在 C++ 中,constexpr
和 const
都用于修饰变量,使其成为只读变量,但两者的作用和适用场景不同,尤其在编译时计算方面存在本质区别。
1. const
关键字
✅ 作用:表示变量是常量,不能被修改。
✅ 特点:
- 变量的值不能修改(编译期检查)。
- 可以用于编译期或运行期的常量(但不一定是编译时常量)。
- 可以用于指针、函数参数、返回值等。
const
可能是运行期变量
int getValue() { return 42; }
void test() {
int x = getValue(); // x 只有运行时才能确定
const int y = x; // y 是 const,但它的值只有运行时才能确定
}
✅ const
变量 不一定 在编译时确定,它可能依赖运行时计算。
2. constexpr
关键字
✅ 作用:表示变量、函数、对象的值必须在编译期可计算。
✅ 特点:
- 强制在编译期求值,提高运行时效率。
- 适用于常量表达式(编译时能确定的值)。
- 可用于修饰函数,确保返回值在编译期计算(
constexpr
函数)。
(1)constexpr
变量(必须是编译时常量)
constexpr int a = 10; // OK,a 是编译时常量
constexpr int b = a + 5; // OK,b 也是编译时常量
int getValue() { return 42; }
constexpr int c = getValue(); // ❌ 编译错误:getValue() 不是编译期常量
✅ constexpr
变量必须在编译期确定,不能依赖运行时计算。
(2)constexpr
函数
constexpr int square(int x) {
return x * x;
}
constexpr int result = square(5); // OK,在编译时计算
int runtime_result = square(getValue()); // 运行时计算
✅ constexpr
函数如果传入编译时常量,则编译期计算;如果传入运行时变量,则在运行时计算。
3. constexpr
vs const
详细对比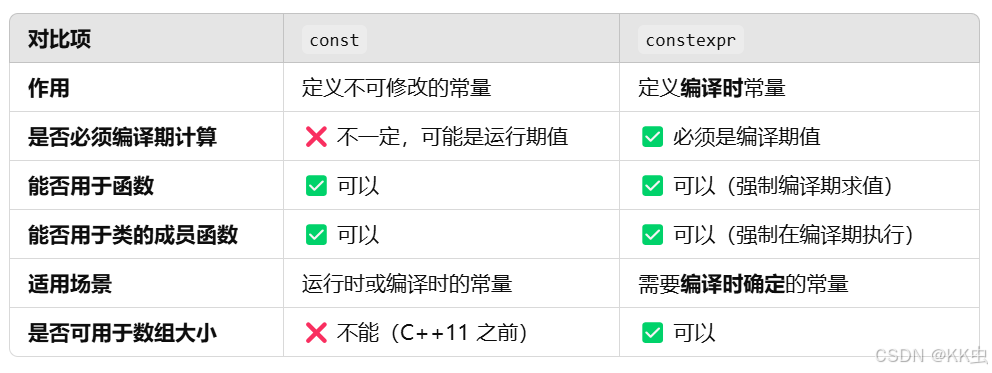
4. constexpr
vs const
使用场景
✅ 使用 const
的场景
- 变量的值可能是运行时确定的。
- 只想让变量只读,但不强制它是编译期常量。
✅ 使用 constexpr
的场景
- 需要在编译期计算值,提高效率。
- 作为数组大小,
switch
语句的 case 标签。 - 定义
constexpr
函数,以便在编译期计算。
5. 面试常见问题
🔥 1. constexpr
是 const
吗? ✅ 是的,constexpr
隐式地是 const
,但它要求必须是编译期常量。
🔥 2. const
一定是编译时常量吗? ❌ 不一定,const
变量可能在运行时确定。
🔥 3. constexpr
变量能存储运行时值吗? ❌ 不能,constexpr
变量必须在编译期确定。
🔥 4. constexpr
和 const
什么时候用? ✅ 如果变量值运行时才能确定,用 const
。
✅ 如果变量值必须在编译期确定,用 constexpr
。
6. 结论
💡 口诀记忆
const
只是“只读”,但值可以在运行时确定。constexpr
是“编译时常量”,必须在编译期求值。constexpr
默认是const
,但更严格。- C++ 中应优先使用
constexpr
进行常量计算,提高性能!