java exception分类
1、Throwable 结构图
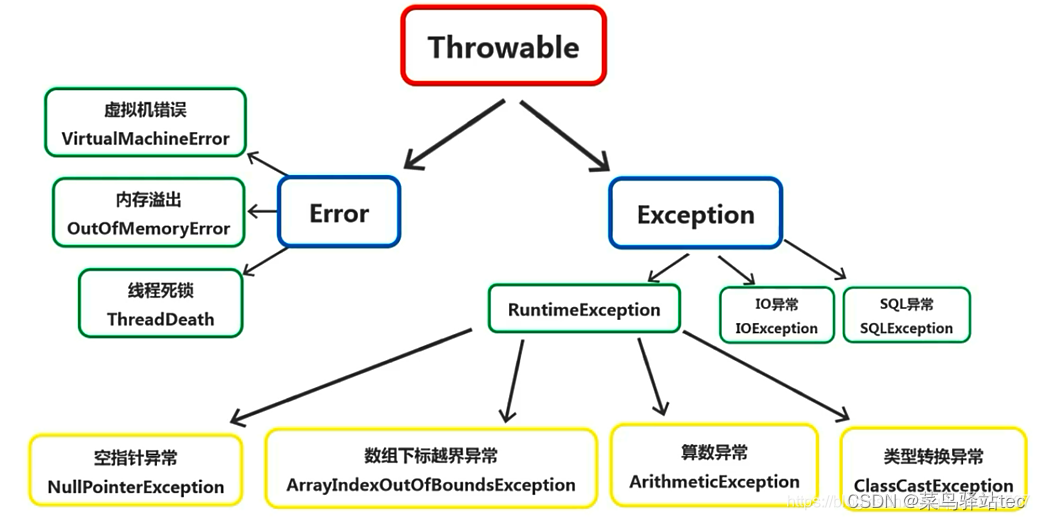
2、可检查与非检查异常:
1、可检查异常(checked exceptions)是程序运行过程中很容易出现的的异常情况,编译器要求必须捕获处理。这种错误在一定程度上是可以预见的,如IOException,FileNotFoundException等。
2、不可检查异常(unchecked exceptions)是编译器不强制要求处理的异常,主要包括运行时异常(RuntimeException与其子类)和错误(Error)。
3、运行与非运行异常:
1、运行时异常:都是RuntimeException类及其子类异常,如NullPointerException(空指针异常)、IndexOutOfBoundsException(下标越界异常)等,
2、非运行时异常(编译异常):是RuntimeException以外的异常,类型上都属于Exception类及其子类。
4、自定义异常
public class MyException extends RuntimeException{
private String msg;
public MyException(){
}
public MyException(String msg){
super();
this.msg = msg;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
public static void main(String[] args){
try {
testException();
}catch (MyException e){
System.out.println(e.getMsg());
}
}
public static void testException(){
try {
int a = 10/0;
}catch (Exception e){
throw new MyException("exception test");
}
}