的list,没有做错误处理
x = list(x)
n = len(x)
for i in range(n):
try:
x[i]=int(x[i])
except:
print("请输入数字")
print(type(x[i]),"格式错误")
return 0
out = np.zeros((n, 8))
#建立输出
for i in range(n):
# 符号位
if x[i] > 0:
out[i, 0] = 1
else:
out[i, 0] = 0
#段落位
if abs(x[i]) < 16:
out[i, 1], out[i, 2], out[i, 3], step, home = 0, 0, 0, 1, 0
elif abs(x[i]) < 32:
out[i, 1], out[i, 2], out[i, 3], step, home = 0, 0, 1, 1, 16
elif abs(x[i]) < 64:
out[i, 1], out[i, 2], out[i, 3], step, home = 0, 1, 0, 2, 32
elif abs(x[i]) < 128:
out[i, 1], out[i, 2], out[i, 3], step, home = 0, 1, 1, 4, 64
elif abs(x[i]) < 256:
out[i, 1], out[i, 2], out[i, 3], step, home = 1, 0, 0, 8, 128
elif abs(x[i]) < 512:
out[i, 1], out[i, 2], out[i, 3], step, home = 1, 0, 1, 16, 256
elif abs(x[i]) < 1024:
out[i, 1], out[i, 2], out[i, 3], step, home = 1, 1, 0, 32, 512
else:
out[i, 1], out[i, 2], out[i, 3], step, home = 1, 1, 1, 64, 1024
#段内码
sub = int(abs(x[i]) - home)
bin_str = bin( int( sub / step) )[2:]
res = [0,0,0,0]
#格外重要,防止在编码过程中由于长度不够导致的-1错误
for now in bin_str:
res.append(now)
mylen=len(res)
out[i, 4], out[i, 5], out[i, 6],out[i, 7]=int(res[mylen-4]),int(res[mylen-3]),int(res[mylen-2]),int(res[mylen-1])
#print(step, '****', sub,"***",res,'****',out[i, 4], out[i, 5], out[i, 6],out[i, 7])
#检验是否数据位出错
print(type(x),type(out))
print(out)
return out
PCM译码
import numpy as np
#解码过程
def PCM_decode(ins):
n=len(ins)
out = np.zeros((n, 1))
for i in range(n):
#符号位判断
if ins[i][0] == 1:
sign=1
else:
sign=-1
#段落位判断
if ins[i][1] == 0:
if ins[i][2]==0:
if ins[i][3]==0:
step,home=1,0
else:
step,home=1,16
elif ins[i][3]==0:
step,home=2,32
else:
step,home=4,64
elif ins[i][2]==0:
if ins[i][ 3] == 0:
step, home = 8, 128
else:
step, home = 16,256
else:
if ins[i][3] == 0:
step, home = 32, 512
else:
step, home = 64,1024
#段内码
newcode=(ins[i][4]*8+ins[i][5]*4+ins[i][6]*2+ins[i][7])*step+home
print(newcode)
out[i]=newcode
return out
注意格式,没什么难的。
以下是结果:
code=PCM_encode(["1522",25])
<class 'list'> <class 'numpy.ndarray'>
[[1. 1. 1. 1. 0. 1. 1. 1.]
[1. 0. 0. 1. 1. 0. 0. 1.]]
myDecode=PCM_decode([[1,1,1,1,0,1,1,1],[0,0,0,1,1,1,1,1]])
1472
31