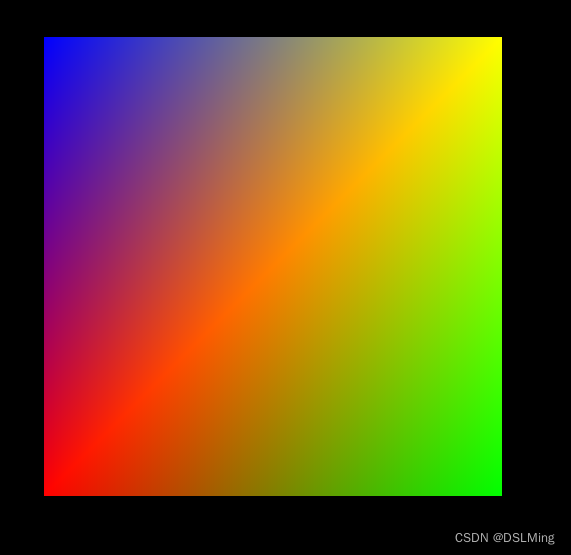
const planeGeometry = new Cesium.PlaneGeometry({
vertexFormat: Cesium.VertexFormat.POSITION_AND_ST,
});
const geometry = Cesium.PlaneGeometry.createGeometry(planeGeometry);
geometry.attributes.colors = new Cesium.GeometryAttribute({
componentDatatype: Cesium.ComponentDatatype.FLOAT,
componentsPerAttribute: 3,
values: [
1,0,0,
0,1,0,
1,1,0,
0,0,1,
],
});
const material = new Cesium.Material({
fabric: {
uniforms: {
},
source: `
czm_material czm_getMaterial(czm_materialInput materialInput){
czm_material material = czm_getDefaultMaterial(materialInput);
return material;
}`
},
});
const center = Cesium.Cartesian3.fromDegrees(106, 26, 10)
const translateMatrix = Cesium.Transforms.eastNorthUpToFixedFrame(center);
const dimensions = new Cesium.Cartesian3(400.0, 400.0, 1.0);
const scaleMatrix = Cesium.Matrix4.fromScale(dimensions);
const modelMatrix = new Cesium.Matrix4();
Cesium.Matrix4.multiply(translateMatrix, scaleMatrix, modelMatrix);
const plane = new Cesium.Primitive({
asynchronous: false,
geometryInstances: new Cesium.GeometryInstance({
geometry: geometry,
attributes: {
color: Cesium.ColorGeometryInstanceAttribute.fromColor(Cesium.Color.BLUE)
},
modelMatrix
}),
appearance: new Cesium.MaterialAppearance({
material: material,
vertexShaderSource: `
in vec3 position3DHigh;
in vec3 position3DLow;
in vec3 normal;
in vec2 st;
in float batchId;
in vec4 color;
in vec4 colors;
out vec3 v_positionEC;
out vec3 v_normalEC;
out vec2 v_st;
out vec4 v_color;
void main() {
vec4 p = czm_computePosition();
v_positionEC = (czm_modelViewRelativeToEye * p).xyz;
v_normalEC = czm_normal * normal;
v_st = st;
v_color = colors;
gl_Position = czm_modelViewProjectionRelativeToEye * p;
}
`,
fragmentShaderSource: `
in vec2 v_st;
in vec4 v_color;
void main() {
out_FragColor = vec4(v_color.rgb, 1.);
}
`
}),
show: true,
})
viewer.scene.primitives.add(plane);