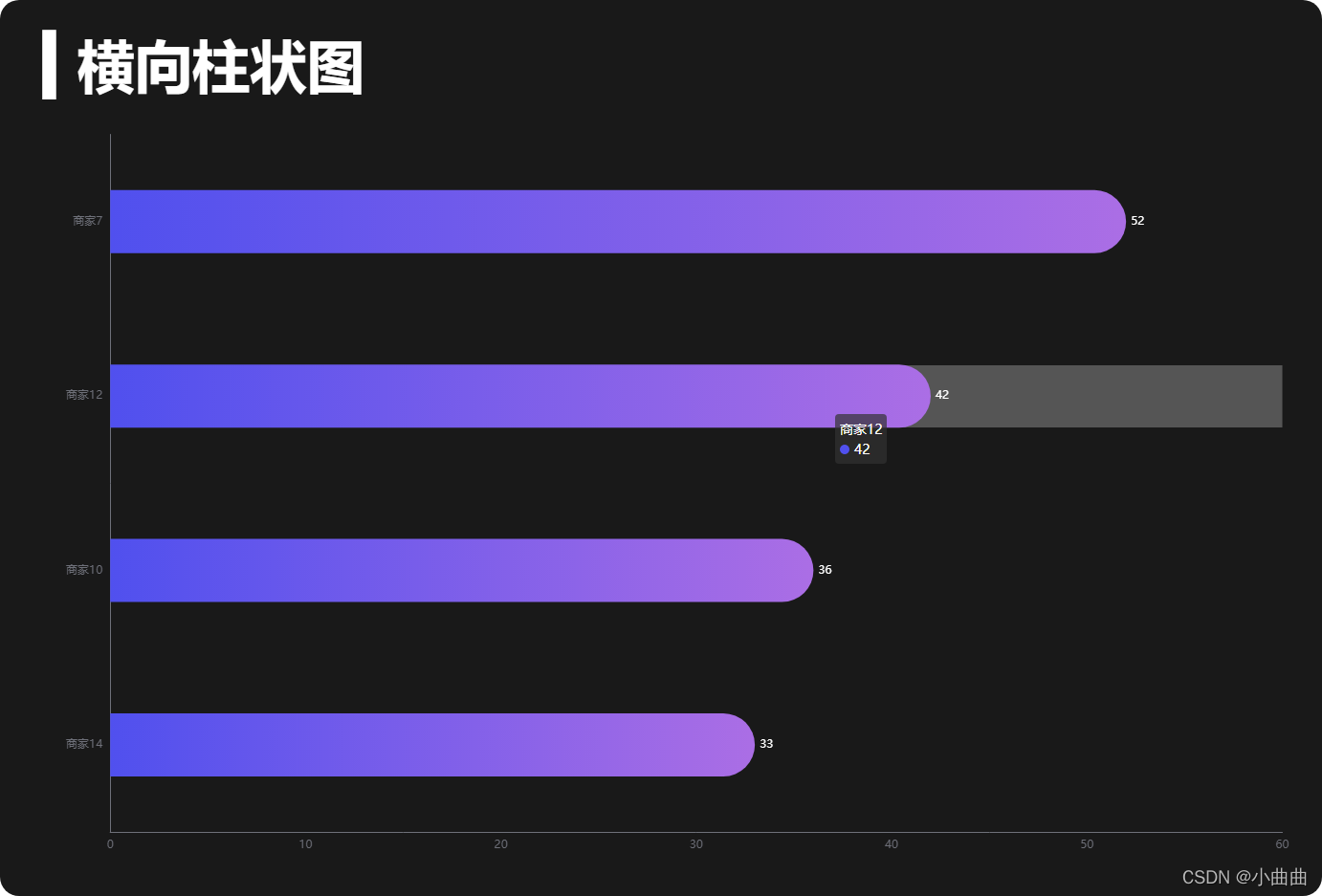
功能描述
- 全屏横向柱状图
- 从小到大排序,每次显示5条,每3秒切换循环显示
- 鼠标移入停止切换,鼠标移出继续切换
柱状图组件源码
<template>
<div class="w100 h100">
<div ref="bar" class="w100 h100"></div>
</div>
</template>
<script>
import { ququ } from "../../public/static/theme/ququ";
export default {
data() {
return {
barChart: null,
barData: [
{
name: "商家1",
value: 12,
},
{
name: "商家2",
value: 22,
},
{
name: "商家3",
value: 10,
},
{
name: "商家4",
value: 32,
},
{
name: "商家5",
value: 25,
},
{
name: "商家6",
value: 16,
},
{
name: "商家7",
value: 52,
},
{
name: "商家8",
value: 33,
},
{
name: "商家9",
value: 19,
},
{
name: "商家10",
value: 36,
},
{
name: "商家11",
value: 24,
},
{
name: "商家12",
value: 42,
},
{
name: "商家13",
value: 29,
},
{
name: "商家14",
value: 33,
},
],
current: 1,
total: null,
timerId: null,
};
},
mounted() {
this.init();
this.update();
this.openTimer();
},
beforeDestroy() {
clearInterval(this.timerId);
},
methods: {
init() {
this.$echarts.registerTheme("ququ", ququ);
this.barChart = this.$echarts.init(this.$refs.bar, "ququ");
this.barChart.on("mouseover", (params) => {
console.log(params);
clearInterval(this.timerId);
});
this.barChart.on("mouseout", () => {
this.openTimer();
});
},
update() {
this.barData = this.barData.sort((a, b) => a.value - b.value);
this.total =
this.barData.length % 5 == 0
? this.barData.length / 5
: parseInt(this.barData.length / 5) + 1;
let list = this.barData.slice((this.current - 1) * 5, this.current * 5);
let barDataY = list.map((item) => item.name);
let barDataX = list.map((item) => item.value);
let option = {
title: {
text: "▍横向柱状图",
left: 40,
top: 40,
textStyle: {
fontSize: 60,
color: "#fff",
},
},
grid: {
top: "15%",
left: "5%",
right: "3%",
bottom: "5%",
containLabel: true,
},
xAxis: {
type: "value",
},
yAxis: {
type: "category",
data: barDataY,
},
series: [
{
type: "bar",
data: barDataX,
barWidth: 66,
label: {
show: true,
position: "right",
color: "white",
},
itemStyle: {
barBorderRadius: [0, 34, 34, 0],
color: new this.$echarts.graphic.LinearGradient(0, 0, 1, 0, [
{ offset: 0, color: "#5050ee" },
{ offset: 1, color: "#ab6ee5" },
]),
},
},
],
tooltip: {
trigger: "axis",
axisPointer: {
type: "line",
z: 0,
lineStyle: {
color: "rgba(225,225,225,.3)",
width: 65,
},
},
},
};
this.barChart.setOption(option);
},
openTimer() {
if (this.timerId) {
clearInterval(this.timerId);
}
this.timerId = setInterval(() => {
if (this.current < this.total) {
this.current++;
} else {
this.current = 1;
}
this.update();
}, 6000);
},
},
};
</script>
option配置项的拆分
- initOption:初始化的静态配置
- dataOption:获取到动态数据后的数据配置
- screenOption:适配屏幕分辨率大小的配置
this.barChart.setOption(option);
加上适配屏幕和option拆分的代码
<template>
<div class="w100 h100">
<div ref="bar" class="w100 h100"></div>
</div>
</template>
<script>
import { ququ } from "../../public/static/theme/ququ";
export default {
