public function dumpData()
{
error_reporting(0);
ini_set('display_errors', 0);
$limit = $this->request->post('limit', 20, 'intval');
$offset = $this->request->post('offset', 0, 'intval');
$page = floor($offset / $limit) +1 ;
$where = [];
$name = trim($this->request->param('product_type_name'));
$product_type_id = $this->request->param('product_type_id');
$brand_ids = $this->request->param('brand_ids');
if ($name != ''){
$where[] = ['product_type.l_name_zh',"LIKE","%{$name}%"];
}
$brand_ids && $where[] = ['category.brand_ids',"in",$brand_ids];
if (!empty($product_type_id)){
$product_type_ids = $this->model->getChildIds($product_type_id);
if (empty($product_type_ids)) $product_type_ids = [-9999];
$where[] = ['product_type.product_type_id',"in",$product_type_ids];
}
$field = [
'category.category_carousel_id',
'category.product_type_id',
'category.description',
'category.create_time',
'category.update_time',
'category.create_user_id',
'category.update_user_id',
'category.category_carousel_id',
];
$orderby = 'category_carousel_id desc';
$res = CategoryCarouselService::indexList(formatWhere($where),$field,$orderby,$limit,$page);
$list = $res['rows'];
$img_count = array_column($list, 'img_list');
$img_count_list = [];
foreach ($img_count as $v){
$img_count_list[] = count($v);
}
rsort($img_count_list);
$max_img_count = $img_count_list[0] ?? 100;
$spreadsheet = \PhpOffice\PhpSpreadsheet\IOFactory::load(root_path() . 'public/tpl/分类优势导出模板.xls');
$worksheet = $spreadsheet->getActiveSheet();
$worksheet->setTitle('Product catalog');
$count=count($list);
$worksheet->insertNewRowBefore(3,$count-1);
$worksheet->getColumnDimension('E')->setWidth($max_img_count * 20);
$startline=2;
$path = root_path() . 'public/tmpxls/category/';
@mkdir($path);
$arr=['序号','产品总类','产品大类','产品小类','图片列表','产品小类优势','创建时间','更新时间','创建人','更新人'];
foreach($list as $k=>$v){
$offsetX = 10;
$offsetY = 10;
foreach ($v['img_list'] as $index => $img_list){
$img_url = $img_list['file_path_text'] ?? '';
if ($img_url == '') continue;
$line=$k+$startline;
$fileInfo = pathinfo($img_url);
$file = $fileInfo['filename'] .'.'.$fileInfo['extension'];
if (file_exists( $path.$file) === false) {
copy($img_url, $path.$file);
}
if (!file_exists($path.$file)) {
continue;
}
if(file_exists($path.$file)===true){
$drawing =new \PhpOffice\PhpSpreadsheet\Worksheet\Drawing();
$drawing->setName('Image ' . ($index + 1));
$drawing->setDescription('Image ' . ($index + 1));
$drawing->setPath($path.$file);
$drawing->setWidth(80);
$drawing->setHeight(80);
$drawing->setCoordinates('E'.$line);
$drawing->setOffsetX($offsetX);
$drawing->setOffsetY($offsetY);
$drawing->setWorksheet($spreadsheet->getActiveSheet());
$offsetX += 90;
}
}
$worksheet->getCell('A'.$line)->setValue(++$k);
$worksheet->getCell('B'.$line)->setValue($v['first_level_name']);
$worksheet->getCell('C'.$line)->setValue($v['second_level_name']);
$worksheet->getCell('D'.$line)->setValue($v['third_level_name']);
$worksheet->getCell('F'.$line)->setValue($v['description']);
$worksheet->getCell('G'.$line)->setValue($v['create_time']);
$worksheet->getCell('H'.$line)->setValue($v['update_time']);
$worksheet->getCell('I'.$line)->setValue($v['create_user_text']);
$worksheet->getCell('J'.$line)->setValue($v['update_user_text']);
}
$filename = date("YmdHis").'分类优势';
header('Content-Type: application/vnd.ms-excel');
header('Content-Disposition: attachment;filename='.$filename.'.Xlsx');
header('Cache-Control: max-age=0');
$writer = new \PhpOffice\PhpSpreadsheet\Writer\Xlsx($spreadsheet);
return $writer->save('php://output');
}
模板
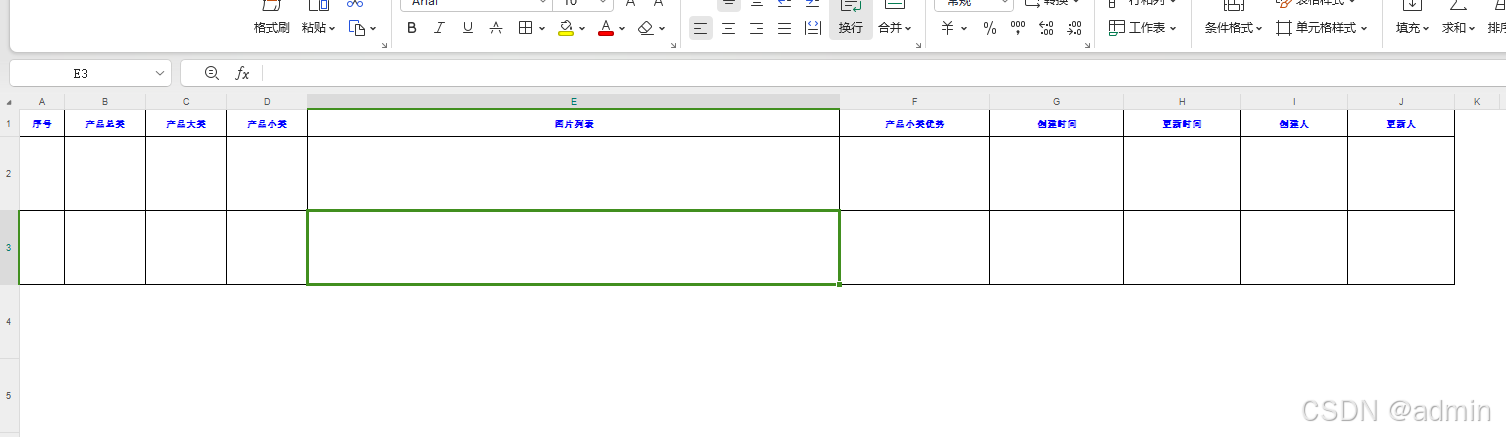