目录
Express中间件
中间件的概念
next函数
全局中间与局部中间件
多个中间件
中间的5个注意事项
中间的分类
应用级中间件
路由级中间件
错误级中间件
Express内置中间件
express.json
const express = require('express')
const app = express()
// 内置中间件 express.json()
// 注意:除了错误级别的中间件express.error(),其他的中间件,必须在路由之前进行配置
// 通过express.json()这个中间件,解析json格式的数据
app.use(express.json())
app.post('/user',(req,res) => {
// 在服务器可以使用 req.body属性,接收客户端发送的请求体数据
//默认情况下,不配置解析表单数据的中间件,则req.body默认等于undefined
console.log('post ok,req.body = ',req.body)
res.send('post ok')
})
app.listen(80,() => {
console.log('http://127.0.0.1')
})
express.urlencoded
const express = require('express')
const app = express()
// 解析表单数据的中间件 express.urlencoded()
// 注意:当需要使用express.urlencoded()解析表单数据的时候,不要使用express.json()
app.use(express.urlencoded({extended:false}))
app.post('/book',(req,res) => {
// 在服务器可以使用 req.body属性,接收客户端发送的JSON格式的请求体数据
console.log('book:post ok,req.body = ',req.body)
res.send('book:post ok')
})
app.listen(80,() => {
console.log('http://127.0.0.1')
})
第三方中间件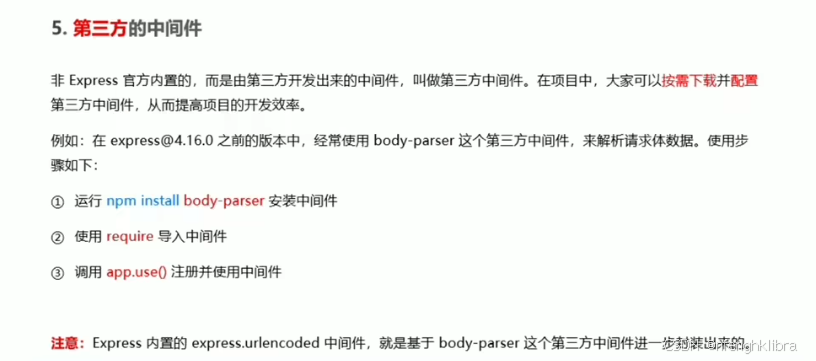
// body-parser中间件使用方法
// 导入expresss模块
const express = require('express')
// 穿件express的服务器实例
const app = express()
// 导入解析表单数据的body-parser中间件
const bodyParser = require('body-parser')
//使用app.use()注册中间件
app.use(bodyParser.urlencoded({extended:false}))
app.post('/user',(req,res) => {
console.log('user:post ok,req.body = ',req.body)
res.send('user:post ok')
})
// 调用 app.listen()方法,指定端口号并启动web服务器
app.listen(80,() => {
console.log('http://127.0.0.1')
})
自定义中间件
//自定义中间件
// 导入expresss模块
const express = require('express')
// 创建express的服务器实例
const app = express()
//导入node.js 内置的querystring模块
const qs= require('querystring')
//1.定义中间件,解析表单数据的中间件
app.use((req,res,next) => {
// 定义中间件具体业务逻辑
// 1.1 定义1个str字符串,专门用来存储客户端发送过来的请求体数据
let str = ''
// 1.2 监听req的data事件
req.on('data',(chunk) => {
str += chunk
})
// 1.3 监听req的end事件
req.on('end',() => {
// 在str中存放的是完整的请求体数据
// TODO:把字符串格式的请求体数据,解析为对象格式
const body = qs.parse(str)
// 1.4 把解析出来的对象格式的请求体数据,挂载到req.body属性上
req.body = body
// 1.5 调用next()函数,执行后续的业务逻辑
next()
})
})
app.post('/user',(req,res) => {
console.log('user:post ok,req.body = ',req.body)
res.send(req.body)
})
//调用app.listen()方法,指定端口号并启动web服务器
app.listen(80,() => {
console.log('http://127.0.0.1')
})