props: {
msg: String,
},
data() {
return {
barChart: null,
barData: [
{
name: "商家1",
value: 12,
},
{
name: "商家2",
value: 22,
},
{
name: "商家3",
value: 10,
},
{
name: "商家4",
value: 32,
},
{
name: "商家5",
value: 25,
},
{
name: "商家6",
value: 16,
},
{
name: "商家7",
value: 52,
},
{
name: "商家8",
value: 33,
},
{
name: "商家9",
value: 19,
},
{
name: "商家10",
value: 36,
},
{
name: "商家11",
value: 24,
},
{
name: "商家12",
value: 42,
},
{
name: "商家13",
value: 29,
},
{
name: "商家14",
value: 33,
},
],
current: 1,
total: null,
timerId: null,
};
},
mounted() {
this.init();
this.getData();
this.openTimer();
window.addEventListener("resize", this.screenResize);
this.screenResize();
},
beforeDestroy() {
clearInterval(this.timerId);
window.removeEventListener("resize", this.screenResize);
},
methods: {
init() {
this.$echarts.registerTheme("ququ", ququ);
this.barChart = this.$echarts.init(this.$refs.bar, "ququ");
let initOption = {
title: {
text: "▍横向柱状图",
left: 40,
top: 40,
textStyle: {
color: "#fff",
},
},
grid: {
top: "15%",
left: "5%",
right: "3%",
bottom: "5%",
containLabel: true,
},
xAxis: {
type: "value",
},
yAxis: {
type: "category",
},
series: [
{
type: "bar",
label: {
show: true,
position: "right",
color: "white",
},
itemStyle: {
color: new this.$echarts.graphic.LinearGradient(0, 0, 1, 0, [
{ offset: 0, color: "#5050ee" },
{ offset: 1, color: "#ab6ee5" },
]),
},
},
],
tooltip: {
trigger: "axis",
axisPointer: {
type: "line",
z: 0,
lineStyle: {
color: "rgba(225,225,225,.3)",
},
},
},
};
this.barChart.setOption(initOption);
this.barChart.on("mouseover", (params) => {
console.log(params);
clearInterval(this.timerId);
});
this.barChart.on("mouseout", () => {
this.openTimer();
});
},
getData() {
this.barData = this.barData.sort((a, b) => a.value - b.value);
this.total =
this.barData.length % 5 == 0
? this.barData.length / 5
: parseInt(this.barData.length / 5) + 1;
let list = this.barData.slice((this.current - 1) * 5, this.current * 5);
let barDataY = list.map((item) => item.name);
let barDataX = list.map((item) => item.value);
let dataOption = {
yAxis: {
data: barDataY,
},
series: [
{
data: barDataX,
},
],
};
this.barChart.setOption(dataOption);
},
openTimer() {
if (this.timerId) {
clearInterval(this.timerId);
}
this.timerId = setInterval(() => {
if (this.current < this.total) {
this.current++;
} else {
this.current = 1;
}
this.getData();
}, 6000);
},
screenResize() {
this.$nextTick(() => {
let width = this.$refs.bar.offsetWidth;
let size = (width / 100) * 3.6;
let screenOption = {
title: {
textStyle: {
fontSize: size,
},
},
series: [
{
barWidth: size,
itemStyle: {
barBorderRadius: [0, size / 2, size / 2, 0],
},
},
],
tooltip: {
axisPointer: {
lineStyle: {
width: size,
},
},
},
};
this.barChart.setOption(screenOption);
this.barChart.resize();
});
},
},
};
</script>