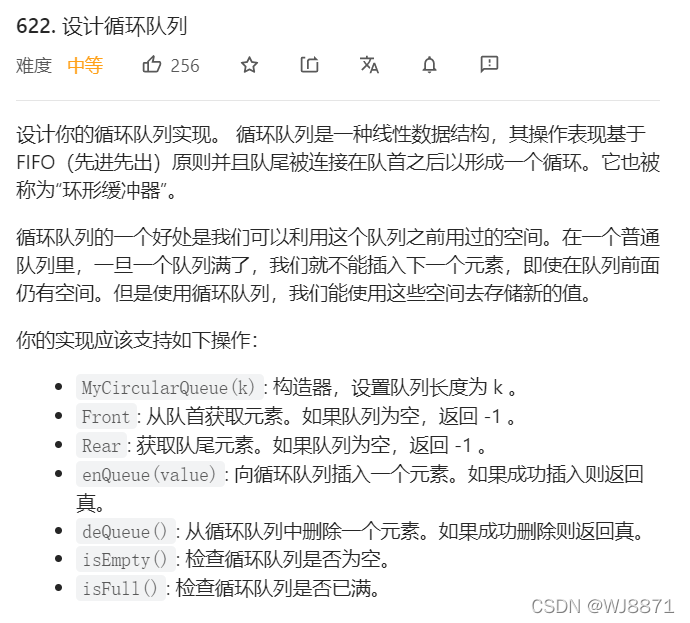
typedef struct {
int* array;
int front;
int rear;
int count; //用count++的方式判断循环队列是否满
int N; //总个数
} MyCircularQueue;
//初始化
MyCircularQueue* myCircularQueueCreate(int k) {
MyCircularQueue* myQueue=(MyCircularQueue*)malloc(sizeof(MyCircularQueue));
myQueue->array=(int *)malloc(sizeof(int)*k);
myQueue->front=0;
myQueue->rear=0;
myQueue->count=0;
myQueue->N=k;
return myQueue;
}
//判满
bool myCircularQueueIsFull(MyCircularQueue* obj) {
assert(obj);
return obj->count==obj->N;
}
//入队
bool myCircularQueueEnQueue(MyCircularQueue* obj, int value) {
assert(obj);
if(myCircularQueueIsFull(obj))
{
return false;
}
obj->array[obj->rear]=value;
obj->rear+=1;
// obj->rear=(obj->rear+1)%obj->N;
obj->count++;
if(obj->rear==obj->N)
{
obj->rear=0;
}
return true;
}
//判空
bool myCircularQueueIsEmpty(MyCircularQueue* obj) {
assert(obj);
return obj->count==0;
}
//出队
bool myCircularQueueDeQueue(MyCircularQueue* obj) {
assert(obj);
if(myCircularQueueIsEmpty(obj))
{
return false;
}
obj->front=(obj->front+1)%obj->N;
obj->count--;
return true;
}
//获取队头元素
int myCircularQueueFront(MyCircularQueue* obj) {
if(myCircularQueueIsEmpty(obj))
{
return -1;
}
assert(obj);
return obj->array[obj->front];
}
//获取队尾元素
int myCircularQueueRear(MyCircularQueue* obj) {
if(myCircularQueueIsEmpty(obj))
{
return -1;
}
assert(obj);
return obj->array[(obj->rear-1+obj->N)%obj->N];
}
//销毁
void myCircularQueueFree(MyCircularQueue* obj) {
assert(obj);
if(obj)
{
free(obj->array);
free(obj);
}
}